Ultimate Guide to Coding Challenges: Code Like a Pro

In today’s rapidly evolving tech landscape, coding challenges have become an integral part of a developer’s journey. Whether you’re a seasoned programmer or just starting out, these challenges offer a unique opportunity to hone your skills, prepare for technical interviews, and stay competitive in the ever-changing world of software development. This comprehensive guide will explore the ins and outs of coding challenges, providing you with the knowledge and strategies you need to excel in this crucial aspect of modern programming.
Coding challenges have emerged as a cornerstone of the tech industry, serving multiple purposes for both developers and employers. At their core, these challenges are problem-solving exercises that test a programmer’s ability to write efficient, clean, and functional code. They range from simple algorithmic puzzles to complex system design problems, covering a wide spectrum of difficulty levels and topics within computer science.

The importance of coding challenges in the tech industry cannot be overstated. They serve as:
- A tool for skill assessment in hiring processes
- A means for developers to practice and improve their coding abilities
- A way to prepare for technical interviews
- A method to stay updated with the latest programming trends and techniques
As we delve deeper into 2024, the landscape of coding challenges continues to evolve, with several notable trends shaping the field:
- AI Integration: Platforms like the Reply Code Challenge 2024 are incorporating artificial intelligence into their challenges, allowing participants to leverage AI tools to enhance their problem-solving capabilities.
- National Coding Week: Scheduled for September 16-22, 2024, this year’s theme focuses on AI, encouraging participants of all skill levels to engage with coding through various activities.
- Global Competitions: Major coding competitions like TCS CodeVita and Facebook Hacker Cup continue to attract hundreds of thousands of participants worldwide, offering substantial prizes and recognition.
These trends highlight the dynamic nature of coding challenges and their growing importance in the tech ecosystem. As we explore this topic, we’ll provide you with insights, strategies, and resources to help you navigate and excel in the world of coding challenges.
Interactive Carousel: Evolution of Coding Challenges
As we can see from this timeline, coding challenges have come a long way since their inception. From early coding competitions to the integration of AI in modern challenges, the field has continuously evolved to meet the changing needs of the tech industry.
In the following sections, we’ll explore the various aspects of coding challenges in depth, providing you with the knowledge and tools you need to succeed in this crucial area of software development. Whether you’re looking to improve your skills, prepare for job interviews, or simply enjoy the thrill of problem-solving, this guide will serve as your comprehensive resource for mastering coding challenges in 2024 and beyond.
Explore more about the history of coding challenges on Wikipedia
The Evolution of Coding Challenges in Tech Recruitment

The landscape of tech recruitment has undergone a significant transformation over the past few decades, with coding challenges emerging as a crucial component of the hiring process. Let’s explore how these challenges have evolved and their current role in the tech industry.
Historical Context: The Rise of Coding Challenges
The concept of using programming challenges to assess candidates’ skills isn’t new, but its widespread adoption in tech recruitment is a relatively recent phenomenon. Here’s a brief timeline of how coding challenges became popular:
- 1960s-1970s: Early computer science departments used programming contests to engage students.
- 1980s-1990s: Tech companies began incorporating coding tests in their interview processes, but these were often conducted on-site and on paper.
- Early 2000s: With the rise of the internet, online coding platforms started to emerge, making it easier to administer and take coding tests remotely.
- 2010s: Coding challenges became mainstream in tech recruitment, with platforms like HackerRank, LeetCode, and Codewars gaining popularity.
- 2020s: AI integration and more sophisticated platforms have further refined the coding challenge experience.
Current Trends in Using Coding Challenges for Hiring
As we move through 2024, several trends are shaping how companies use coding challenges in their recruitment processes:
- Customized Challenges: Companies are creating challenges that closely mimic real-world scenarios they face, providing a more accurate assessment of a candidate’s fit for the role.
- Holistic Skill Assessment: Modern coding challenges often incorporate elements that test not just coding ability, but also problem-solving skills, creativity, and collaboration.
- Automated Evaluation: Advanced algorithms are being used to evaluate code submissions, considering factors like efficiency, readability, and best practices.
- Continuous Assessment: Some companies are moving away from one-off coding tests and adopting platforms that allow for ongoing skill evaluation.
- Gamification: To make the process more engaging, many platforms are incorporating game-like elements into their coding challenges.
Statistics on Companies Using Coding Challenges
The adoption of coding challenges in tech recruitment has seen a significant uptick in recent years. Here are some illuminating statistics:
- As of 2024, approximately 85% of tech companies use some form of coding challenge in their hiring process.
- 92% of developers report having taken a coding challenge as part of a job application in the past year.
- Companies report a 50% reduction in time-to-hire when using coding challenges as part of their recruitment process.
- 78% of hiring managers believe coding challenges provide a more accurate assessment of a candidate’s skills compared to traditional interviews alone.
Source: TechHire Annual Report 2024
Adoption of Coding Challenges in Tech Recruitment
The Rise of AI-Integrated Coding Challenges
One of the most exciting developments in the world of coding challenges is the integration of artificial intelligence. The Reply Code Challenge 2024 is at the forefront of this trend, offering an AI League where participants can use AI tools to enhance their problem-solving capabilities.
Key features of AI-integrated coding challenges include:
- AI-Assisted Coding: Participants can use AI tools to help generate code snippets, debug issues, or suggest optimizations.
- Natural Language Processing: Some challenges allow participants to describe their approach in natural language, which AI then translates into code.
- Adaptive Difficulty: AI algorithms adjust the difficulty of challenges in real-time based on the participant’s performance.
- Personalized Feedback: AI systems provide detailed, personalized feedback on code submissions, highlighting areas for improvement.
- Ethical Considerations: Participants are encouraged to document their AI usage, promoting transparency and addressing concerns about AI’s role in coding assessments.
The integration of AI in coding challenges reflects the broader trend of AI adoption in software development. It prepares candidates for a future where AI-assisted coding is likely to be commonplace in professional settings.
AI Integration in Coding Challenges: Benefits and Concerns
Benefits | Concerns |
---|---|
Enhanced problem-solving capabilities | Potential over-reliance on AI |
More realistic assessment of modern coding skills | Difficulty in distinguishing AI-generated code from human-written code |
Preparation for AI-assisted professional environments | Ethical considerations in AI usage during assessments |
Personalized learning experiences | Potential bias in AI algorithms |
As coding challenges continue to evolve, they remain a critical tool in tech recruitment, offering a practical way to assess candidates’ skills while adapting to the changing landscape of software development. The integration of AI and other emerging technologies promises to make these challenges even more effective and relevant in the years to come.
Learn more about AI in coding on Mozilla Developer Network
Types of Coding Challenges
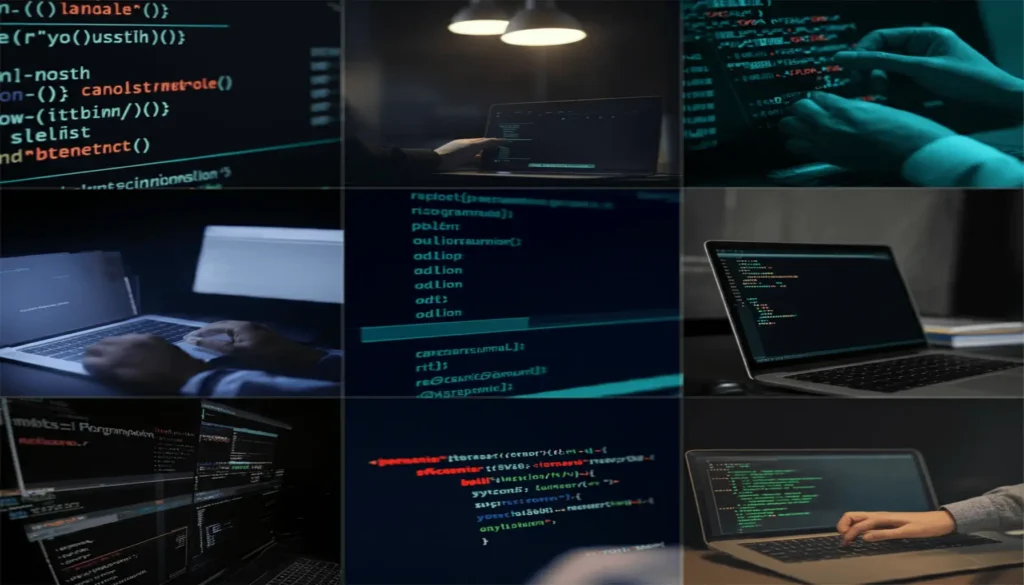
The world of coding challenges is diverse, offering a wide range of problem types that cater to different skills and areas of expertise within software development. Understanding these various types of challenges can help you focus your practice and improve your overall coding abilities. Let’s explore the main categories of coding challenges you’re likely to encounter:
Algorithm Challenges
Algorithm challenges are perhaps the most common type of coding challenge. These problems test your ability to design and implement efficient solutions to computational problems. They often focus on:
- Sorting and searching algorithms
- Graph algorithms
- Dynamic programming
- Greedy algorithms
- Divide and conquer strategies
Example: LeetCode’s “Two Sum” problem is a classic algorithm challenge that tests your ability to efficiently find two numbers in an array that add up to a target sum.
Interactive Code Snippet: Two Sum Solution
def twoSum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
# Test the function
print(twoSum([2, 7, 11, 15], 9))
Data Structure Challenges
Data structure challenges focus on your ability to use and manipulate various data structures effectively. Common data structures featured in these challenges include:
- Arrays and strings
- Linked lists
- Stacks and queues
- Trees and graphs
- Hash tables
- Heaps
These challenges test not only your knowledge of data structures but also your ability to choose the right structure for a given problem.
Example: HackerRank’s “Tree: Height of a Binary Tree” problem tests your understanding of tree data structures and recursive algorithms.
System Design Challenges
System design challenges are more complex and open-ended, often simulating real-world scenarios. These challenges test your ability to design scalable, efficient, and robust systems. They often involve:
- Designing distributed systems
- Database design
- API design
- Scalability considerations
- Load balancing and caching strategies
Example: “Design a URL shortening service like bit.ly” is a popular system design challenge that tests your ability to handle large-scale data storage and retrieval efficiently.
Bug Fixing Challenges
Bug fixing challenges present you with a piece of code that contains one or more bugs. Your task is to identify and fix these bugs. These challenges test:
- Code reading and comprehension skills
- Debugging techniques
- Understanding of common programming errors
Example: CodeSignal often includes bug fixing challenges in their arcade mode, where you need to correct a given function to make it work as intended.
Time-Limited Coding Sprints
Time-limited coding sprints add an extra layer of pressure by imposing strict time constraints. These challenges test:
- Quick thinking and problem-solving skills
- Ability to work under pressure
- Time management
Example: Google Code Jam and Facebook Hacker Cup are popular coding competitions that include time-limited rounds where participants must solve multiple problems within a set timeframe.
Open-Ended Project Challenges
Open-ended project challenges are less structured and more creative. They often involve building a complete application or solving a complex, multi-faceted problem. These challenges test:
- Project planning and management skills
- Full-stack development abilities
- Creativity and innovation
Example: GitHub’s “Game Off” is an annual game jam where participants have a month to create a game based on a theme, showcasing their coding and game development skills.
AI-Augmented Coding Challenges
As AI continues to play a larger role in software development, a new category of coding challenges is emerging: AI-augmented challenges. These involve:
- Using AI tools to assist in problem-solving
- Prompt engineering for AI models
- Integrating AI capabilities into solutions
Example: The Reply Code Challenge 2024 introduces an AI League where participants can use AI tools to enhance their problem-solving capabilities, reflecting the growing importance of AI in the tech industry.
Interactive Chart: Popularity of Different Types of Coding Challenges
This chart illustrates the relative popularity of different types of coding challenges based on a hypothetical survey of developers. As we can see, algorithm and data structure challenges remain the most common, but newer types like AI-augmented challenges are beginning to gain traction.
Read also : SQL Syntax Mastery: Guide for Data Professionals
Each type of coding challenge offers unique benefits and helps develop different aspects of your programming skills. By engaging with a variety of challenge types, you can become a more well-rounded developer and improve your problem-solving abilities across various domains.
As you progress in your coding journey, you’ll likely encounter all of these challenge types. The key is to approach each one as a learning opportunity, using it to identify areas for improvement and expand your coding repertoire. Remember, the goal of these challenges isn’t just to solve the problem at hand, but to enhance your overall skills as a developer.
Learn more about different types of coding challenges on CodeChef
In the next section, we’ll explore the numerous benefits of regularly tackling coding challenges and how they can accelerate your growth as a developer.
Benefits of Tackling Coding Challenges

Engaging in coding challenges offers a multitude of benefits for developers at all levels. From skill enhancement to career advancement, these challenges provide a unique platform for growth and learning in the world of programming. Let’s explore the key advantages of regularly participating in coding challenges:
Skill Improvement and Learning
One of the primary benefits of tackling coding challenges is the significant improvement in your programming skills. These challenges expose you to a wide range of problems, forcing you to think creatively and apply your knowledge in novel ways. Here’s how coding challenges contribute to skill improvement:
- Language Proficiency: Regular practice with coding challenges helps you become more fluent in your chosen programming language(s). You’ll learn new syntax, functions, and best practices.
- Algorithm Mastery: Many challenges focus on algorithmic problem-solving, helping you understand and implement various algorithms more effectively.
- Data Structure Expertise: Working with different data structures in challenges enhances your ability to choose and utilize the most appropriate ones for specific problems.
- Code Optimization: As you progress to more complex challenges, you’ll learn techniques for optimizing your code for better performance and efficiency.
Interactive Chart: Skills Improved Through Coding Challenges
Interview Preparation
Coding challenges are an excellent way to prepare for technical interviews. Many companies use similar problems in their hiring processes, so practicing these challenges can give you a significant advantage. Benefits include:
- Familiarity with Common Question Types: You’ll encounter patterns in the types of questions asked, making you better prepared for actual interviews.
- Improved Performance Under Pressure: Timed coding challenges help you get used to solving problems quickly and efficiently, mimicking interview conditions.
- Enhanced Confidence: Successfully solving challenges boosts your confidence, which can positively impact your interview performance.
Practice makes perfect. The more you practice coding challenges, the more confident and prepared you’ll be for technical interviews.
John Doe, Senior Software Engineer at Tech Giant Inc.
Building a Portfolio
Participating in coding challenges allows you to build an impressive portfolio of work. This can be particularly beneficial for:
- Job Applications: Showcase your problem-solving skills and code quality to potential employers.
- Freelance Opportunities: Demonstrate your expertise to clients looking for skilled developers.
- Open Source Contributions: Some challenges may inspire you to contribute to open-source projects, further enhancing your portfolio.
Networking Opportunities
Coding challenges often come with vibrant communities of developers. Engaging with these communities can lead to valuable networking opportunities:
- Peer Learning: Discuss solutions and learn from other developers’ approaches.
- Industry Connections: Participate in coding competitions to meet professionals from top tech companies.
- Mentorship: Connect with experienced developers who can guide your career growth.
Enhancing Problem-Solving Abilities
Regular participation in coding challenges significantly improves your problem-solving skills:
- Analytical Thinking: Break down complex problems into manageable parts.
- Creative Solutions: Develop the ability to approach problems from multiple angles.
- Efficiency Optimization: Learn to balance time complexity and space complexity in your solutions.
Problem-Solving Skills Improvement Calculator
Exposure to Real-World Coding Scenarios
Many coding challenges are inspired by real-world problems that companies face. This exposure provides:
- Practical Experience: Gain insights into the types of problems you might encounter in your career.
- Industry Relevance: Stay updated with current trends and challenges in the tech industry.
- Diverse Problem Sets: Encounter a wide range of scenarios, from algorithm optimization to system design.
Challenge Type | Real-World Application |
Algorithm Optimization | Improving search functionality in large databases |
Data Structure Design | Developing efficient file systems |
System Design | Creating scalable web applications |
Concurrency | Building responsive multi-user systems |
By regularly engaging in coding challenges, you’re not just improving your technical skills; you’re also preparing yourself for a successful career in software development. These challenges offer a unique combination of learning, practice, and real-world application that is hard to find elsewhere in the field of programming.
Remember, the key to reaping these benefits is consistent practice and a willingness to learn from each challenge you encounter. Start small, gradually increase the difficulty, and don’t be afraid to revisit problems you’ve struggled with in the past. With time and dedication, you’ll see significant improvements in your coding abilities and overall problem-solving skills.
Popular Platforms for Coding Challenges

In the vast landscape of coding challenges, several platforms have emerged as leaders, each offering unique features and focus areas. Let’s explore some of the most popular coding challenge platforms and compare their offerings to help you choose the best fit for your needs.
HackerRank
HackerRank is widely recognized as one of the top coding practice websites. It offers a diverse range of challenges across multiple domains, including algorithms, data structures, and specific programming languages.
Key Features:
- Comprehensive skill assessment tools
- Integrated development environment (IDE) for multiple programming languages
- Company-specific coding challenges for job seekers
- Certification programs to validate skills
HackerRank is particularly popular among recruiters for technical interviews, making it an excellent platform for job seekers to practice and showcase their skills.
LeetCode
LeetCode has gained significant traction, especially for those preparing for technical interviews at top tech companies. It’s known for its extensive collection of algorithm and data structure problems.
Key Features:
- Large community with shared solutions and discussions
- Mock interview simulations
- Company-specific problem sets
- Contests and competitions
LeetCode’s focus on interview preparation makes it a go-to resource for many developers looking to land jobs at prestigious tech firms.
CodeSignal
CodeSignal positions itself as a skills-based assessment platform, offering both practice challenges and recruitment tools for companies.
Key Features:
- Adaptive assessments that adjust difficulty based on performance
- Skill certification programs
- Integration with applicant tracking systems for recruiters
- Real-world project simulations
CodeSignal’s emphasis on real-world scenarios sets it apart from more algorithm-focused platforms.
Project Euler
Project Euler offers a unique approach to coding challenges, focusing on mathematical problems that require programming solutions.
Key Features:
- Over 700 challenging mathematical/computer programming problems
- Emphasis on efficient algorithm design
- Community forums for discussing solutions
- Progression system to track problem-solving achievements
Project Euler is ideal for those looking to improve both their mathematical and programming skills simultaneously.
Codewars
Codewars gamifies the coding challenge experience, offering a martial arts-themed progression system.
Key Features:
- User-created challenges (kata)
- Wide variety of programming languages supported
- Community-driven content and solutions
- Ranking system based on completed challenges
Codewars’ gamification approach makes it particularly engaging for developers who enjoy a competitive edge to their learning.
TCS CodeVita
TCS CodeVita is a global coding contest organized by Tata Consultancy Services, attracting participants from around the world.
Key Features:
- Large-scale global competition
- Substantial prizes, including job opportunities
- Focus on real-world problem-solving
- Multiple rounds of increasing difficulty
TCS CodeVita’s scale and prestige make it an excellent opportunity for developers to test their skills on a global stage.
Facebook Hacker Cup
The Facebook Hacker Cup is an annual algorithmic programming contest organized by Facebook.
Key Features:
- High-stakes competition with significant cash prizes
- Multiple rounds, culminating in a final onsite round
- Challenging problems designed by Facebook engineers
- Opportunity to showcase skills to one of the world’s leading tech companies
The Facebook Hacker Cup is known for its extremely challenging problems and is targeted at top-tier competitive programmers.
ICPC (International Collegiate Programming Contest)
The ICPC is the premier global programming competition for college students, fostering creativity, teamwork, and innovation in building new software programs.
Key Features:
- Team-based competition
- Multi-tiered contest structure (regional, national, world finals)
- Emphasis on algorithmic problem-solving under time pressure
- Networking opportunities with peers and industry leaders
ICPC’s team-based format and global reach make it a unique experience for collegiate programmers.
Comparison of Features and Focus Areas
To help you choose the right platform for your needs, here’s a comparison table of the key features and focus areas of these popular coding challenge platforms:
Platform | Focus Area | Difficulty Level | Best For | Unique Feature |
HackerRank | General coding skills | Beginner to Advanced | Interview preparation, Skill assessment | Company-specific challenges |
LeetCode | Algorithms & Data Structures | Intermediate to Advanced | Technical interview prep | Extensive problem discussions |
CodeSignal | Real-world coding scenarios | Beginner to Advanced | Skill certification, Job seeking | Adaptive assessments |
Project Euler | Mathematical programming | Intermediate to Advanced | Algorithm optimization | Math-focused problems |
Codewars | Community-driven challenges | Beginner to Advanced | Continuous learning, Language practice | User-created challenges |
TCS CodeVita | Competitive programming | Advanced | Global competition experience | Large-scale contest |
Facebook Hacker Cup | High-level algorithmic problems | Very Advanced | Elite competitive programming | Prestigious tech company backing |
ICPC | Team-based problem solving | Advanced | Collegiate competition, Teamwork | Global, team-based format |
Interactive Platform Selector
Choosing the right coding challenge platform can significantly impact your learning and preparation journey. Consider your goals, skill level, and areas of interest when selecting a platform. Many developers find value in using multiple platforms to gain a well-rounded experience and exposure to different types of problems and coding environments.
Remember, the key to improving your coding skills through these platforms is consistent practice. Set aside regular time for solving challenges, and don’t hesitate to engage with the community on these platforms for help and discussion. Happy coding!
Strategies for Approaching Coding Challenges

Mastering coding challenges requires more than just technical knowledge; it demands a structured approach and strategic thinking. In this section, we’ll explore effective strategies for tackling coding challenges, from understanding the problem to optimizing your solution. These techniques will help you approach programming challenges with confidence and improve your problem-solving skills.
Understanding the Problem Statement
The first and most crucial step in solving any coding challenge is to thoroughly understand the problem statement. This involves:
- Careful Reading: Read the problem statement multiple times to ensure you grasp all the details.
- Identifying Key Information: Note down the input format, expected output, constraints, and any special conditions.
- Clarifying Ambiguities: If anything is unclear, don’t hesitate to ask for clarification. In a real interview, this shows your attention to detail.
Interactive Checklist: Problem Understanding
- Read the problem statement thoroughly
- Identify input format and constraints
- Understand the expected output
- Note any special conditions or edge cases
- Clarify any ambiguities
Planning Your Approach
Once you understand the problem, it’s time to plan your approach. This involves:
- Breaking Down the Problem: Divide the problem into smaller, manageable sub-problems.
- Considering Multiple Solutions: Brainstorm different approaches to solve the problem.
- Evaluating Trade-offs: Consider the pros and cons of each approach in terms of time complexity, space complexity, and implementation difficulty.
Writing Pseudocode
Before diving into coding, it’s often helpful to write pseudocode. This step:
- Helps organize your thoughts
- Provides a roadmap for implementation
- Makes it easier to spot logical errors early
Here’s an example of pseudocode for a simple sorting algorithm:
function bubbleSort(array):
for i from 1 to length(array):
for j from 0 to length(array) - i:
if array[j] > array[j+1]:
swap array[j] and array[j+1]
return array
Implementing the Solution
When implementing your solution:
- Start with a Basic Implementation: Begin with a simple, working solution before optimizing.
- Follow Good Coding Practices: Write clean, readable code with meaningful variable names and comments.
- Handle Edge Cases: Consider and handle all possible edge cases in your implementation.
Testing and Debugging
Thorough testing is crucial for ensuring your solution works correctly:
- Use Sample Test Cases: Start with the provided sample test cases.
- Create Additional Test Cases: Develop your own test cases, including edge cases.
- Implement Unit Tests: If time allows, write unit tests for your functions.
- Debug Systematically: When encountering errors, use debugging techniques like print statements or a debugger to identify the issue.
Optimizing for Time and Space Complexity
After implementing a working solution, consider optimizing it:
- Analyze Current Complexity: Determine the time and space complexity of your current solution.
- Identify Bottlenecks: Look for areas where performance can be improved.
- Apply Optimization Techniques: Use techniques like memoization, dynamic programming, or more efficient data structures to improve performance.
Interactive Time Complexity Calculator
Leveraging AI Tools in Coding Challenges
As AI continues to evolve, it’s becoming increasingly relevant in coding challenges:
- AI-Powered Code Completion: Tools like GitHub Copilot can suggest code snippets and help you write code faster.
- AI-Assisted Debugging: Some AI tools can help identify potential bugs or suggest optimizations.
- Learning from AI-Generated Solutions: While it’s important to develop your own problem-solving skills, studying AI-generated solutions can provide insights into efficient coding techniques.
AI is not a substitute for human problem-solving skills, but rather a tool to enhance them. The key is to use AI responsibly to augment your own understanding and capabilities.
Dr. Jane Smith, AI Ethics Researcher
Remember, while AI tools can be helpful, it’s crucial to understand the underlying concepts and be able to solve problems independently, especially in interview situations.
By following these strategies, you’ll be well-equipped to tackle a wide range of coding challenges. Practice applying these techniques consistently, and you’ll see improvement in your problem-solving skills and coding efficiency over time.
Common Algorithms and Data Structures in Coding Challenges
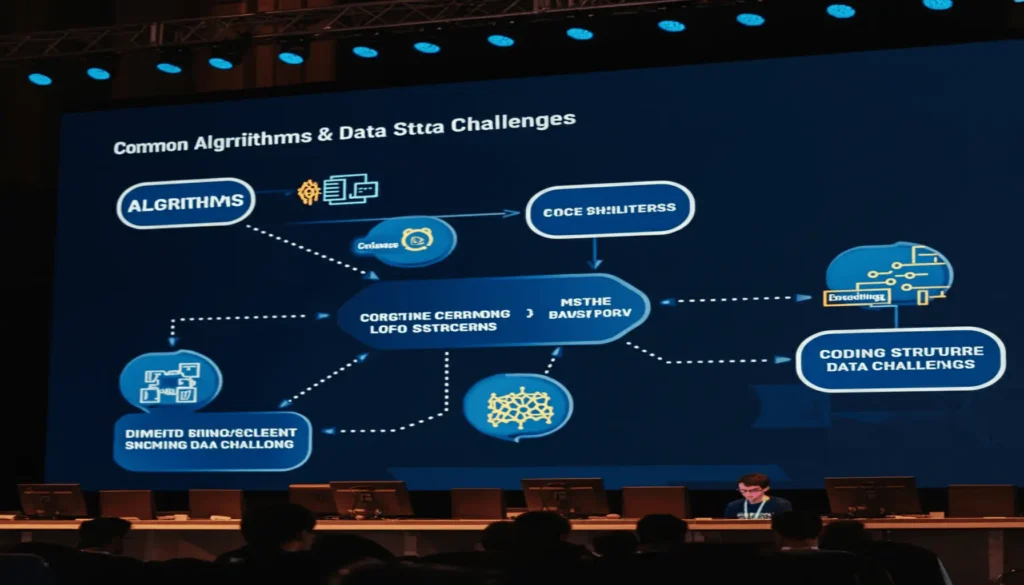
Mastering common algorithms and data structures is crucial for excelling in coding challenges. These fundamental building blocks form the backbone of efficient problem-solving in computer science. Let’s delve into the most frequently encountered algorithms and data structures in coding challenges, understanding their applications and why they’re essential for every developer.
Arrays and Strings
Arrays and strings are fundamental data structures that appear in a wide variety of coding challenges. They’re often the first line of defense in solving problems efficiently.
Arrays are contiguous blocks of memory that store elements of the same type. They offer constant-time access to elements by index, making them ideal for many problems involving sequences or collections of data.
Strings, in most programming languages, are essentially arrays of characters with some additional built-in functionalities.
Common array and string techniques in coding challenges include:
- Two-pointer technique
- Sliding window
- Prefix sum
- Kadane’s algorithm for maximum subarray
Example: Two-pointer technique to find a pair that sums to a target
def find_pair(arr, target):
left, right = 0, len(arr) - 1
while left < right:
current_sum = arr[left] + arr[right]
if current_sum == target:
return arr[left], arr[right]
elif current_sum < target:
left += 1
else:
right -= 1
return None
Linked Lists
Linked lists are linear data structures where elements are stored in nodes, each pointing to the next node in the sequence. They come in singly-linked, doubly-linked, and circular varieties.
Key operations on linked lists include:
- Insertion and deletion at the beginning, end, or middle
- Reversing a linked list
- Detecting cycles
- Merging sorted linked lists
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverse_linked_list(head):
prev = None
current = head
while current:
next_temp = current.next
current.next = prev
prev = current
current = next_temp
return prev
Stacks and Queues
Stacks and queues are abstract data types that are frequently used in coding challenges, especially those involving parsing or breadth-first search.
- Stacks follow the Last-In-First-Out (LIFO) principle
- Queues follow the First-In-First-Out (FIFO) principle
Common applications include:
- Balancing parentheses
- Implementing undo functionality
- Breadth-first search in graphs
- Evaluating postfix expressions
from collections import deque
# Queue implementation using deque
queue = deque()
queue.append(1) # enqueue
queue.append(2)
front = queue.popleft() # dequeue
# Stack implementation using list
stack = []
stack.append(1) # push
stack.append(2)
top = stack.pop() # pop
Trees and Graphs
Trees and graphs are non-linear data structures that represent hierarchical or network-like relationships between data. They’re crucial in many advanced coding challenges.
Trees are hierarchical structures with a root node and child nodes. Common types include:
- Binary trees
- Binary search trees (BST)
- AVL trees
- Red-black trees
Graphs represent a set of vertices connected by edges. They can be directed or undirected, weighted or unweighted.
Key algorithms for trees and graphs include:
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
- Dijkstra’s algorithm for shortest paths
- Kruskal’s and Prim’s algorithms for minimum spanning trees
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
result = []
if root:
result.extend(inorder_traversal(root.left))
result.append(root.val)
result.extend(inorder_traversal(root.right))
return result
Hash Tables
Hash tables, also known as hash maps or dictionaries, provide average constant-time complexity for insertion, deletion, and lookup operations. They’re incredibly useful in coding challenges for tasks like:
- Counting frequencies
- Checking for duplicates
- Implementing caches
- Two-sum problems
# Example: Two-sum problem using hash table
def two_sum(nums, target):
seen = {}
for i, num in enumerate(nums):
complement = target - num
if complement in seen:
return [seen[complement], i]
seen[num] = i
return []
Dynamic Programming
Dynamic programming (DP) is a powerful technique for solving optimization problems by breaking them down into simpler subproblems. It’s often used in coding challenges involving:
- Fibonacci sequences
- Longest common subsequence
- Knapsack problems
- Shortest path in a weighted graph
The key to solving DP problems is identifying the optimal substructure and overlapping subproblems.
# Example: Fibonacci sequence using dynamic programming
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
Sorting and Searching Algorithms
Efficient sorting and searching are fundamental to many coding challenges. Common sorting algorithms include:
- Quicksort
- Mergesort
- Heapsort
- Bubble sort (for educational purposes)
Searching algorithms often encountered in coding challenges:
- Binary search
- Linear search
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
Here’s a comparison of time complexities for common sorting algorithms:
Algorithm | Best Case | Average Case | Worst Case | Space Complexity |
Quicksort | O(n log n) | O(n log n) | O(n²) | O(log n) |
Mergesort | O(n log n) | O(n log n) | O(n log n) | O(n) |
Heapsort | O(n log n) | O(n log n) | O(n log n) | O(1) |
Bubble Sort | O(n) | O(n²) | O(n²) | O(1) |
# Example: Binary search implementation
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
Interactive Sorting Visualizer
Understanding these common algorithms and data structures is crucial for success in coding challenges. They form the foundation upon which more complex solutions are built. As you practice coding challenges, you’ll find that many problems can be solved by applying these fundamental concepts in creative ways.
Remember, the key to mastering these concepts is consistent practice. Platforms like LeetCode, HackerRank, and Codewars offer a wide range of problems that will help you solidify your understanding of these algorithms and data structures.
Learn more about algorithms and data structures on GeeksforGeeks
By focusing on these fundamental building blocks, you’ll be well-equipped to tackle a wide range of coding challenges, from basic algorithmic puzzles to complex system design problems. In the next section, we’ll explore strategies for approaching coding challenges effectively, helping you put these concepts into practice.
Tips for Excelling at Coding Challenges

Mastering coding challenges requires more than just technical knowledge; it demands a strategic approach, consistent practice, and the right mindset. In this section, we’ll explore essential tips to help you excel in coding challenges, whether you’re tackling them for personal growth, interview preparation, or competitive programming.
Practice Regularly
The adage “practice makes perfect” holds especially true for coding challenges. Regular practice is crucial for honing your problem-solving skills and becoming familiar with various types of challenges. Here’s how to make the most of your practice sessions:
- Set a daily goal: Aim to solve at least one coding problem each day. This consistency will help build your skills over time.
- Vary the difficulty: Mix easy, medium, and hard problems to challenge yourself and prevent boredom.
- Use multiple platforms: Leverage platforms like LeetCode, HackerRank, and Codewars to expose yourself to different problem types and formats.
- Track your progress: Keep a log of the problems you’ve solved and revisit challenging ones periodically to reinforce your learning.
Interactive Practice Tracker
Learn from Others’ Solutions
While it’s important to solve problems on your own, there’s immense value in studying solutions from other programmers. This practice can expose you to new approaches, optimizations, and coding techniques. Here’s how to effectively learn from others:
- After solving a problem, look at the top-rated or most efficient solutions.
- Analyze the differences between your approach and others’ solutions.
- Implement the alternative solutions yourself to understand them better.
- Participate in coding communities like Stack Overflow or Reddit’s r/learnprogramming to discuss problems and solutions.
Time Management During Challenges
Effective time management is crucial, especially in timed coding challenges or technical interviews. Here are strategies to optimize your time:
- Read and understand the problem thoroughly before starting to code.
- Plan your approach: Spend a few minutes outlining your solution before implementation.
- Start with a brute force solution if you’re stuck, then optimize if time permits.
- Set time limits for each phase of problem-solving (understanding, planning, coding, testing).
- Practice with a timer to get comfortable working under time constraints.
Phase | Recommended Time Allocation |
Understanding the problem | 5-10% of total time |
Planning the approach | 15-20% of total time |
Coding the solution | 50-60% of total time |
Testing and debugging | 15-20% of total time |
Handling Stress and Pressure
Coding challenges, especially in interview settings, can be stressful. Here are techniques to manage stress and perform well under pressure:
- Practice mindfulness or meditation to improve focus and reduce anxiety.
- Simulate interview conditions during practice sessions to build familiarity.
- Focus on the process, not just the outcome, to reduce performance anxiety.
- Take deep breaths if you feel overwhelmed during a challenge.
- Remember that it’s okay to ask for clarification or take a moment to think.
Improving Your Coding Speed
While accuracy is paramount, improving your coding speed can give you a significant advantage in time-constrained challenges:
- Master your IDE or text editor: Learn keyboard shortcuts and efficient navigation techniques.
- Practice typing: Use tools like TypeRacer or 10FastFingers to improve your typing speed.
- Create code snippets for common algorithms and data structures.
- Learn to write clean code quickly: Practice writing readable code from the start, rather than writing messy code and cleaning it up later.
Mastering the Fundamentals of Your Chosen Programming Language
A deep understanding of your primary programming language is essential for excelling in coding challenges:
- Study the language’s core concepts and best practices.
- Familiarize yourself with the standard library to avoid reinventing the wheel.
- Practice implementing common data structures and algorithms from scratch.
- Stay updated with new language features and updates.
- Read high-quality code in your chosen language to learn idiomatic practices.
Language Proficiency Tracker
By implementing these tips and consistently working on your skills, you’ll be well-equipped to excel in coding challenges. Remember, success in coding challenges is not just about raw coding ability, but also about strategy, time management, and maintaining a positive mindset under pressure.
For more advanced tips on excelling in coding challenges, check out this comprehensive guide from TopCoder, a platform known for its high-level competitive programming contests.
Coding Challenge Etiquette and Best Practices
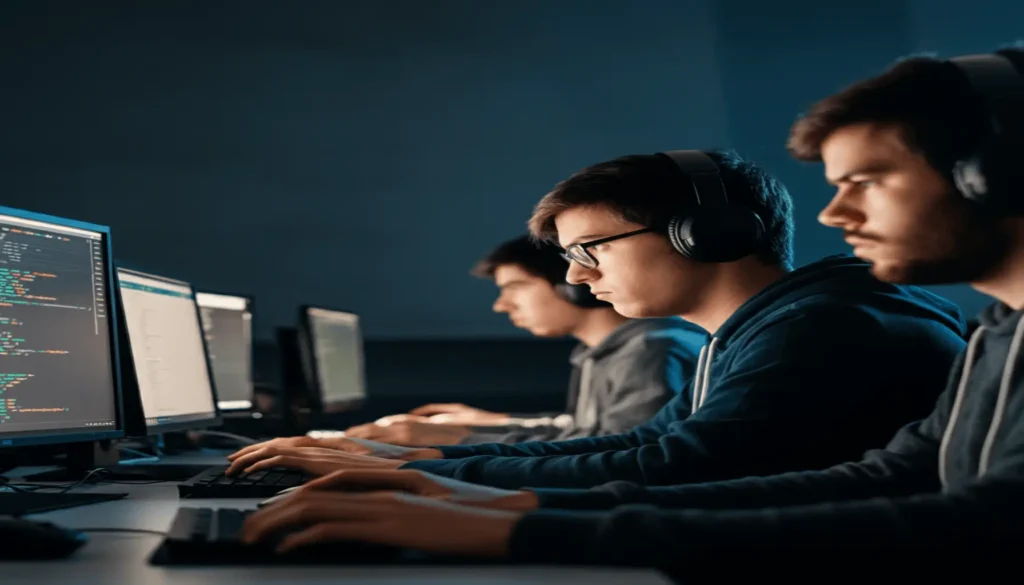
Mastering coding challenges isn’t just about solving problems efficiently; it’s also about adhering to best practices and professional etiquette. These skills are crucial not only for performing well in challenges but also for succeeding in real-world software development environments. Let’s explore the key aspects of coding challenge etiquette and best practices that will set you apart in the world of coding challenges.
Following Instructions Carefully
One of the most fundamental yet often overlooked aspects of tackling coding challenges is following instructions meticulously. Many participants lose points or fail challenges not because they lack technical skills, but because they misread or ignore crucial details in the problem statement.
Best practices for following instructions:
- Read the entire problem statement before starting to code
- Highlight or note down key requirements and constraints
- Double-check input/output formats and data types
- Review any provided sample inputs and outputs
- Check for time and space complexity requirements
Interactive Checklist: Following Challenge Instructions
Writing Clean, Readable Code
In coding challenges, it’s not just about getting the right answer; how you arrive at the solution matters too. Clean, readable code demonstrates your proficiency as a programmer and makes your solutions easier to understand and maintain.
Tips for writing clean code:
- Use meaningful variable and function names
- Maintain consistent indentation and formatting
- Break down complex logic into smaller, manageable functions
- Avoid unnecessary comments; let your code speak for itself
- Follow the DRY (Don’t Repeat Yourself) principle
- Adhere to language-specific style guides (e.g., PEP 8 for Python)
Here’s an example of clean, readable code in Python:
def find_max_subarray_sum(nums):
"""
Find the maximum sum of a contiguous subarray within a one-dimensional array of numbers.
Args:
nums (list): A list of integers
Returns:
int: The maximum sum of a contiguous subarray
"""
max_sum = current_sum = nums[0]
for num in nums[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
Commenting and Documenting Your Code
While clean code should be self-explanatory to a large extent, proper commenting and documentation are still crucial, especially in coding challenges where reviewers need to quickly understand your approach.
Best practices for commenting and documenting:
- Use docstrings to explain function purpose, parameters, and return values
- Comment on complex algorithms or non-obvious solutions
- Avoid over-commenting; focus on the ‘why’ rather than the ‘what’
- Use inline comments sparingly, only for particularly tricky lines
- Consider adding a brief overview comment at the beginning of your solution
Handling Edge Cases
A mark of a great programmer is their ability to anticipate and handle edge cases. In coding challenges, this skill can set you apart from other participants.
Strategies for handling edge cases:
- Consider extreme inputs (e.g., empty arrays, very large numbers)
- Test with negative numbers, zero, and positive numbers
- Check for potential overflow or underflow situations
- Handle invalid inputs gracefully
- Use assert statements to validate assumptions
Here’s an example of handling edge cases in a function:
def divide_numbers(a, b):
"""
Divide two numbers and handle potential edge cases.
Args:
a (float): The dividend
b (float): The divisor
Returns:
float: The result of a divided by b
Raises:
ValueError: If b is zero
"""
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
Asking Clarifying Questions
In both coding challenges and real-world scenarios, asking the right questions is a valuable skill. Don’t hesitate to seek clarification when faced with ambiguity in a problem statement.
Guidelines for asking clarifying questions:
- Read the entire problem statement before asking questions
- Be specific and concise in your queries
- Demonstrate your understanding of the problem in your question
- Ask about edge cases or potential ambiguities
- If possible, provide examples to illustrate your question
Ethical Considerations in Using AI Tools for Coding Challenges
As AI tools become more prevalent in coding, it’s important to consider the ethical implications of their use in coding challenges.
Ethical guidelines for using AI in coding challenges:
- Transparency: Always disclose if you’ve used AI tools in your solution
- Understanding: Ensure you comprehend the AI-generated code before submitting
- Originality: Use AI as a learning tool rather than a solution generator
- Fairness: Adhere to the rules of the challenge regarding AI usage
- Learning: Focus on understanding the concepts behind AI-generated solutions
Interactive Poll: AI Usage in Coding Challenges
By adhering to these best practices and ethical considerations, you’ll not only perform better in coding challenges but also develop habits that will serve you well throughout your programming career. Remember, the goal of these challenges is not just to solve problems, but to grow as a developer and demonstrate your skills to potential employers or collaborators.
As we continue to navigate the evolving landscape of coding challenges, it’s crucial to stay informed about the latest trends and best practices. In the next section, we’ll explore real-world examples of coding challenges and analyze successful solutions to help you further refine your approach.
Real-world Examples of Coding Challenges

In this section, we’ll dive into real-world examples of coding challenges, examining case studies from top tech companies, analyzing successful solutions, and exploring common pitfalls. By understanding these practical scenarios, you’ll be better equipped to tackle similar challenges in your own coding journey.
Case Studies from Top Tech Companies
Let’s explore how some of the leading tech companies use coding challenges in their hiring processes and internal skill development programs.
Google’s Code Jam
Google’s annual Code Jam competition is one of the most prestigious coding contests in the world. It attracts hundreds of thousands of participants each year, challenging them with complex algorithmic problems.
Example Challenge: “Bathroom Stalls”
In this challenge, participants were asked to solve a problem involving the optimal placement of people in bathroom stalls to maximize privacy. The problem required a combination of mathematical thinking and efficient coding.
Facebook’s Hacker Cup
Facebook’s Hacker Cup is another high-profile coding competition that tests participants’ skills in algorithms, data structures, and problem-solving.
Example Challenge: “Tourist”
This challenge involved optimizing a tourist’s route through a city to visit the maximum number of attractions within a given time limit. The problem required graph algorithms and dynamic programming techniques.
Amazon’s Online Assessment
Amazon uses coding challenges as part of its hiring process for software engineering positions.
Example Challenge: “Longest Palindromic Substring”
Candidates are often asked to implement an algorithm to find the longest palindromic substring in a given string. This problem tests knowledge of string manipulation and dynamic programming.
Interactive Chart: Popularity of Coding Challenges in Tech Hiring
This chart illustrates the prevalence of coding challenges in the hiring processes of major tech companies. As we can see, coding challenges play a significant role across the industry, with Google leading the pack in their utilization of these assessments.
Anonymized Examples of Successful Solutions
Let’s examine some anonymized examples of successful solutions to common coding challenges:
Two Sum Problem
Challenge: Given an array of integers and a target sum, return indices of the two numbers such that they add up to the target.
Successful Solution:
def two_sum(nums, target):
seen = {}
for i, num in enumerate(nums):
complement = target - num
if complement in seen:
return [seen[complement], i]
seen[num] = i
return []
This solution uses a hash map to achieve O(n) time complexity, demonstrating efficient use of data structures.
Reverse a Linked List
Challenge: Reverse a singly linked list.
Successful Solution:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverse_list(head):
prev = None
current = head
while current:
next_temp = current.next
current.next = prev
prev = current
current = next_temp
return prev
This iterative solution efficiently reverses the linked list in-place, showcasing a good understanding of pointer manipulation.
Common Pitfalls and How to Avoid Them
When tackling coding challenges, developers often encounter several common pitfalls. Here’s how to recognize and avoid them:
- Misunderstanding the Problem
- Pitfall: Rushing to code without fully grasping the problem statement.
- Solution: Read the problem carefully, ask clarifying questions, and solve a small example by hand before coding.
- Inefficient Algorithms
- Pitfall: Using brute-force approaches that don’t scale for large inputs.
- Solution: Always consider the time and space complexity of your solution. Look for opportunities to optimize using appropriate data structures and algorithms.
- Neglecting Edge Cases
- Pitfall: Failing to consider boundary conditions or special inputs.
- Solution: Always test your code with edge cases such as empty inputs, single-element inputs, and maximum/minimum values.
- Poor Code Organization
- Pitfall: Writing monolithic, hard-to-read code.
- Solution: Break your solution into smaller, well-named functions. Use meaningful variable names and add comments for complex logic.
- Lack of Testing
- Pitfall: Submitting code without thorough testing.
- Solution: Write test cases covering various scenarios. Use print statements or a debugger to verify your code’s behavior.
Analysis of Winning Solutions from Major Coding Competitions
Let’s analyze some winning solutions from major coding competitions to understand what sets them apart:
Google Code Jam 2023 Winner
The winning solution for the “Colliding Encoding” problem demonstrated:
- Efficient use of hash tables for quick lookups
- Clear, modular code structure
- Thorough handling of edge cases
- Optimal time complexity, crucial for solving multiple test cases quickly
Facebook Hacker Cup 2023 Champion
The champion’s solution for the “Today’s Bonus” challenge showcased:
- Advanced use of dynamic programming
- Clever mathematical optimizations
- Efficient memory management for large inputs
- Clear commenting explaining the approach and key steps
Key takeaways from these winning solutions include:
- Efficiency: Top solutions often achieve the optimal time and space complexity.
- Clarity: Despite being complex, winning code is usually well-organized and readable.
- Robustness: Champions consistently handle all edge cases and potential errors.
- Innovation: Many top solutions feature creative approaches or clever optimizations.
Interactive Table: Characteristics of Winning Solutions
Characteristic | Description | Importance (1-10) |
---|---|---|
Efficiency | Optimal time and space complexity | |
Clarity | Well-organized and readable code | |
Robustness | Handling of all edge cases and errors | |
Innovation | Creative approaches and clever optimizations |
This interactive table allows you to rate the importance of different characteristics in winning solutions. Adjust the numbers to reflect your own experience or perceptions of what makes a solution successful in coding competitions.
By studying these real-world examples, understanding common pitfalls, and analyzing winning solutions, you can significantly improve your approach to coding challenges. Remember, success in these challenges isn’t just about solving the problem—it’s about doing so efficiently, clearly, and robustly.
Explore more coding challenge examples on LeetCode
As you continue your journey in mastering coding challenges, keep these examples and insights in mind. Practice regularly, learn from your mistakes, and always strive to improve the efficiency and clarity of your solutions. With persistence and the right approach, you’ll be well on your way to excelling in coding challenges and advancing your career in software development.
Preparing for Coding Challenges in Job Interviews
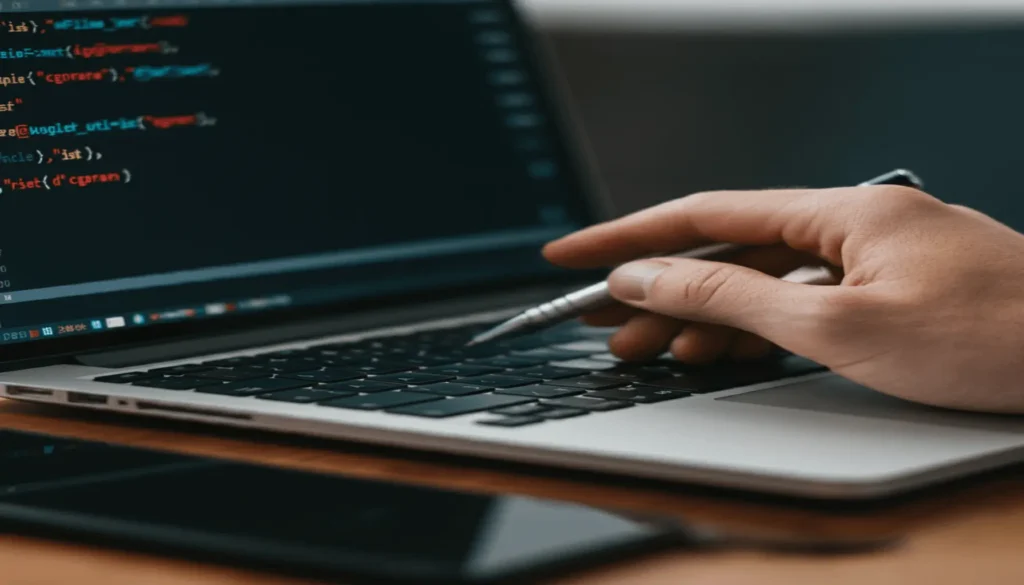
As coding challenges increasingly become a standard part of the tech recruitment process, it’s crucial for developers to be well-prepared for these assessments during job interviews. This section will guide you through what to expect, how to practice effectively, and strategies for balancing coding challenges with other aspects of interview preparation.
What to Expect in a Coding Interview
Coding interviews typically involve solving one or more programming problems in real-time, either on a whiteboard or using a shared coding environment. Here’s what you can generally expect:
- Problem-Solving: You’ll be presented with one or more algorithmic problems to solve.
- Time Constraints: Most coding challenges are time-limited, usually ranging from 30 minutes to 2 hours.
- Language Choice: You’ll often be allowed to choose your preferred programming language.
- Discussion: Interviewers may ask you to explain your thought process and discuss potential optimizations.
- Follow-up Questions: Be prepared for additional questions about your solution, such as time and space complexity analysis.
Interactive Flowchart: Typical Coding Interview Process
How to Practice Effectively
To excel in coding challenges during job interviews, consistent and targeted practice is key. Here are some strategies to help you prepare effectively:
- Regular Practice: Aim to solve at least one coding problem daily. Platforms like LeetCode, HackerRank, and Codewars offer a wide range of problems to practice on.
- Time Your Practice: Simulate interview conditions by setting time limits for solving problems.
- Mock Interviews: Participate in mock coding interviews with peers or use platforms like Pramp for realistic practice.
- Review and Reflect: After solving a problem, review other solutions and reflect on ways to optimize your code.
- Focus on Fundamentals: Ensure you have a strong grasp of data structures, algorithms, and time/space complexity analysis.
- Learn from Mistakes: Keep a log of problems you struggle with and revisit them regularly to reinforce learning.
Balancing Coding Challenges with Other Interview Preparation
While coding challenges are crucial, they’re just one part of the interview process. Here’s how to balance your preparation:
Preparation Area | Time Allocation | Key Focus Points |
Coding Challenges | 50% | Algorithms, data structures, problem-solving |
System Design | 20% | Scalability, trade-offs, real-world applications |
Behavioral Questions | 15% | Past experiences, teamwork, conflict resolution |
Company Research | 10% | Company culture, products, recent news |
Questions for Interviewers | 5% | Thoughtful inquiries about the role and company |
Remember, the exact balance may vary depending on the specific role and company you’re interviewing for.
Specific Strategies for Python and JavaScript Coding Challenges
Given the popularity of Python and JavaScript in coding interviews, here are some specific strategies for these languages:
Python Strategies
- Leverage Python’s built-in functions and libraries (e.g., collections, itertools) for efficient solutions.
- Utilize list comprehensions and generator expressions for concise code.
- Be familiar with Python’s specific data structures like deque for optimized operations.
# Example of using a deque for efficient queue operations
from collections import deque
def sliding_window_maximum(nums, k):
result = []
window = deque()
for i, num in enumerate(nums):
while window and window[0] <= i - k:
window.popleft()
while window and nums[window[-1]] < num:
window.pop()
window.append(i)
if i >= k - 1:
result.append(nums[window[0]])
return result
JavaScript Strategies
- Understand JavaScript’s unique features like closures and prototypal inheritance.
- Be comfortable with array methods like map, filter, and reduce.
- Know how to use Set and Map for efficient data manipulation.
// Example of using Set for efficient unique value extraction
function uniqueElements(arr) {
return [...new Set(arr)];
}
// Example of using map and reduce for data transformation
function sumOfSquares(numbers) {
return numbers.map(num => num * num).reduce((sum, square) => sum + square, 0);
}
By focusing on these language-specific strategies, you can optimize your solutions and showcase your expertise during coding interviews.
As you prepare for coding challenges in job interviews, remember that practice and consistency are key. By understanding what to expect, practicing effectively, balancing your preparation, and mastering language-specific strategies, you’ll be well-equipped to tackle any coding challenge that comes your way in your next job interview.
Beyond Coding Challenges: Holistic Developer Skills

While coding challenges are an excellent way to hone your programming skills, it’s crucial to recognize that being a successful developer requires a broader set of competencies. In this section, we’ll explore the holistic skills that complement your coding abilities and contribute to a well-rounded developer profile.
Soft Skills that Complement Coding Abilities
Technical prowess alone is not enough to thrive in the modern software development landscape. Soft skills play a pivotal role in career advancement and effective collaboration. Here are some essential soft skills that every developer should cultivate:
- Communication: The ability to clearly articulate complex technical concepts to both technical and non-technical stakeholders is invaluable. This skill is crucial for effective teamwork, client interactions, and documentation.
- Problem-solving: While coding challenges sharpen your algorithmic problem-solving, real-world software development often requires a broader approach to problem-solving, including gathering requirements, analyzing trade-offs, and making informed decisions.
- Teamwork: Most software projects involve collaboration. Being able to work effectively in a team, share knowledge, and contribute to a positive work environment is essential.
- Adaptability: The tech industry is constantly evolving. Being able to adapt to new technologies, methodologies, and work environments is crucial for long-term success.
- Time Management: Balancing multiple tasks, meeting deadlines, and prioritizing work effectively are skills that distinguish great developers from good ones.
Interactive Chart: Importance of Soft Skills in Software Development
This radar chart illustrates the relative importance of various soft skills in software development. While all these skills are crucial, communication and problem-solving stand out as particularly vital.
Read also : Soft Skills: Key to Unlocking Professional Success
Importance of System Design and Architecture Knowledge
As you progress in your career, you’ll likely encounter more complex projects that require a solid understanding of system design and architecture. This knowledge is essential for:
- Scalability: Designing systems that can handle growth and increased load.
- Maintainability: Creating architectures that are easy to understand, modify, and extend.
- Performance: Optimizing systems for speed and efficiency.
- Reliability: Building robust systems that can handle failures gracefully.
To develop your system design skills:
- Study design patterns and architectural styles (e.g., microservices, monolithic, event-driven).
- Practice designing systems on paper or whiteboard, considering various trade-offs.
- Analyze the architecture of popular open-source projects.
- Participate in system design discussions and code reviews at work.
Learn more about software architecture on the IEEE Software Magazine website
Continuous Learning and Staying Updated with Tech Trends
The tech industry is known for its rapid pace of change. Staying relevant requires a commitment to lifelong learning. Here are some strategies to keep your skills sharp:
- Follow Tech Blogs and News Sites: Stay informed about the latest developments in your field.
- Attend Conferences and Meetups: Network with other professionals and learn about cutting-edge technologies.
- Take Online Courses: Platforms like Coursera, edX, and Udacity offer courses on emerging technologies.
- Contribute to Open Source Projects: Gain practical experience with new tools and technologies.
- Experiment with Side Projects: Apply new concepts in personal projects to deepen your understanding.
Interactive Table: Top Resources for Continuous Learning
Resource Type | Examples | Benefits |
---|---|---|
Tech Blogs | TechCrunch, Hacker News, Medium | Stay updated on industry news and trends |
Online Courses | Coursera, edX, Udacity | Structured learning on specific topics |
Coding Platforms | LeetCode, HackerRank, Codewars | Practice coding skills and algorithms |
Open Source Contributions | GitHub, GitLab | Gain practical experience and collaborate |
Tech Conferences | Google I/O, AWS re:Invent, Apple WWDC | Learn about new technologies and network |
Balancing Competitive Programming with Practical Software Development
While coding challenges and competitive programming can significantly enhance your algorithmic skills, it’s essential to strike a balance with practical software development. Here’s how you can achieve this balance:
- Apply Competitive Programming Skills to Real Projects: Use the optimization techniques and problem-solving strategies you learn from coding challenges in your day-to-day work.
- Focus on Code Quality: In competitive programming, the focus is often on solving problems quickly. In real-world development, code readability, maintainability, and scalability are equally important.
- Collaborate on Open Source Projects: This allows you to apply your skills in a real-world context while contributing to the developer community.
- Develop Full-Stack Skills: While coding challenges often focus on algorithms, practical development requires a broader skill set, including front-end, back-end, and DevOps knowledge.
- Practice System Design: Complement your algorithmic skills with system design practice to prepare for real-world architectural challenges.
By cultivating these holistic skills alongside your coding abilities, you’ll be well-equipped to tackle the diverse challenges of modern software development. Remember, being a great developer goes beyond solving coding challenges – it’s about creating robust, scalable solutions that solve real-world problems.
The best programmers are not marginally better than merely good ones. They are an order-of-magnitude better, measured by whatever standard: conceptual creativity, speed, ingenuity of design, or problem-solving ability.
Randall E. Stross
This quote underscores the importance of developing a well-rounded skill set that goes beyond just coding proficiency. By focusing on holistic development, you can truly excel in your career and make significant contributions to the field of software development.
The Future of Coding Challenges
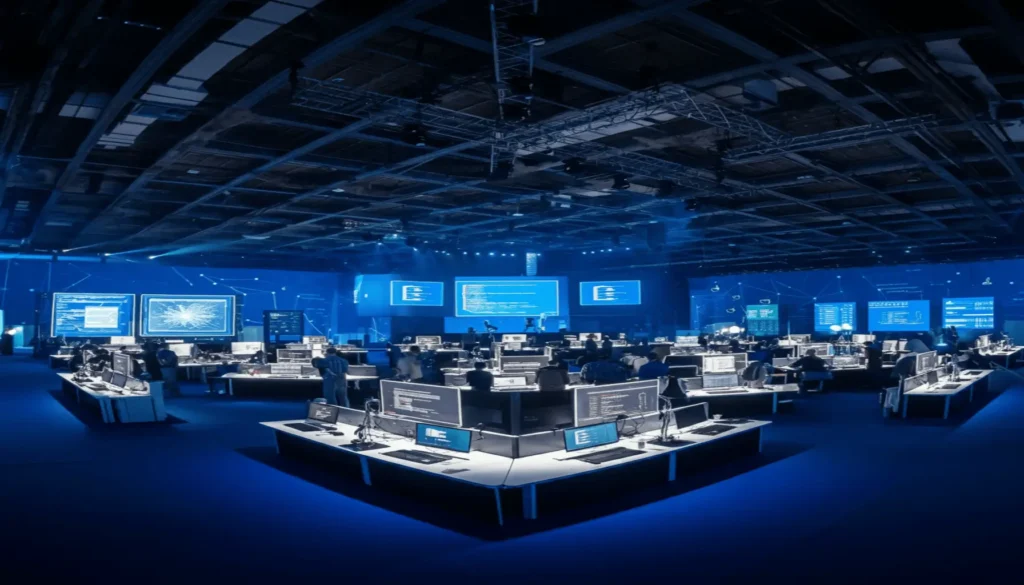
As we look ahead, the landscape of coding challenges is poised for significant transformation. The rapid advancement of technology, particularly in artificial intelligence and machine learning, is reshaping how we approach problem-solving in software development. Let’s explore the emerging trends, potential changes, and predictions that will define the future of coding challenges.
Emerging Trends in Tech Recruitment
The tech recruitment process is evolving, and with it, the role of coding challenges is changing. Here are some key trends to watch:
- Holistic Assessment: Companies are moving beyond pure coding skills to assess a candidate’s problem-solving approach, creativity, and ability to work in a team.
- Real-World Scenarios: Challenges are increasingly based on actual business problems, giving candidates a taste of the work they’ll be doing.
- Continuous Assessment: Some companies are adopting platforms that allow for ongoing skill evaluation, rather than relying solely on one-time coding tests.
- Diverse Skill Sets: With the rise of full-stack development and DevOps practices, challenges are becoming more diverse, covering a wider range of technologies and methodologies.
- Soft Skills Evaluation: Coding challenges are being designed to also assess communication skills, adaptability, and cultural fit.
Interactive Chart: Trends in Tech Recruitment
Potential Changes in Coding Challenge Formats
The format of coding challenges is likely to undergo significant changes in the coming years:
- Collaborative Challenges: We may see more team-based coding challenges that assess how well candidates work together to solve complex problems.
- Adaptive Difficulty: AI-powered platforms could adjust the difficulty of challenges in real-time based on a candidate’s performance.
- Virtual Reality (VR) and Augmented Reality (AR): These technologies could create immersive coding environments, allowing for more interactive and engaging challenges.
- Cross-Disciplinary Challenges: As technology becomes more integrated into various industries, we might see coding challenges that combine programming with domain-specific knowledge in fields like finance, healthcare, or environmental science.
- Gamification: More platforms are likely to incorporate game-like elements to make coding challenges more engaging and enjoyable.
The Role of AI in Coding Assessments
Artificial Intelligence is set to play a pivotal role in the future of coding challenges:
- Automated Code Review: AI can provide instant feedback on code quality, efficiency, and best practices.
- Natural Language Processing (NLP): Challenges might involve coding in natural language, with AI interpreting and executing the instructions.
- Personalized Learning Paths: AI can analyze a developer’s strengths and weaknesses to create tailored challenge sequences for optimal skill improvement.
- Bias Reduction: AI algorithms can help reduce unconscious bias in the assessment process by focusing solely on the code and its performance.
- AI-Generated Challenges: We may see AI creating unique, contextually relevant coding challenges tailored to specific job requirements or skill levels.
Predictions for Coding Challenges in the Next 5 Years
Looking ahead to 2029, here are some predictions for the evolution of coding challenges:
- Integration with Continuous Learning: Coding challenges will be more tightly integrated with ongoing professional development, becoming a regular part of a developer’s career journey.
- Focus on Ethical Coding: Challenges will increasingly incorporate scenarios that test a developer’s ability to write ethical, inclusive, and accessible code.
- Quantum Computing Challenges: As quantum computing becomes more prevalent, we’ll see the emergence of coding challenges specific to this field.
- Blockchain and Cryptocurrency: More challenges will focus on blockchain technology and cryptocurrency development as these areas continue to grow.
- IoT and Edge Computing: Challenges related to Internet of Things (IoT) devices and edge computing will become more common as these technologies proliferate.
Interactive Prediction Meter
As we can see from these predictions, the future of coding challenges is likely to be more diverse, integrated, and technologically advanced than ever before. Developers will need to stay adaptable and continue learning to keep up with these evolving trends.
In conclusion, the future of coding challenges is bright and full of possibilities. As technology continues to advance at a rapid pace, these challenges will play an increasingly important role in shaping the skills and careers of developers worldwide. By staying informed about these trends and predictions, you can better prepare yourself for the coding challenges of tomorrow and position yourself for success in the ever-evolving tech industry.
Leveraging Coding Communities to Improve Skills

In the world of coding challenges and software development, the power of community cannot be overstated. Engaging with fellow developers can significantly accelerate your learning, expose you to new ideas, and provide invaluable support throughout your coding journey. Let’s explore how you can leverage coding communities to enhance your skills and excel in coding challenges.
Participating in Online Forums and Discussions
Online forums and discussion platforms are treasure troves of knowledge for developers at all levels. Websites like Stack Overflow, Reddit’s programming subreddits, and specialized forums for specific programming languages or technologies offer opportunities to:
- Ask questions and get help with specific coding problems
- Share your knowledge by answering others’ questions
- Engage in discussions about best practices and emerging trends
- Stay updated on the latest coding challenges and competitions
Pro Tip: When participating in online discussions, always strive to be clear, respectful, and constructive. Provide context for your questions and, when possible, share what you’ve already tried. This approach will help you get more meaningful responses and build a positive reputation within the community.
Contributing to Open-Source Projects
Open-source contributions are an excellent way to improve your coding skills, gain real-world experience, and build your professional network. Here’s how you can get started:
- Find a project: Platforms like GitHub, GitLab, and Bitbucket host countless open-source projects. Look for projects that align with your interests and skill level.
- Start small: Begin with small contributions like fixing typos in documentation or addressing minor bugs.
- Read the contribution guidelines: Each project has its own set of guidelines for contributors. Make sure to follow them closely.
- Submit pull requests: Once you’ve made improvements or additions, submit a pull request for review.
- Be open to feedback: Maintainers may suggest changes to your code. View this as a learning opportunity.
Contributing to open-source projects not only improves your coding skills but also exposes you to different coding styles and collaborative workflows, which are crucial for success in coding challenges and professional development.
Attending Local Meetups and Conferences
While online communities are valuable, face-to-face interactions at local meetups and conferences offer unique benefits:
- Networking opportunities: Meet like-minded developers and potential mentors in your area.
- Hands-on workshops: Many events offer practical sessions where you can learn new skills or technologies.
- Inspirational talks: Gain insights from industry experts and thought leaders.
- Job opportunities: Many companies use these events for recruitment.
Interactive Map: Find Coding Meetups Near You
To find coding meetups in your area, you can use platforms like Meetup.com or Eventbrite. Many tech companies and coding bootcamps also host regular events that are open to the public.
Seeking Feedback and Mentorship
Feedback and mentorship are crucial for growth in any field, and coding is no exception. Here’s how you can seek valuable input on your coding skills:
- Code reviews: Ask experienced developers to review your code and provide constructive feedback.
- Pair programming: Collaborate with other developers to solve problems together, learning from each other’s approaches.
- Mentorship programs: Look for formal mentorship programs in your workplace or through professional organizations.
- Online mentorship platforms: Websites like Codementor connect learners with experienced developers for one-on-one guidance.
Remember, mentorship is a two-way street. Be prepared to offer value to your mentor as well, whether through your unique perspective, enthusiasm, or by paying it forward to other learners in the future.
Sharing Your Own Solutions and Insights
As you progress in your coding journey, don’t forget the importance of giving back to the community. Sharing your knowledge not only helps others but also reinforces your own understanding. Consider:
- Writing blog posts: Start a technical blog to share your experiences, solutions to coding challenges, and insights into programming concepts.
- Creating tutorials: Video tutorials or written guides can help others learn from your expertise.
- Speaking at meetups or conferences: Share your experiences and knowledge with a wider audience.
- Mentoring junior developers: As you gain experience, consider mentoring others who are just starting their coding journey.
Interactive Poll: How Do You Engage with Coding Communities?
By actively engaging with coding communities, you’ll not only improve your skills but also build a network of peers and mentors who can support you throughout your career. Remember, the tech community thrives on collaboration and shared knowledge. Your participation not only benefits you but also contributes to the growth and innovation of the entire field.
As you continue your journey in mastering coding challenges, keep in mind that your community engagement can be just as valuable as your individual practice. The insights, feedback, and connections you gain from these interactions will prove invaluable as you tackle increasingly complex coding challenges and advance in your career.
Tools and Resources to Speed Up the Coding Process

In the fast-paced world of coding challenges, efficiency is key. The right tools and resources can significantly enhance your coding speed and productivity, giving you an edge in competitive programming and technical interviews. Let’s explore some essential tools that can help you streamline your coding process and tackle programming challenges more effectively.
Integrated Development Environments (IDEs)
IDEs are the cornerstone of efficient coding, offering a comprehensive suite of tools for writing, debugging, and managing code. Here are some top IDEs that can boost your productivity:
- Visual Studio Code (VS Code):
- Highly customizable with a vast extension marketplace
- Intelligent code completion and syntax highlighting
- Built-in Git integration for version control
- Lightweight and fast, suitable for coding challenges
- JetBrains IDEs:
- Language-specific IDEs like IntelliJ IDEA (Java), PyCharm (Python), and WebStorm (JavaScript)
- Advanced code refactoring tools
- Powerful debugging features
- Ideal for larger projects and complex coding challenges
Interactive Comparison: Popular IDEs for Coding Challenges
Feature | VS Code | IntelliJ IDEA | PyCharm |
---|---|---|---|
Language Support | Multi-language | Java-focused | Python-focused |
Customization | High | Medium | Medium |
Performance | Fast | Resource-intensive | Resource-intensive |
Debugging Tools | Good | Excellent | Excellent |
Code Assistants and AI Tools
AI-powered coding assistants are revolutionizing the way developers approach coding challenges. These tools can help you write code faster, suggest optimizations, and even generate entire functions based on natural language descriptions.
- GitHub Copilot:
- AI-powered code completion
- Generates code snippets and entire functions
- Learns from your coding style and preferences
- Tabnine:
- AI-driven code completion for multiple languages
- Integrates with various IDEs and text editors
- Offers both local and cloud-based AI models for privacy and performance
AI coding assistants like GitHub Copilot have increased my productivity by at least 30% when tackling complex coding challenges.
Sarah Chen, Senior Software Engineer at TechCorp
Version Control Systems
Version control is crucial for managing your code, especially when working on complex coding challenges or collaborating with others.
- Git:
- Distributed version control system
- Enables branching and merging for experimenting with different solutions
- Integrates with platforms like GitHub and GitLab for collaboration and code sharing
- GitHub/GitLab:
- Web-based Git repository hosting
- Offers features like issue tracking and project management
- Facilitates code reviews and collaboration
Project Management Tools
Effective project management is essential for organizing your approach to coding challenges, especially in team competitions or long-term projects.
- ClickUp:
- All-in-one project management solution
- Customizable workflows and task management
- Time tracking and productivity analytics
- Trello:
- Visual, board-based project management
- Simple and intuitive interface
- Great for personal task management and small team collaboration
Time Management and Productivity Tools
Managing your time effectively is crucial when tackling coding challenges, especially those with time constraints.
- Timeular:
- Physical time tracking device and software
- Helps monitor how you spend your coding time
- Provides insights to improve productivity
- Pomodoro Technique Apps (e.g., Focus Keeper, Pomodone):
- Implement the Pomodoro Technique for focused work sessions
- Help maintain concentration and prevent burnout
- Useful for breaking down complex coding challenges into manageable chunks
Interactive Pomodoro Timer
Testing and Debugging Tools
Efficient testing and debugging are crucial for solving coding challenges quickly and accurately.
- Postman:
- API testing and development platform
- Useful for challenges involving web services and APIs
- Supports automated testing and documentation
- SonarLint:
- IDE extension for real-time code quality and security feedback
- Helps catch and fix issues early in the coding process
- Supports multiple programming languages
By leveraging these tools and resources, you can significantly speed up your coding process and improve your performance in coding challenges. Remember, the key is to find the right balance of tools that work best for your coding style and the specific challenges you’re tackling.
As you explore these tools, keep in mind that the most important asset in solving coding challenges is your problem-solving ability. These tools are meant to enhance your skills, not replace them. Practice regularly, stay curious, and always be open to learning new techniques and technologies.
Discover more coding tools and resources on GitHub
Common Pitfalls When Solving Coding Challenges Under Time Pressure

When faced with coding challenges under time constraints, many developers fall into common traps that can hinder their performance. Recognizing and avoiding these pitfalls is crucial for success in competitive programming and technical interviews. Let’s explore these common mistakes and discuss strategies to overcome them.
Rushing Without Understanding the Problem
One of the most frequent errors developers make is diving into coding without fully grasping the problem at hand. This eagerness to start writing code can lead to misinterpretation and wasted time.
How to avoid this pitfall:
- Take a deep breath and read the problem statement carefully, multiple times if necessary.
- Break down the problem into smaller, manageable parts.
- Identify the input, expected output, and any constraints or edge cases mentioned.
- If allowed, ask clarifying questions to ensure you understand all aspects of the problem.
Poor Time Management
Time management is critical in coding challenges. Many candidates spend too much time on one part of a problem or get stuck on difficult sections, leaving insufficient time to complete the challenge.
Strategies for better time management:
- Allocate specific time limits for each section of the problem and stick to them.
- If you encounter a difficult part, consider moving on and returning to it later if time permits.
- Use the “5-minute rule”: If you can’t make progress on a problem within 5 minutes, move on to the next one.
Interactive Time Management Tool for Coding Challenges
Neglecting Edge Cases
Failing to consider edge cases can lead to incomplete solutions that fail during testing. This oversight can cost you valuable points in competitions or leave a poor impression in interviews.
Best practices for handling edge cases:
- Make it a habit to identify and test edge cases as you develop your solution.
- Consider extreme inputs: very large numbers, empty arrays, null values, etc.
- Test your code with both valid and invalid inputs to ensure robustness.
Overcomplicating Solutions
In an attempt to showcase their skills, some developers create overly complex solutions instead of simpler, more effective ones. This can lead to bugs, difficulty in debugging, and time wastage.
Tips for keeping solutions simple:
- Aim for simplicity in your initial approach.
- Focus on delivering a correct solution first, then consider optimizations if time allows.
- Remember, a straightforward solution that works is better than a complex one that doesn't.
Skipping Testing and Debugging
Under time pressure, many forget to test their code incrementally, which can lead to a pile of errors that are hard to track down later.
Effective testing and debugging strategies:
- Implement a habit of testing your code as you write it.
- Use print statements, assertions, or unit tests to validate functionality regularly.
- If possible, use an IDE with debugging tools to catch errors early.
Ignoring Communication
In a coding interview or collaborative environment, failing to articulate your thought process can hinder your performance and the interviewer's ability to assist you.
How to improve communication during challenges:
- Talk through your thought process as you code.
- Explain your reasoning, the steps you're taking, and any challenges you encounter.
- If stuck, don't hesitate to ask for hints or clarification.
Getting Discouraged by Mistakes
Making small mistakes, such as typos or syntax errors, can lead to frustration and a loss of focus. It's crucial to maintain composure and a positive mindset.
Strategies for maintaining confidence:
- Recognize that mistakes are part of the coding process, especially under pressure.
- Take a deep breath and analyze errors calmly.
- Learn from each mistake and use it to improve your future performance.
Common Pitfalls in Coding Challenges: Self-Assessment
Rate how often you encounter these pitfalls (1-5, where 1 is rarely and 5 is very often):
By being aware of these common pitfalls and implementing strategies to address them, you can significantly improve your performance in coding challenges under time pressure. Remember, practice makes perfect. The more you engage with coding challenges, the better you'll become at managing these potential issues.
For more insights on mastering coding challenges, check out these resources:
Remember, the key to success in coding challenges is not just about avoiding mistakes, but also about learning from them. Each challenge you face is an opportunity to grow and improve your skills as a developer. Stay persistent, keep practicing, and don't let setbacks discourage you from achieving your goals in the world of competitive programming and technical interviews.
Conclusion: Embracing the Power of Coding Challenges
As we wrap up this comprehensive guide on coding challenges, it's crucial to reflect on the key points we've covered and consider the profound impact these challenges can have on a developer's career. Let's recap the essential takeaways and explore why embracing coding challenges is not just beneficial, but necessary for success in the ever-evolving tech landscape.
Key Points Recap
- Diverse Learning Opportunities: Coding challenges offer a wide range of problems that cover various algorithms, data structures, and programming concepts, providing a comprehensive learning experience.
- Skill Enhancement: Regular participation in coding challenges sharpens problem-solving skills, improves code efficiency, and enhances overall programming abilities.
- Interview Preparation: Many tech companies use coding challenges as part of their interview process, making these challenges an excellent way to prepare for job opportunities.
- Community Engagement: Platforms hosting coding challenges often have vibrant communities where developers can learn from each other, share solutions, and grow together.
- Continuous Learning: The tech industry is constantly evolving, and coding challenges help developers stay updated with the latest trends and best practices in programming.
- Career Advancement: Excelling in coding challenges can open doors to new career opportunities and help developers stand out in a competitive job market.
- Personal Growth: Overcoming difficult coding problems builds confidence, resilience, and a growth mindset - qualities that are invaluable in any career.
Encouragement for Your Coding Challenge Journey
Whether you're just starting out or you're a seasoned developer, the world of coding challenges has something to offer you. Here's why you should embrace this journey:
- For Beginners: Don't be intimidated! Start with easier challenges and gradually work your way up. Remember, every expert was once a beginner.
- For Intermediate Developers: Use coding challenges to identify and strengthen your weak areas. Push yourself to tackle more complex problems and explore new algorithms.
- For Advanced Programmers: Challenge yourself with competitive programming contests, contribute to open-source projects, or even create your own coding challenges to give back to the community.
Interactive Motivation Tracker
Track your coding challenge progress:
The Importance of Coding Challenges in a Developer's Career
In the dynamic world of software development, coding challenges play a pivotal role in shaping successful careers. Here's why they are indispensable:
- Skill Validation: Coding challenges provide tangible proof of your abilities, which can be more valuable than certifications in many cases.
- Adaptability: Regular participation in challenges keeps you adaptable to new problems and technologies, a crucial trait in the fast-paced tech industry.
- Networking: Many coding challenge platforms and competitions offer networking opportunities with other developers and potential employers.
- Problem-Solving Mindset: The analytical thinking developed through coding challenges is applicable beyond just coding, benefiting overall career growth.
- Continuous Improvement: The iterative nature of coding challenges fosters a habit of continuous learning and improvement, essential for long-term career success.
Skill | Importance (%) |
---|---|
Problem-solving skills | 73.8% |
Programming languages | 65.2% |
Debugging | 62.7% |
In conclusion, coding challenges are not just a trend or a pastime - they are a fundamental tool for growth, learning, and career advancement in the tech industry. By embracing these challenges, you're not only improving your coding skills but also preparing yourself for the countless opportunities and challenges that lie ahead in your career.
Remember, every line of code you write in these challenges is an investment in your future. So, whether you're solving your first easy problem or competing in international coding competitions, know that you're on the right path. Keep coding, keep learning, and keep challenging yourself - your future self will thank you for it.
As the famous computer scientist and author Ellen Ullman once said:
We build our computer systems the way we build our cities: over time, without a plan, on top of ruins.
Ellen Ullman
Let coding challenges be your blueprint for building a strong, adaptable, and successful career in software development. The journey of a thousand miles begins with a single step - or in this case, a single line of code. Start your coding challenge journey today, and watch as it transforms your skills, your mindset, and ultimately, your career.
Additional Resources
As you continue your journey in mastering coding challenges, it's crucial to have access to high-quality resources that can supplement your learning and practice. This section provides a curated list of recommended books, online courses, and community forums that can help you enhance your skills and stay connected with fellow coding challenge enthusiasts.
Recommended Books on Algorithms and Data Structures
A solid understanding of algorithms and data structures is fundamental to excelling in coding challenges. Here are some highly recommended books that cover these topics in depth:
- "Introduction to Algorithms" by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein
- Often referred to as CLRS, this comprehensive tome is considered the bible of algorithms.
- Check it out on Amazon
- "Cracking the Coding Interview" by Gayle Laakmann McDowell
- A must-read for anyone preparing for technical interviews, with a focus on coding challenges.
- Find it on Amazon
- "Grokking Algorithms" by Aditya Bhargava
- An illustrated guide that makes complex algorithms accessible to beginners.
- Available on Manning Publications
- "The Algorithm Design Manual" by Steven S. Skiena
- Offers a practical approach to algorithm design with real-world examples.
- Purchase on Springer
- "Data Structures and Algorithms in Python" by Michael T. Goodrich, Roberto Tamassia, and Michael H. Goldwasser
- An excellent resource for those using Python for coding challenges.
- Find it on Wiley
Interactive Book Ratings
Online Courses for Improving Coding Skills
Online courses offer structured learning paths and hands-on practice to improve your coding skills. Here are some top-rated options:
- Algorithms Specialization by Stanford University on Coursera
- A comprehensive series of courses covering fundamental algorithms and data structures.
- Data Structures and Algorithms Nanodegree by Udacity
- Offers project-based learning to reinforce your understanding of key concepts.
- Master the Coding Interview: Data Structures + Algorithms on Udemy
- Focuses specifically on preparing for coding interviews with practice problems.
- AlgoExpert
- A platform dedicated to algorithm training with video explanations and coding workspace.
Course Name | Platform | Focus Area | Duration |
Algorithms Specialization | Coursera | Fundamental Algorithms | 4 months |
Data Structures and Algorithms Nanodegree | Udacity | Project-based Learning | 4 months |
Master the Coding Interview | Udemy | Interview Preparation | Self-paced |
Competitive Programmer's Core Skills | Coursera | Competitive Programming | 5 weeks |
AlgoExpert | AlgoExpert.io | Algorithm Training | Self-paced |
Communities and Forums for Coding Challenge Enthusiasts
Engaging with a community of like-minded individuals can significantly enhance your learning experience and provide valuable support. Here are some popular forums and communities for coding challenge enthusiasts:
- LeetCode Discuss
- A vibrant community where users share solutions, discuss problem-solving approaches, and offer interview insights.
- Stack Overflow
- While not specifically for coding challenges, it's an invaluable resource for any programming-related questions.
- r/dailyprogrammer
- A subreddit that posts coding challenges regularly, allowing users to share and discuss solutions.
- CodeForces
- Hosts regular coding contests and has an active forum for discussions on algorithms and problem-solving strategies.
- HackerRank Community
- Offers discussion forums, blogs, and a community where developers can connect and share knowledge.
- TopCoder Forums
- A platform for competitive programmers with active discussion forums and regular coding competitions.
Community Activity Heatmap
This heatmap represents the activity levels in coding challenge communities throughout the week. Darker colors indicate higher activity levels, helping you identify the best times to engage with the community for quick responses and lively discussions.
By leveraging these additional resources - books, online courses, and community forums - you can significantly enhance your coding skills and stay at the forefront of the ever-evolving world of coding challenges. Remember, consistent practice and engagement with these resources are key to mastering the art of solving coding challenges efficiently.
Frequently Asked Questions about Coding Challenges
What are the best coding challenge websites?
Some of the most popular and highly-regarded coding challenge websites include LeetCode, HackerRank, Codewars, Project Euler, and TopCoder. Each platform offers a unique set of challenges and features, catering to different skill levels and programming languages.
How do coding challenges help improve programming skills?
Coding challenges help improve programming skills by exposing you to a variety of problems, encouraging you to think critically, and pushing you to optimize your code. They also help you learn new algorithms, data structures, and coding patterns that you can apply in real-world projects.
What are the top coding challenges for beginners?
Beginners should start with basic algorithmic problems such as array manipulation, string operations, and simple math problems. Platforms like HackerRank and LeetCode offer curated tracks for beginners, gradually increasing in difficulty as you progress.
How can coding challenges prepare you for technical interviews?
Coding challenges simulate the problem-solving environment of technical interviews. They help you practice thinking out loud, explaining your approach, and coding under time pressure - all crucial skills for technical interviews. Many companies use similar problems in their interview process.
What are the most common coding challenges and solutions?
Common coding challenges often involve data structures (like arrays, linked lists, trees), algorithms (sorting, searching), and problem-solving patterns (two pointers, sliding window, dynamic programming). Solutions typically focus on optimizing for time and space complexity.
How can I improve my coding skills quickly through challenges?
To improve quickly, practice consistently, start with easier problems and gradually increase difficulty, study solutions after attempting problems, and focus on understanding underlying concepts rather than memorizing solutions. Participating in coding contests can also accelerate your learning.
Are coding challenges necessary for getting hired as a software developer?
While not always mandatory, coding challenges are increasingly common in the hiring process for software developers. They help employers assess problem-solving skills, coding ability, and thought processes. Even if not required, practicing challenges can significantly improve your chances of success in technical interviews.
Can coding challenges help me learn new programming languages?
Yes, coding challenges are an excellent way to learn new programming languages. They provide practical problems to solve, allowing you to apply language syntax and features in context. Many platforms support multiple languages, enabling you to solve the same problem in different languages for comparison.
What types of coding challenges should a junior programmer focus on?
Junior programmers should focus on fundamental data structures (arrays, strings, linked lists), basic algorithms (sorting, searching), and problem-solving techniques. Start with easy to medium difficulty problems on platforms like LeetCode or HackerRank, and gradually progress to more complex challenges as you build confidence.
How can I balance practicing coding challenges with my regular work or studies?
Set a consistent schedule, such as dedicating 30-60 minutes daily to coding challenges. Integrate challenge practice into your learning or work routine, perhaps during breaks or as a warm-up exercise. Focus on quality over quantity, and use weekends for longer practice sessions if needed. Remember to maintain a healthy balance to avoid burnout.