Fix ENOENT Error: Guide for All Platforms and Tools
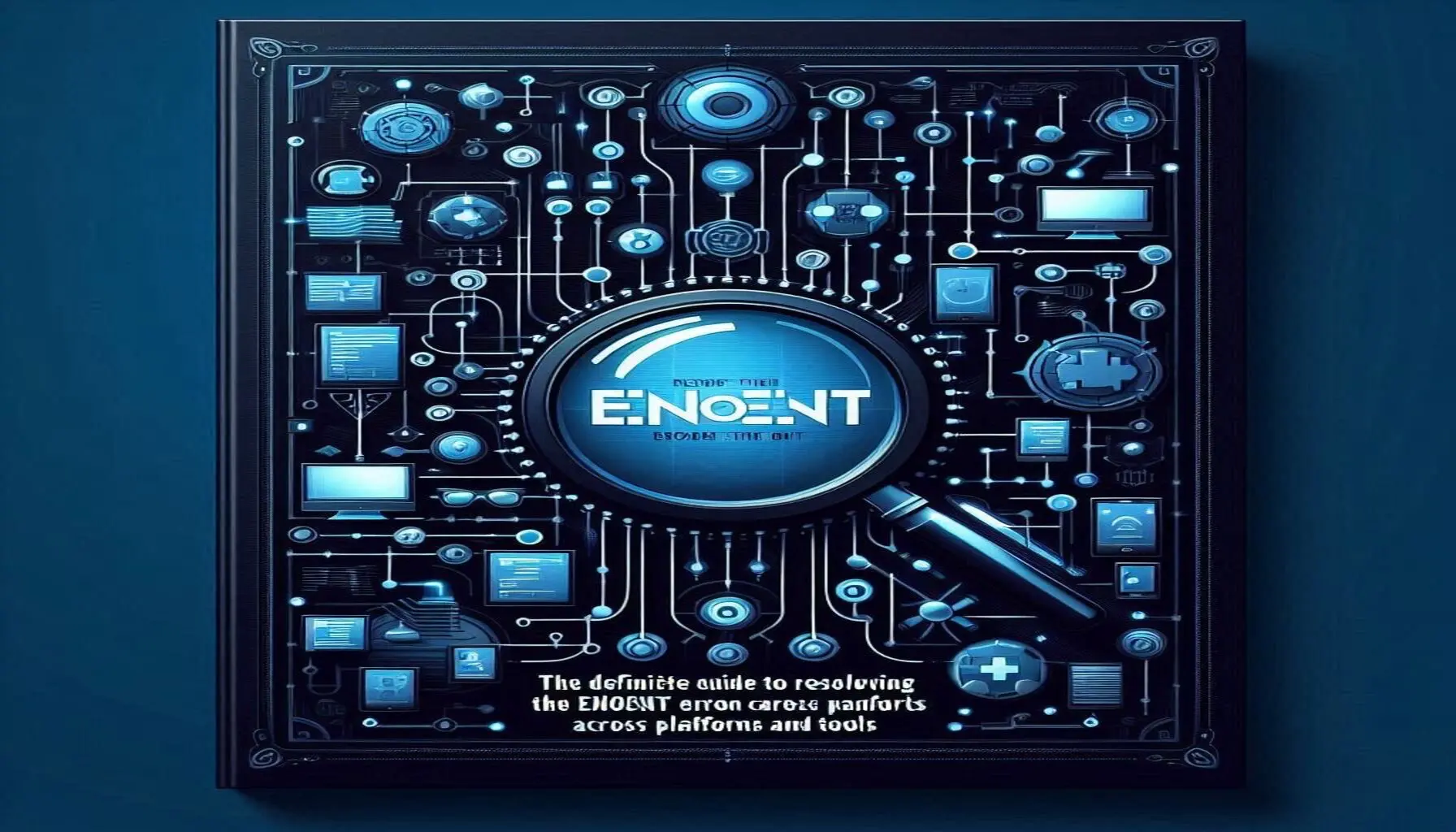
The ENOENT error, which stands for “Error No Entry” or “Error No Entity,” is a common issue that developers encounter across various programming languages, tools, and platforms. This error typically occurs when a program attempts to access a file or directory that does not exist or when there is a problem with file paths or permissions.
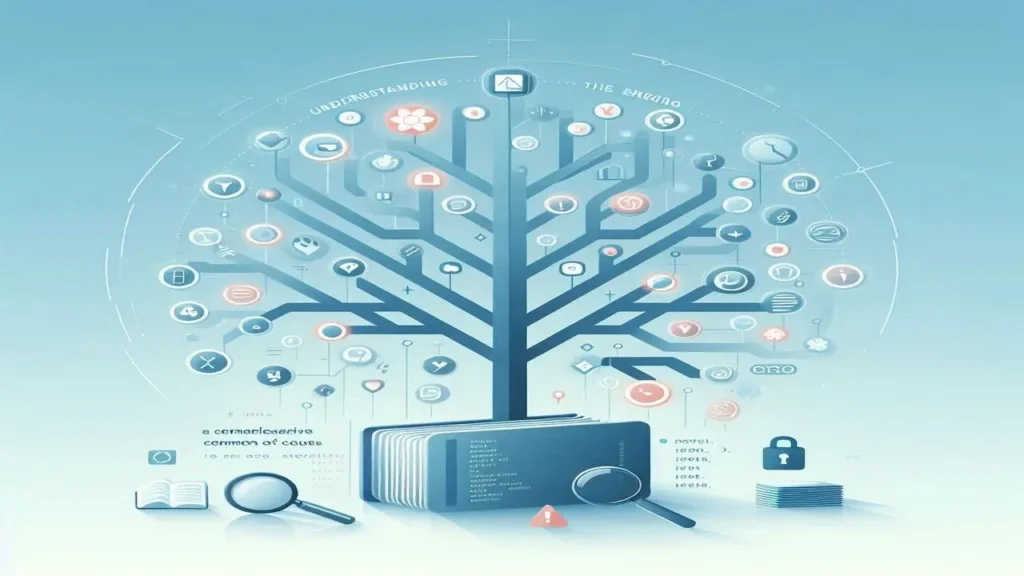
The ENOENT error can be frustrating and time-consuming to resolve, especially for those who are new to programming or unfamiliar with the specific environment or tool they are working with. However, understanding the root causes of this error and knowing how to troubleshoot it effectively can save developers a significant amount of time and effort.
In this comprehensive guide, we will dive deep into the ENOENT error, exploring its meaning, common causes, and practical solutions for resolving it across various programming contexts. We will also cover best practices for preventing ENOENT errors and provide valuable insights from the developer community on Stack Overflow.
What is the ENOENT Error?
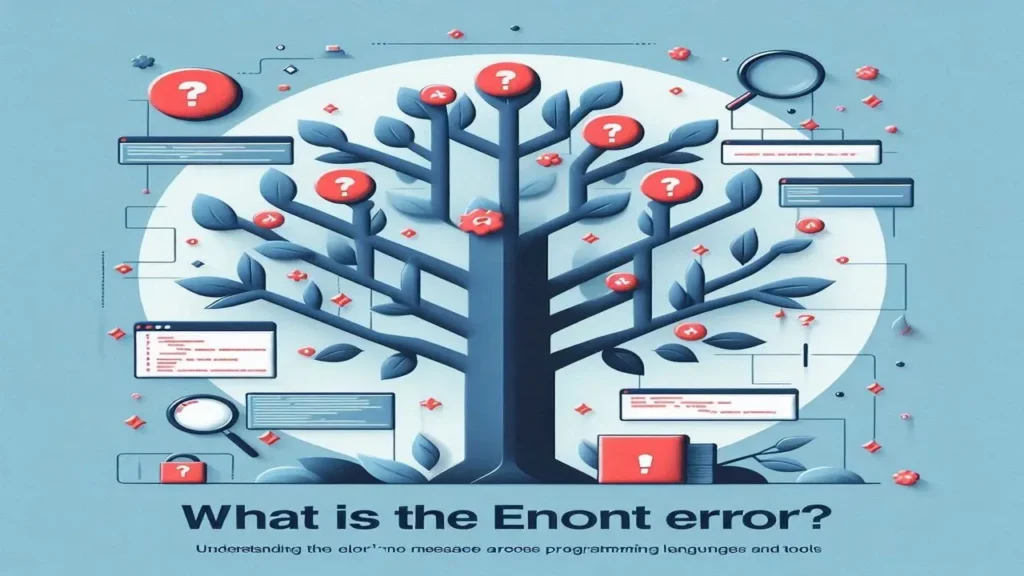
The ENOENT error is a common error message that indicates a file or directory does not exist or cannot be accessed. This error can occur in various programming languages and tools, including Node.js, React, Java, Ruby, and more. The abbreviation “ENOENT” stands for “Error NO ENTry” or “Error NO ENTity,” and it has its origins in the early days of C programming when compilers had limitations on the length of symbols.
Here’s an example of an ENOENT error message you might encounter in Node.js:
Error: ENOENT: no such file or directory, open 'file.txt'
In this example, the error message indicates that the program is unable to open the file file.txt because it does not exist in the specified location or path.
It’s important to note that while the ENOENT error is commonly associated with file and directory operations, it can also occur in other contexts, such as when attempting to bind an algorithm that doesn’t exist in certain sockets or when a command is not found in Node.js child processes.
Common Causes of the ENOENT Error
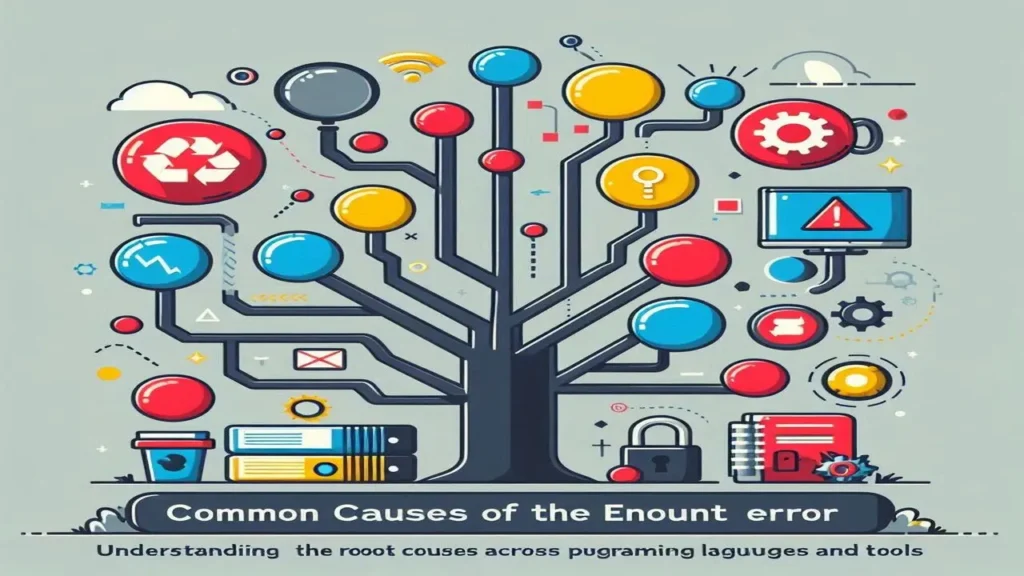
The ENOENT error can be caused by various factors, including:
Incorrect file paths
One of the most common causes of the ENOENT error is specifying an incorrect file path. This can happen when there are typos or inconsistencies in the file path, or when the path is relative and the program is being executed from a different directory than expected.
Deleted or missing files/directories
If a file or directory that a program is trying to access has been deleted or moved, the ENOENT error will occur.
Permission issues
In some cases, the ENOENT error can occur due to insufficient permissions to access a file or directory. This is particularly common in scenarios where a program is running with limited privileges or in a restricted environment.
Environmental factors
Issues with dependencies, configurations, or system resources (such as low RAM) can also contribute to the occurrence of ENOENT errors, especially during build processes or when working with complex toolchains.
It’s worth noting that the specific cause of the ENOENT error can vary depending on the programming language, tool, or platform being used, as well as the context in which the error occurs.
Related Articles:
Troubleshooting the ‘npm err code enoent’
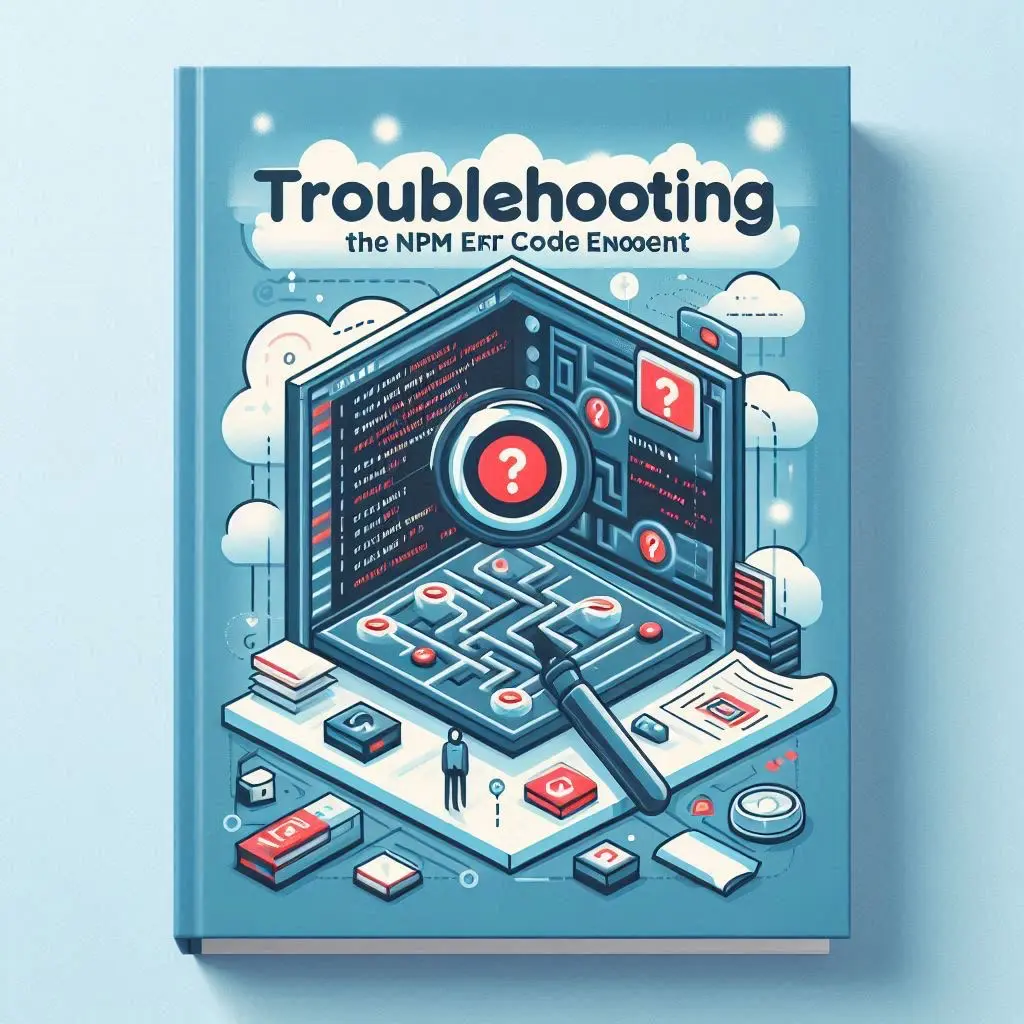
One of the most common contexts where developers encounter the ENOENT error is when working with Node.js and the npm package manager. The ‘npm err code enoent’ error typically occurs when npm is unable to find or access a file or directory specified in the package.json file or when there is an issue with file paths during the installation or execution of Node.js packages.
Here are some common scenarios that can lead to the ‘npm err code enoent’ error:
- Missing package.json file: If the package.json file is missing from the project directory, npm will not be able to find the necessary information to install or manage dependencies, resulting in the ENOENT error.
- Incorrect file paths: If the file paths specified in the package.json file for dependencies, scripts, or other resources are incorrect or outdated, npm may not be able to locate the required files, leading to the ENOENT error.
- Deleted or moved files: If files referenced in the package.json file have been deleted or moved without updating the corresponding paths, npm will encounter the ENOENT error when attempting to access those files.
- Permission issues: In some cases, the ENOENT error can occur due to insufficient permissions to access files or directories required by npm or the packages being installed.
To troubleshoot the ‘npm err code enoent’ error, you can try the following steps:
- Quick fixes:
- Verify the paths specified in the package.json file and ensure they are correct.
- If the package.json file is missing, run npm init to create a new one.
- Advanced strategies:
- Audit your project’s dependencies using npm audit to identify and resolve any issues with outdated or insecure packages.
- Use npm ci instead of npm install to ensure a consistent and reproducible installation of dependencies based on the package-lock.json file.
- Check for any missing dependencies or scripts in the package.json file and update them as needed.
- Docker environments:
- In Docker environments, ensure that the working directory is correctly set up and that all necessary files, including the package.json and any referenced files, are included in the Docker image or mounted volume.
- Continuous Integration/Continuous Deployment (CI/CD) pipelines:
- If you are encountering ENOENT errors in CI/CD pipelines, verify that the correct files are being copied or downloaded during the build or deployment process.
- Check for any caching or dependency installation issues that may be causing the ENOENT error in the pipeline.
To further expand your knowledge and mastery of npm, consider consulting the official npm Documentation, the Node.js Guides, and the The npm Blog for comprehensive coverage of npm best practices, advanced features, and troubleshooting techniques.
In addition to the ‘npm err code enoent’ error, the ENOENT error can occur in various other programming contexts, which we will explore in the following sections.
ENOENT Errors in Different Programming Contexts
While the ENOENT error is commonly encountered in Node.js and npm environments, it can also occur in various other programming contexts and languages. Understanding the specific scenarios where this error arises and how to troubleshoot it can be invaluable for developers working across different platforms and tools.
Troubleshooting ENOENT errors in Development
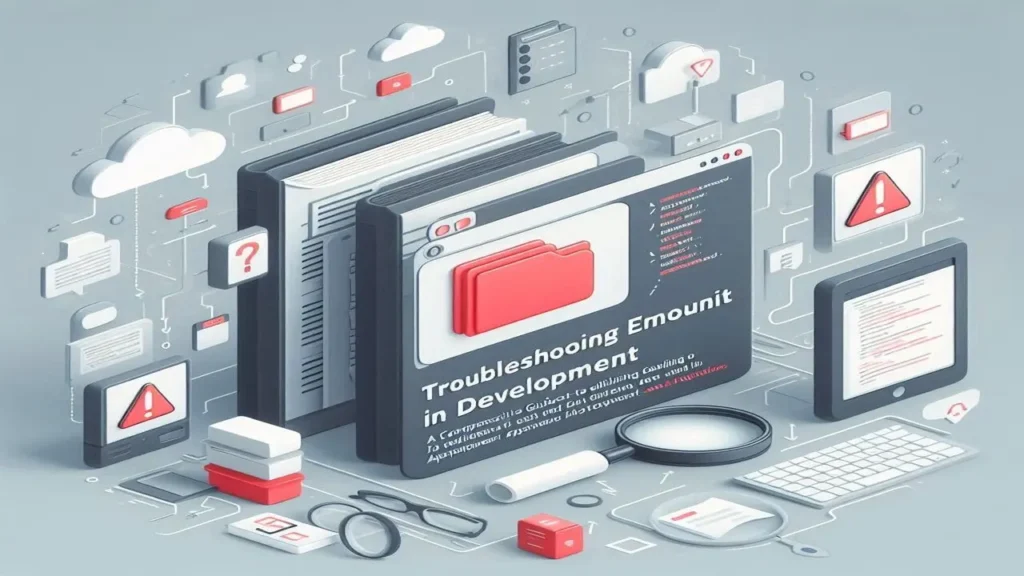
During the development phase, ENOENT errors can occur when working with file operations, imports, or require statements. Here are some frequent circumstances with troubleshooting steps:
- Front-end applications (React, Next.js, etc.):
- Verify that the file paths specified in import statements or require calls are correct.
- Check if the required files or modules exist in the expected locations.
- Ensure that any necessary build steps or bundling processes have completed successfully.
Example React error:
Failed to compile ./src/components/MyComponent.js
Module not found: You attempted to import ... which falls outside of the project src/ directory
- Java applications:
- Check if the file paths specified in the Java code (e.g., FileInputStream, FileReader) are correct and that the files exist.
- Verify that the application has the necessary permissions to access the files or directories.
Example Java error:
java.io.FileNotFoundException: file.txt (No such file or directory)
- Ruby (creating files, working with gems, etc.):
- Ensure that the file paths specified in Ruby code (e.g., File.open, require) are correct and that the files exist.
- Check if the application has the necessary permissions to create, read, or write files in the specified directories.
Example Ruby error:
Errno::ENOENT: No such file or directory @ rb_sysopen - file.txt
Here’s an example of how you might handle an ENOENT error when reading a file in Node.js:
const fs = require('fs');
try {
const data = fs.readFileSync('file.txt', 'utf8');
console.log(data);
} catch (err) {
if (err.code === 'ENOENT') {
console.error('File not found!');
} else {
console.error('Error occurred while reading file:', err);
}
}
In this example, we use a try…catch block to handle any errors that may occur when reading the file file.txt. If the error is specifically an ENOENT error (indicated by err.code === ‘ENOENT’), we log a message indicating that the file was not found. Otherwise, we log a generic error message.
By properly handling ENOENT errors in your code, you can provide clear feedback to users or developers and implement appropriate fallback or recovery mechanisms.
Troubleshooting ENOENT error in Production
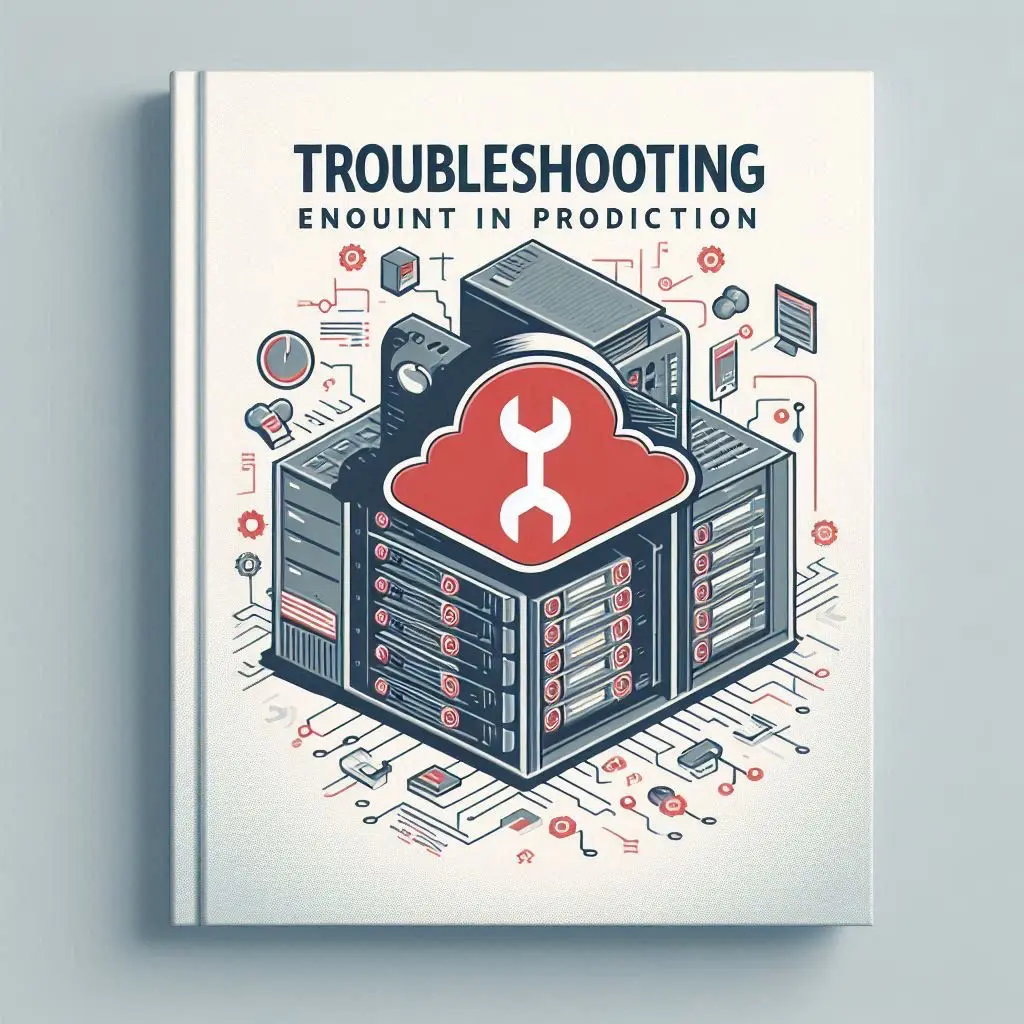
While ENOENT errors are commonly encountered during development, they can also manifest in production environments, particularly during build processes or when dealing with file operations at runtime. Here are some common scenarios and troubleshooting steps for handling ENOENT errors in production:
- Build output paths:
- Verify that the build output paths specified in your project’s configuration files (e.g., next.config.js for Next.js, webpack.config.js for Webpack) are correct and that the output directories exist.
- Check if the build process has the necessary permissions to write files to the specified output directories.
Example Next.js error:
Error: ENOENT: no such file or directory, open 'E:\projects\almostwiki\my-app\.next\BUILD_ID'
- Missing files or directories:
- Ensure that all required files and directories are present in the production environment or deployment package.
- Check if any files or directories have been accidentally deleted or moved during the deployment process.
- Environmental factors:
- Verify that the production environment has sufficient resources (e.g., RAM, disk space) to handle the build or file operations.
- Check if any dependencies or configurations need to be updated or adjusted for the production environment.
Here’s an example of how you might handle an ENOENT error when creating a file in Node.js:
const fs = require('fs');
const path = require('path');
const filePath = path.join(__dirname, 'output', 'file.txt');
try {
fs.writeFileSync(filePath, 'Hello, World!');
console.log('File created successfully.');
} catch (err) {
if (err.code === 'ENOENT') {
console.error('Error: Directory not found. Creating directory...');
fs.mkdirSync(path.dirname(filePath), { recursive: true });
fs.writeFileSync(filePath, 'Hello, World!');
console.log('File created successfully.');
} else {
console.error('Error occurred while creating file:', err);
}
}
In this example, we attempt to create a file named file.txt in the output directory. If the output directory does not exist, we will encounter an ENOENT error. To handle this, we check if the error code is ‘ENOENT’, and if so, we create the missing directory using fs.mkdirSync with the recursive option set to true to create any necessary parent directories. After creating the directory, we retry writing the file.
By implementing proper error handling and recovery mechanisms, you can ensure that your application can gracefully handle ENOENT errors and continue functioning as expected in production environments.
Solutions from Stack Overflow
The Stack Overflow community is a valuable resource for developers seeking solutions to various programming challenges, including ENOENT errors. In this section, we’ll explore some key questions and solutions from Stack Overflow related to ENOENT errors across different programming contexts.
ENOENT error in Node.js
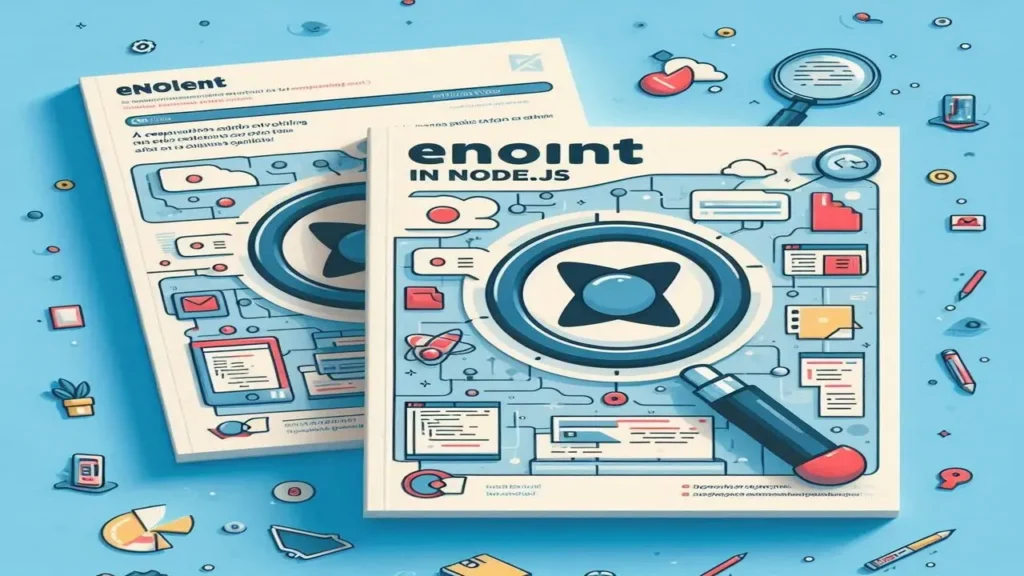
One of the most common contexts where developers encounter ENOENT errors is when working with Node.js and its built-in modules, such as the file system (fs) module. Here are some examples of Stack Overflow questions and solutions related to ENOENT errors in Node.js:
Node.js require() error: ENOENT: no such file or directory
This question deals with a common issue where the require() function in Node.js throws an ENOENT error when attempting to load a module from a file that does not exist or has an incorrect file path.
Solution:
- Verify the file path specified in the require() statement is correct and that the file exists.
- Check if the file is located in the correct directory relative to the current working directory.
- Ensure that the file extension is correct (e.g., .js for JavaScript files).
Error: ENOENT: no such file or directory, open ‘node:timers/promises’
This question revolves around an ENOENT error occurring when using the middy middleware library in a Node.js application.
Solution:
- Update the middy library to the latest version, as the error was related to a breaking change in a specific version of the library.
- Ensure that the Node.js version being used is compatible with the middy library version.
Error: ENOENT: no such file or directory, open ‘E:\projects\almostwiki\my-app\test\my-app.next\BUILD_ID’
This question deals with an ENOENT error encountered during the build process of a Next.js application.
Potential Solutions:
- Increase the available RAM on the system, as Next.js may require more memory for larger projects.
- Update dependencies and build tools (e.g., SWC) to the latest versions.
- Adjust Next.js configuration settings, such as the distDir option, to specify a different output directory for the build.
ENOENT error in React/Front-end
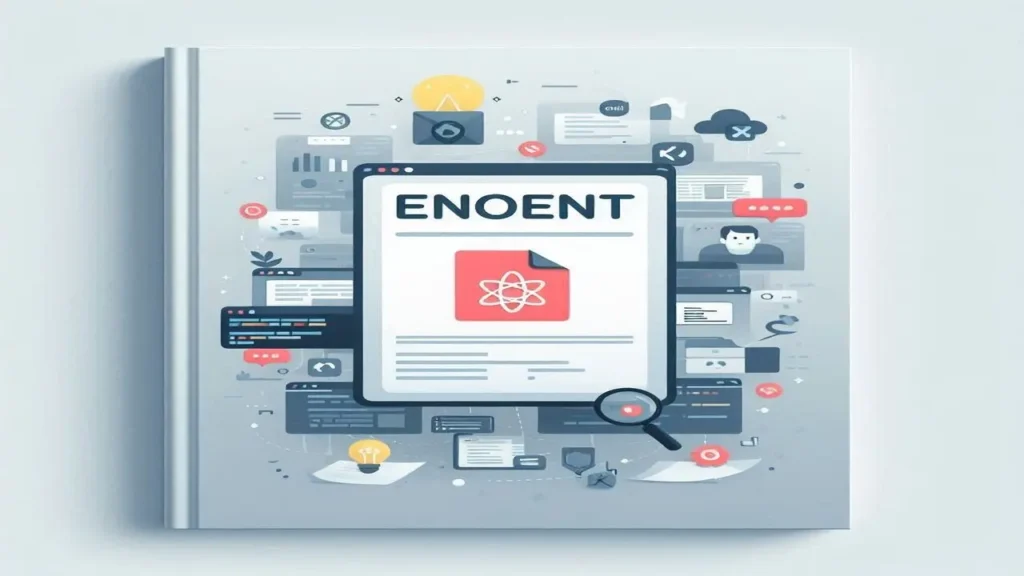
ENOENT errors can also occur in front-end development, particularly when working with popular frameworks and libraries like React and Next.js. Here are some examples of Stack Overflow questions and solutions related to ENOENT errors in front-end development:
Error in Next.js, shadcn-ui on running npm run dev -> Error: ENOENT: no such file or directory, stat ‘C:/components.json’
This question deals with an ENOENT error that occurs when trying to run a Next.js application that uses the shadcn-uicomponent library.
Solution:
- The error is likely due to a misconfiguration or outdated version of the shadcn-ui library.
- Update the shadcn-ui library to the latest version or follow the installation instructions provided by the library maintainers.
tanstack react-query ENOENT error when uninstalling v5 and installing v4
This question discusses an ENOENT error that occurs when attempting to uninstall and install different versions of the react-query library in a React application.
Solution:
- Clear the npm cache using npm cache clean –force and try reinstalling the desired version of the react-querylibrary.
- If the issue persists, try deleting the node_modules folder and the package-lock.json file, and then reinstall all dependencies.
Error: spawn npm ENOENT” in Creating An Expo CLI app
This question relates to an ENOENT error that occurs when trying to create a new Expo CLI app for React Native development.
Solution:
- The error may be caused by a conflicting installation of Node.js or npm on the system.
- Uninstall any existing versions of Node.js and npm, and then reinstall the latest versions from the official website.
- Alternatively, try using a Node.js version manager like nvm or fnm to ensure a clean installation of the desired Node.js version.
ENOENT error in Java
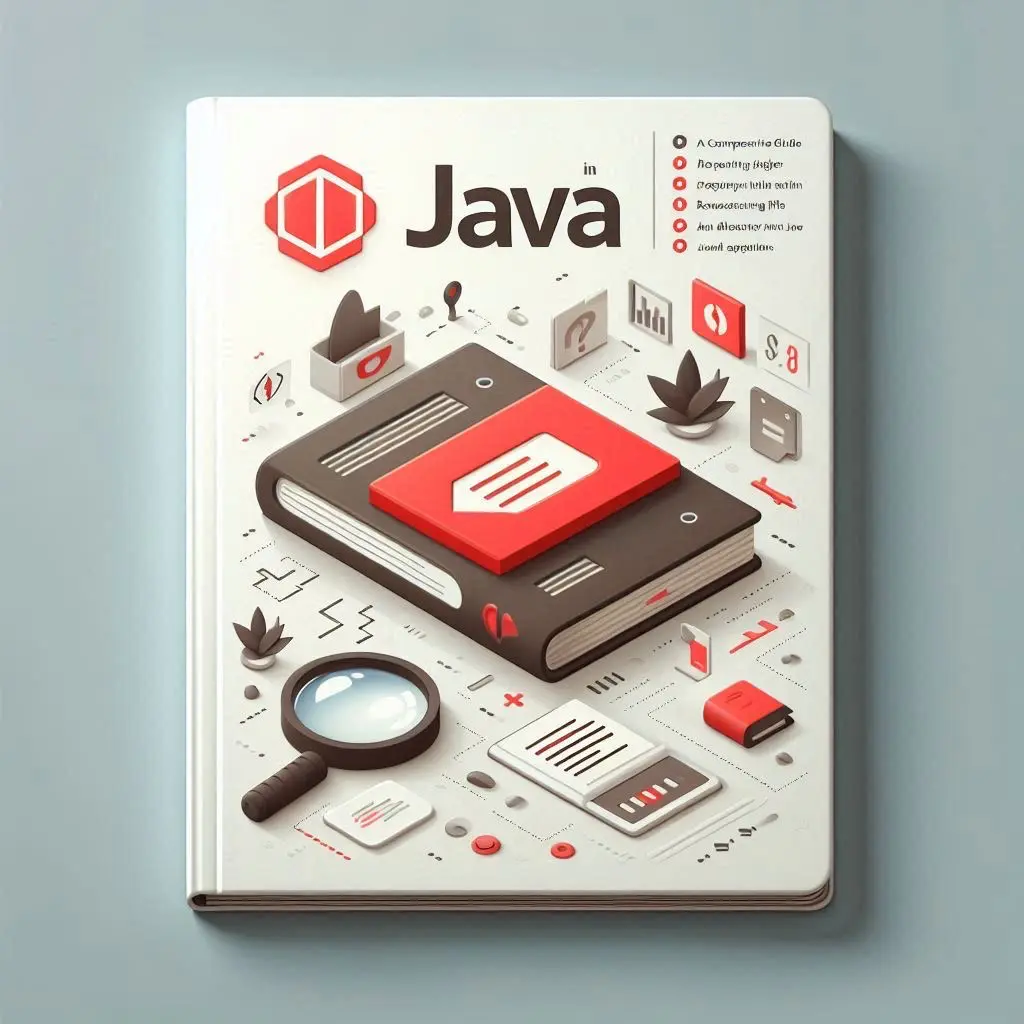
While the ENOENT error is commonly associated with JavaScript and Node.js environments, it can also occur in other programming languages, such as Java. Here are some examples of Stack Overflow questions and solutions related to ENOENT errors in Java:
Java don’t see the file
This question deals with a situation where a Java program is unable to access a file, resulting in an ENOENT error.
Solution:
- Ensure that the file path specified in the Java code is correct and that the file exists in the specified location.
- Check if the Java program has the necessary permissions to access the file or directory.
- Verify that the file is not being used or locked by another process or application.
ENOENT Error when trying to create Excel file in Ruby
While this question is related to Ruby, it highlights a common issue where an ENOENT error can occur when trying to create or write to a file.
Solution:
- Check if the directory where the file is being created exists and if the program has the necessary permissions to write to that directory.
- Ensure that the file path and name are correctly specified in the code.
- Consider using the Dir.mkdir method in Ruby to create the directory if it doesn’t exist before attempting to create the file.
Parcel ENOENT: no such file or directory
This question revolves around an ENOENT error that occurs when using the Parcel bundler, which is a web application bundler similar to Webpack.
Solution:
- Verify that the file paths specified in the Parcel configuration or entry point are correct and that the files exist.
- Check if the Parcel version being used is compatible with the project’s dependencies and configuration.
- Ensure that the correct command is being used to run Parcel (e.g., parcel index.html or parcel build).
ENOENT error in Ruby
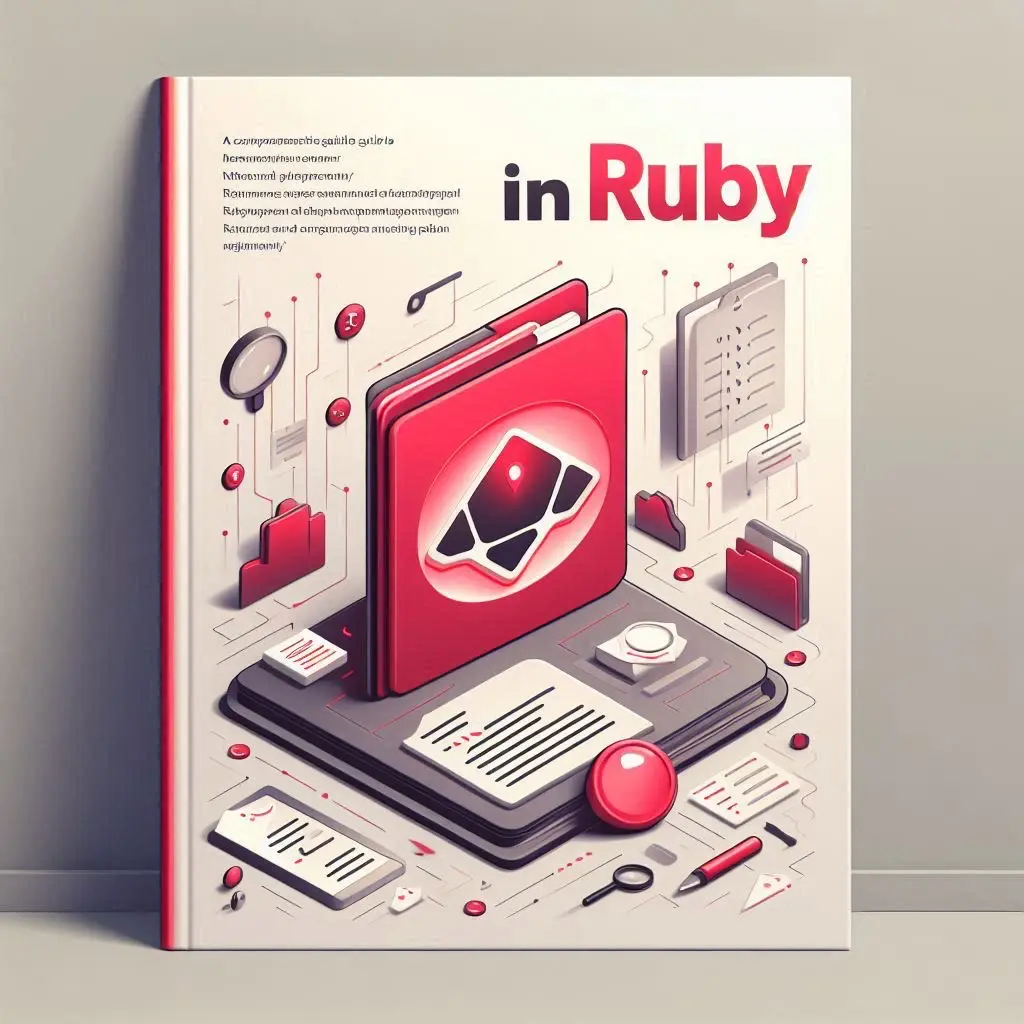
Ruby is another programming language where developers may encounter ENOENT errors, particularly when working with file operations or managing dependencies through tools like RubyGems. Here are some examples of Stack Overflow questions and solutions related to ENOENT errors in Ruby:
Errno::ENOENT: No such file or directory @ rb_sysopen – file.txt
This question deals with a common ENOENT error that occurs when attempting to open a file that does not exist in Ruby.
Solution:
- Verify that the file path specified in the Ruby code is correct and that the file exists in the specified location.
- Check if the Ruby program has the necessary permissions to access the file or directory.
- Consider using the File.exist? method to check if the file exists before attempting to open it.
ENOENT Error when trying to create Excel file in Ruby
This question deals with an ENOENT error that occurs when trying to create an Excel file using the write_xlsx gem in Ruby.
Solution:
- Ensure that the directory where the file is being created exists and that the Ruby program has the necessary permissions to write to that directory.
- Double-check that the file path and name are correctly specified in the code.
- Consider using the Dir.mkdir method in Ruby to create the directory if it doesn’t exist before attempting to create the file.
ENOENT: no such file or directory, Cannot load such file — bundler/setup
This question is related to an ENOENT error that occurs when trying to load the bundler gem in a Ruby on Rails project.
Solution:
- Verify that the bundler gem is installed correctly and that the project’s Gemfile is properly configured.
- Try running bundle install to install or update the project’s dependencies.
- If the issue persists, consider removing the Gemfile.lock file and running bundle install again to regenerate the lock file.
ENOENT error in Other Programming Contexts
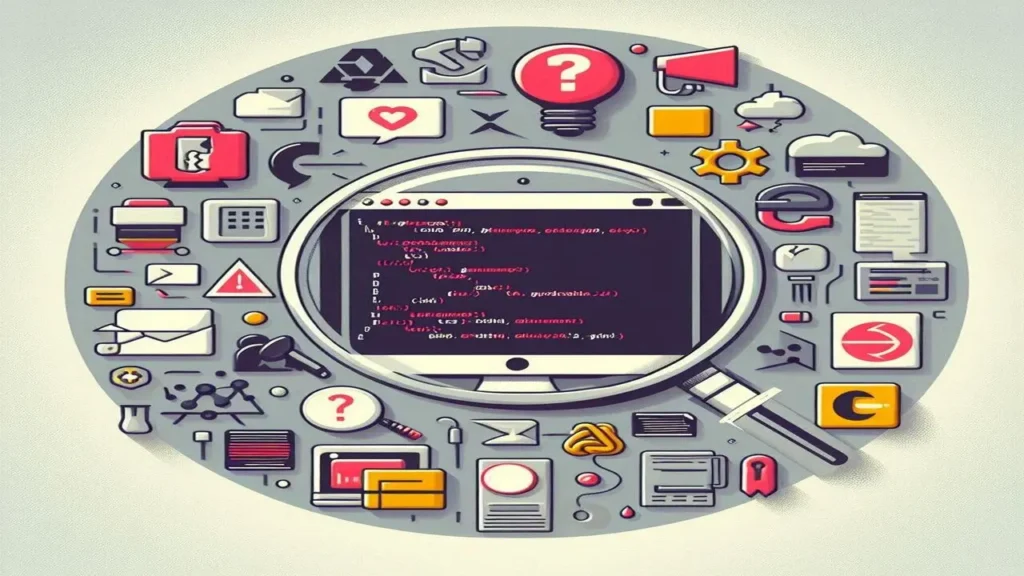
While we’ve covered some of the most common programming contexts where ENOENT errors can occur, such as Node.js, front-end development, Java, and Ruby, the ENOENT error is not limited to these environments. Developers may encounter this error in various other programming languages, tools, and platforms.
Here are some additional examples of Stack Overflow questions and solutions related to ENOENT errors in different programming contexts:
npm ERR! syscall open npm ERR! enoent Could not read package.json: Error: ENOENT: no such file or directory, open ‘/client/package.json’
This question deals with an ENOENT error that occurs when npm is unable to read the package.json file in a specific directory.
Solution:
- Ensure that the package.json file exists in the specified directory (/client in this case).
- Check if the npm command is being executed from the correct directory or if the directory path is correctly specified.
- Consider creating a new package.json file using npm init if the file is missing or corrupted.
idealTree:example.js: sill idealTree buildDeps
This question is related to an ENOENT error that occurs when running the idealTree command in a TypeScript project.
Solution:
- The error may be caused by a missing or outdated dependency required by the idealTree command.
- Try reinstalling or updating the project’s dependencies using the appropriate package manager (e.g., npm installor yarn install).
- If the issue persists, consider reporting it to the maintainers of the idealTree project or seeking further assistance from their support channels.
Cypress Azure “Can’t walk dependency graph: ENOENT: no such file or directory, lstat” error
This question is related to an ENOENT error that occurs when running Cypress tests on an Azure pipeline.
Solution:
- The error may be caused by missing or incorrect file paths in the Cypress configuration or the Azure pipeline setup.
- Verify that the file paths specified in the Cypress configuration and Azure pipeline are correct and that the necessary files exist.
- Check if the Azure pipeline has the required permissions to access the project files and dependencies.
These examples highlight that the ENOENT error can occur in various programming contexts beyond the ones we’ve covered in detail. Developers should be prepared to troubleshoot and handle ENOENT errors regardless of the programming language, tool, or platform they are working with, as proper error handling is crucial for building reliable and robust applications.
Mastering npm for ENOENT Prevention
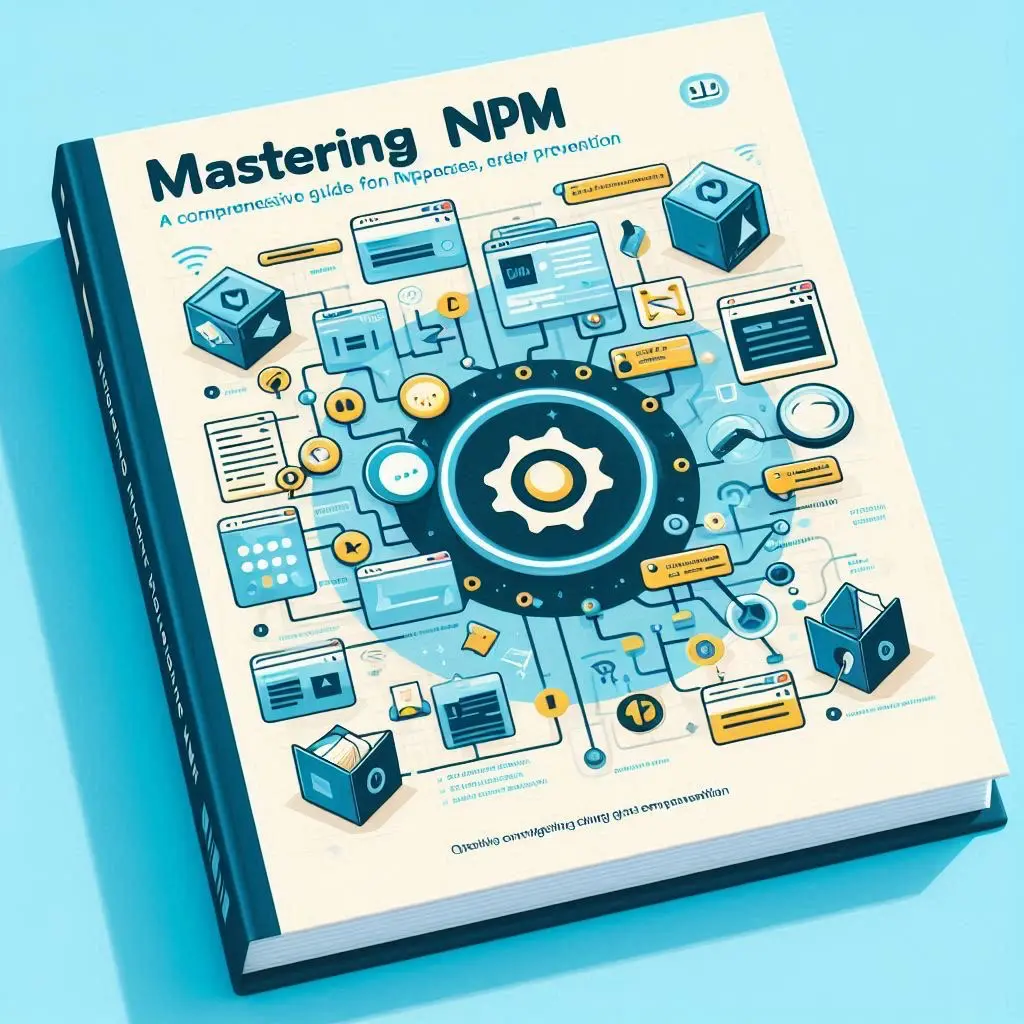
One of the most common contexts where developers encounter ENOENT errors is when working with Node.js and the npm package manager. As npm is a crucial component of the Node.js ecosystem, mastering its usage and best practices can significantly reduce the chances of encountering ENOENT errors and streamline your development workflow.
Utilizing npm Documentation
The official npm Documentation is a comprehensive resource that covers all aspects of npm, from installation and configuration to package management and troubleshooting.
Familiarize yourself with the documentation’s sections on file paths, dependency management, and troubleshooting to gain a deeper understanding of how npm handles file operations and dependencies, which can help prevent ENOENT errors.
Regularly check the documentation for updates and new features, as npm is constantly evolving to improve its functionality and user experience.
npm Guides and Best Practices
In addition to the official documentation, various online guides and resources provide valuable insights and best practices for using npm effectively.
The Node.js Guides offer practical advice and examples for managing packages, dependencies, and working with npm scripts.
The The npm Blog is a great source for staying up-to-date with the latest npm developments, tips, and techniques.
Consider following reputable online communities, such as the npm community on GitHub, to learn from experienced developers and engage in discussions around npm best practices.
Continuously Expanding npm Expertise
Mastering npm is an ongoing process, as new features, updates, and best practices are constantly emerging.
Attend workshops, webinars, or conferences focused on Node.js and npm to learn from experts and stay informed about the latest trends and techniques.
Participate in online forums, such as Stack Overflow or the npm community on GitHub, to engage with other developers, ask questions, and share your experiences.
Experiment with different npm features and workflows in personal or side projects to gain hands-on experience and solidify your understanding.
Consider contributing to open-source projects that utilize npm, as this can provide valuable insights into real-world npm usage and best practices.
By continuously expanding your npm expertise and staying up-to-date with the latest developments and best practices, you can significantly reduce the chances of encountering ENOENT errors and other issues related to package management and file operations in your Node.js projects.
Preventing ENOENT Errors
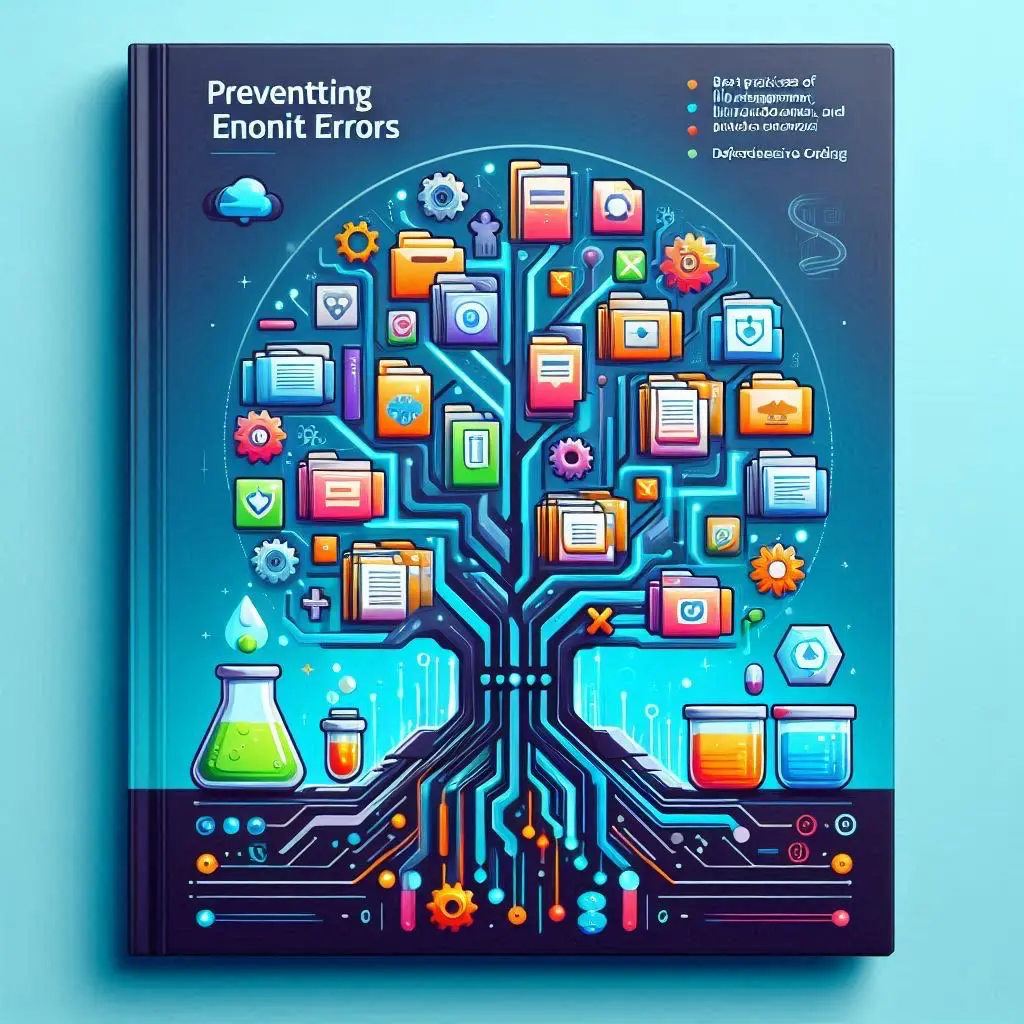
While troubleshooting and resolving ENOENT errors is essential, it’s equally important to adopt best practices and strategies to prevent these errors from occurring in the first place. By implementing proper file management practices, optimizing build and runtime environments, and practicing defensive coding techniques, developers can minimize the chances of encountering ENOENT errors and ensure smoother development and deployment processes.
File Management Best Practices
- Maintain a well-organized project structure with consistent file and directory naming conventions.
- Utilize version control systems like Git to track file changes and avoid accidental deletions or modifications.
- Regularly review and update file paths in your codebase, configuration files, and build scripts to ensure they remain accurate.
- Implement automated testing for file operations to catch potential issues early in the development cycle.
Optimizing Build and Runtime Environments
- Ensure that build tools, package managers, and dependencies are up-to-date and compatible with your project’s requirements.
- Allocate sufficient system resources (e.g., RAM, disk space) for build processes and runtime operations.
- Configure build output paths and deployment settings correctly to avoid issues with missing or incorrect file paths.
- Consider using containerization technologies like Docker to maintain consistent and reproducible build and runtime environments.
Defensive Coding Techniques
- Implement proper error handling and error logging mechanisms in your code to catch and address ENOENT errors proactively.
- Utilize try-catch blocks, check for file existence using functions like fs.existsSync() in Node.js, and gracefully handle missing files or directories.
- Write unit tests to verify the behavior of your code when dealing with file operations and potential ENOENT scenarios.
- Document error handling strategies and edge cases related to ENOENT errors in your codebase for future reference and maintainability.
By adopting these preventive measures and fostering a culture of best practices within your development team, you can significantly reduce the likelihood of encountering ENOENT errors and ensure a smoother and more efficient development and deployment process.
Additionally, staying up-to-date with the latest developments, bug fixes, and discussions in the programming communities you work with can provide valuable insights and proactive measures to prevent or mitigate ENOENT errors before they occur.
Conclusion
The ENOENT error is a common issue that developers encounter across various programming languages, tools, and platforms. While it may seem like a minor annoyance at first, failing to properly understand and address ENOENT errors can lead to significant delays, frustrations, and potential roadblocks in the development and deployment processes.
Throughout this comprehensive guide, we’ve explored the meaning and origins of the ENOENT error, its common causes, and practical solutions for troubleshooting and resolving it in different programming contexts. We’ve also delved into the valuable insights and solutions provided by the developer community on Stack Overflow, showcasing the collaborative effort in overcoming shared challenges.
Preventing ENOENT errors is equally important as resolving them. By adopting best practices such as proper file management, optimizing build and runtime environments, and implementing defensive coding techniques, developers can significantly reduce the likelihood of encountering ENOENT errors and ensure smoother development and deployment processes.
Remember, staying up-to-date with the latest developments, bug fixes, and discussions within the programming communities you work with is crucial. Continuously expanding your knowledge and staying informed about potential issues and preventive measures related to ENOENT errors can help you stay ahead of the curve and maintain a proactive approach to error handling.
Embracing a culture of continuous learning, knowledge sharing, and collaboration within your development team can further enhance your ability to tackle ENOENT errors and other programming challenges effectively. By fostering an environment that encourages open dialogue, problem-solving, and the exchange of best practices, you can collectively build more robust and resilient applications.
As the programming landscape continues to evolve, the ENOENT error may persist, but with the right mindset, tools, and strategies, developers can confidently navigate and overcome this challenge, ensuring the successful delivery of high-quality software solutions.
FAQs
What does ENOENT stand for?
ENOENT stands for “Error NO ENTry” or “Error NO ENTity.” It is an error code that indicates a file or directory that a program is attempting to access does not exist or cannot be found.
What causes ENOENT errors?
ENOENT errors can be caused by various factors, including:
- Incorrect file paths: Specifying an incorrect or non-existent file path in your code, configuration files, or build scripts.
- Deleted or missing files/directories: Attempting to access a file or directory that has been deleted or moved without updating the corresponding file paths.
- Permission issues: Having insufficient permissions to access or create files or directories in a specific location.
- Environmental factors: Issues with dependencies, configurations, or system resources (e.g., low RAM) during build processes or runtime operations.
How to troubleshoot ENOENT errors?
Here are some common troubleshooting steps for ENOENT errors:
- Verify file paths: Double-check that the file paths specified in your code, configuration files, or build scripts are correct and that the files or directories exist in the expected locations.
- Check file existence: Use file system functions or methods (e.g., fs.existsSync() in Node.js, File.exist? in Ruby) to check if the files or directories exist before attempting to access them.
- Check permissions: Ensure that your program or build process has the necessary permissions to access, create, or modify files and directories in the specified locations.
- Update dependencies and configurations: Review and update your project’s dependencies and configurations to ensure they are compatible and up-to-date.
- Optimize build and runtime environments: Allocate sufficient system resources (e.g., RAM, disk space) and configure build output paths and deployment settings correctly.
Common contexts for ENOENT errors?
ENOENT errors can occur in various programming contexts, including:
- Node.js: When working with the file system (fs) module, requiring non-existent modules, or handling file operations during build processes.
- Front-end development: When importing or requiring files in React, Next.js, or other front-end frameworks and libraries.
- Java: When working with file I/O operations, such as FileInputStream or FileReader.
- Ruby: When opening, creating, or managing files, or when working with dependencies and gems.
- Build tools and package managers: During build processes, dependency installations, or when accessing configuration files or output directories.
- Test automation frameworks: When running tests that involve file operations or accessing test data or configuration files.
Preventing ENOENT errors?
To prevent ENOENT errors, developers can adopt the following best practices:
- File management: Maintain a well-organized project structure, utilize version control systems, and regularly review and update file paths in your codebase and configurations.
- Optimizing build and runtime environments: Ensure compatibility and up-to-date dependencies, allocate sufficient system resources, and configure build output paths and deployment settings correctly.
- Defensive coding techniques: Implement proper error handling and error logging mechanisms, utilize try-catch blocks, check for file existence, and write unit tests to verify file operations.
- Stay up-to-date: Follow the latest developments, bug fixes, and discussions in the programming communities you work with to stay informed about potential issues and preventive measures related to ENOENT errors.