ESRCH Error Code 3: Causes, Fixes, Best Practices
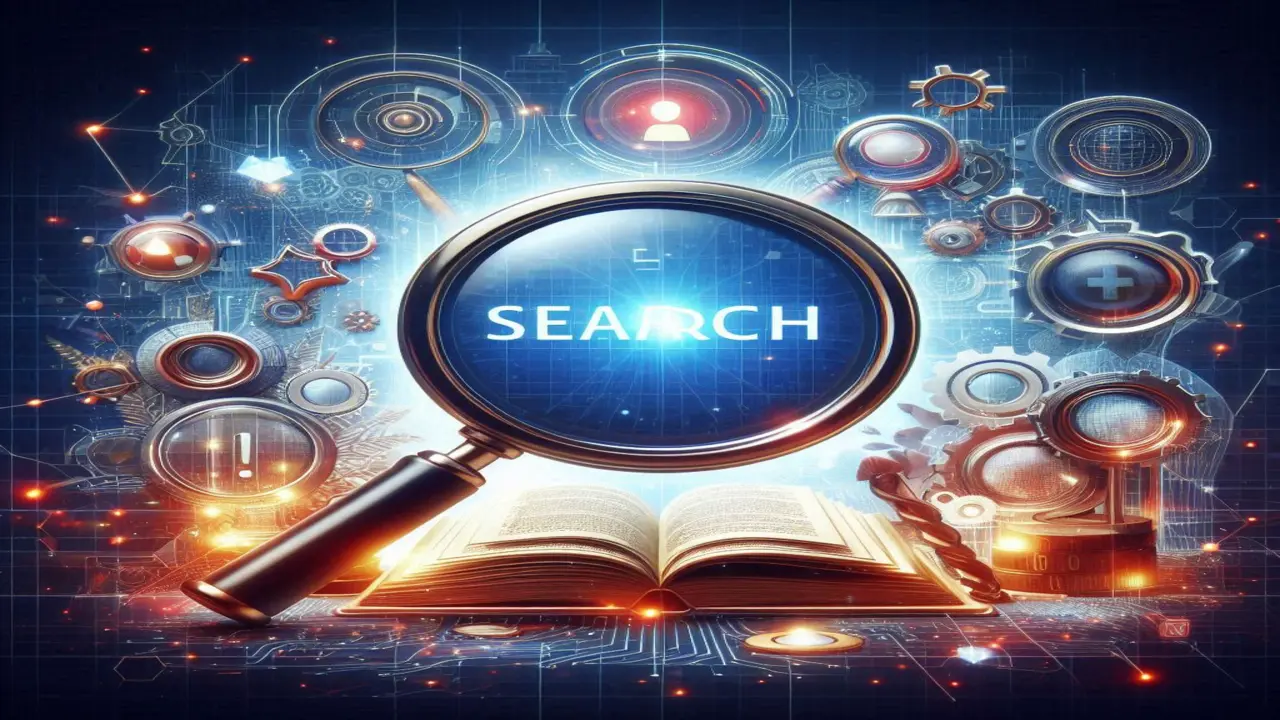
In the vast landscape of system programming and process management, few error codes are as ubiquitous and perplexing as ESRCH. This enigmatic acronym, standing for “Error – Search,” is a constant companion for developers navigating the complexities of Unix-like systems, Node.js applications, Java development, and even advanced search technologies. But what exactly is ESRCH, and why does it matter to developers, system administrators, and software engineers across various platforms?

ESRCH, officially known as Error Code 3 in most Unix-like systems, is fundamentally tied to the concept of process management. It typically emerges when an operation, such as sending a signal or terminating a process, is attempted on a non-existent process or process group. This seemingly simple error can manifest in a myriad of ways across different programming environments and tools, making it a crucial topic for anyone working in software development or system administration.
The importance of understanding ESRCH cannot be overstated. As systems become more complex and distributed, proper process management becomes increasingly critical. Mishandling ESRCH errors can lead to resource leaks, application instability, and in worst-case scenarios, system-wide issues. Moreover, as we’ll explore in this comprehensive guide, ESRCH isn’t just confined to traditional Unix systems – it has implications in modern development frameworks, process management tools like PM2, and even in the realm of enhanced search technologies.
In this in-depth exploration of ESRCH, we’ll journey through its origins in Unix systems, its manifestations in popular tools and languages like Node.js and Java, and its surprising connections to cutting-edge search technologies. We’ll delve into practical troubleshooting techniques, best practices for error handling, and strategies for future-proofing your applications against ESRCH-related issues.
Whether you’re a seasoned system administrator grappling with stubborn processes, a Node.js developer puzzled by unexpected termination errors, or a curious technologist interested in the intricacies of system-level programming, this guide aims to provide you with a comprehensive understanding of ESRCH. By the end of this article, you’ll not only be equipped to handle ESRCH errors more effectively but also gain insights into the underlying principles of process management and error handling across various technological domains.
ESRCH in Unix Systems: The Root of Error Code 3

The story of ESRCH begins in the heart of Unix systems, where it has been a fundamental part of process management and error handling for decades. To truly understand ESRCH, we need to delve into its origins, its meaning within the Unix context, and how it relates to the broader landscape of system calls and process control.
Origin and Meaning of ESRCH
ESRCH, which stands for “Error – Search,” is defined in the errno.h header file, a standard part of Unix-like operating systems. This error code is typically represented by the integer value 3, making it one of the most fundamental error codes in the Unix error handling ecosystem.
The “SRCH” in ESRCH directly relates to the concept of “searching” for a process. When a system call or operation attempts to interact with a process that doesn’t exist, the system returns ESRCH to indicate that the search for the specified process was unsuccessful.
To understand the significance of ESRCH, it’s crucial to recognize the fundamental role of processes in Unix-like systems. A process, in Unix terminology, is an instance of a running program. Each process is assigned a unique identifier known as a Process ID (PID). When operations like sending signals or terminating processes are performed, the system uses these PIDs to locate the target processes.
Here’s a simple representation of how ESRCH fits into the process management ecosystem:
Operation on Process --> System Searches for PID --> Process Found? --> Yes: Operation Proceeds --> No: ESRCH Returned
This straightforward mechanism ensures that system resources are not wasted on operations targeting non-existent processes.
ESRCH in Unix System Calls
The most common encounter with ESRCH occurs in the context of the kill() system call. The kill() function in Unix is used to send signals to processes or process groups. Despite its ominous name, kill() doesn’t necessarily terminate processes; it’s a general-purpose function for inter-process communication.
Here’s a typical usage of the kill() function in C:
#include <signal.h>
#include <errno.h>
#include <stdio.h>
int main() {
pid_t pid = 12345; // Example PID
if (kill(pid, SIGTERM) == -1) {
if (errno == ESRCH) {
fprintf(stderr, "No process with PID %d exists\n", pid);
}
}
return 0;
}
In this example, if the process with PID 12345 doesn’t exist, kill() will return -1 and set errno to ESRCH.
It’s important to note that ESRCH isn’t limited to the kill() function. Other system calls that interact with processes, such as waitpid(), setpgid(), or getpriority(), may also return ESRCH under similar circumstances.
ESRCH and Process Group Operations
ESRCH becomes particularly interesting when dealing with process groups. In Unix systems, processes can be organized into groups, allowing operations to be performed on multiple related processes simultaneously. When a system call targets a process group, ESRCH may be returned if the entire process group is non-existent, adding another layer of complexity to error handling.
For instance, consider this scenario involving process groups:
#include <signal.h>
#include <errno.h>
#include <stdio.h>
int main() {
pid_t pgid = 5000; // Example Process Group ID
if (killpg(pgid, SIGTERM) == -1) {
if (errno == ESRCH) {
fprintf(stderr, "No process group with PGID %d exists\n", pgid);
}
}
return 0;
}
Here, killpg() attempts to send a signal to all processes in the specified process group. If no such group exists, ESRCH is returned.
The Nuances of ESRCH in Modern Unix-like Systems
While the core concept of ESRCH remains consistent across Unix-like systems, it’s crucial to understand that its behavior can vary slightly depending on the specific implementation and kernel version.
For example, in Linux systems, the behavior of kill() and related functions has been refined over time. In older kernel versions, attempting to send a signal to PID 0 (which typically represents the calling process) would return ESRCH if the caller didn’t have permission to signal itself. In more recent versions, this scenario returns EPERM (Operation not permitted) instead, reserving ESRCH strictly for non-existent processes.
These subtle differences highlight the importance of consulting specific system documentation and man pages when dealing with ESRCH in production environments.
ESRCH in the Broader Context of Unix Error Handling
To fully appreciate ESRCH, it’s valuable to consider it within the broader framework of Unix error handling. Unix systems use a set of predefined error codes to communicate various error conditions. ESRCH, with its low error number of 3, is part of the core set of errors that have been present since the early days of Unix.
Here’s a comparison of ESRCH with some related error codes:
Error Code | Symbolic Constant | Typical Meaning |
1 | EPERM | Operation not permitted |
2 | ENOENT | No such file or directory |
3 | ESRCH | No such process |
4 | EINTR | Interrupted system call |
13 | EACCES | Permission denied |
Understanding these related error codes can help in developing more robust error handling strategies, as many system calls can return multiple error types depending on the specific failure condition.
Practical Implications of ESRCH in Unix Programming
For Unix system programmers and administrators, ESRCH serves as a crucial indicator in process management operations. Here are some key points to remember:
- Always check for ESRCH: When performing operations that interact with processes, always include checks for ESRCH in your error handling logic.
- Don’t assume process existence: The presence of a PID doesn’t guarantee that a process exists. Always be prepared to handle ESRCH, even if you’ve just obtained a PID.
- Consider race conditions: In multi-threaded or multi-process environments, be aware that a process might exit between the time you obtain its PID and when you attempt to interact with it.
- Use appropriate alternatives: In some cases, alternatives like waitpid() with the WNOHANG option can be used to check for process existence without risking ESRCH errors.
- Log and monitor: In production systems, logging ESRCH occurrences can provide valuable insights into application behavior and potential issues in process management logic.
By understanding the origins, meaning, and implications of ESRCH in Unix systems, developers and system administrators can build more robust, reliable applications and systems. This foundational knowledge serves as a stepping stone to understanding how ESRCH manifests in higher-level programming environments and tools, which we’ll explore in the subsequent sections of this guide.
ESRCH in Process Management Tools: The PM2 Perspective

As we move from the low-level Unix system calls to higher-level process management tools, ESRCH continues to play a significant role. One tool where ESRCH errors frequently surface is PM2, a popular process manager for Node.js applications. Understanding how ESRCH manifests in PM2 is crucial for developers and system administrators working with Node.js in production environments.
PM2 and ESRCH Errors: An Overview
PM2, or Process Manager 2, is a production process manager for Node.js applications, known for its ability to keep applications alive forever, reload them without downtime, and facilitate common system admin tasks. However, even with its robust features, PM2 is not immune to ESRCH errors, particularly in cluster mode.
The most common ESRCH-related issue in PM2 occurs when attempting to terminate processes. Users often encounter scenarios where PM2 reports that all processes have been stopped, but manual checks reveal that some processes are still running. This discrepancy can lead to resource leaks, unexpected behavior, and difficulties in deploying updates.
Let’s break down a typical ESRCH scenario in PM2:
- A Node.js application is started in cluster mode using PM2.
- The user attempts to stop the application using pm2 delete or pm2 stop.
- PM2 reports successful termination of all processes.
- Manual checks (e.g., using ps aux | grep node) show that some processes are still active.
- Attempts to restart the application may fail due to port conflicts with the lingering processes.
This scenario is often accompanied by the error message: “Process cannot be killed kill ESRCH (PM2 1.0.1) in cluster mode”.
Root Causes of ESRCH Errors in PM2
Several factors can contribute to ESRCH errors in PM2:
- Improper Signal Handling: If the Node.js application doesn’t properly handle SIGINT or SIGTERM signals, it may not shut down gracefully when PM2 attempts to stop it.
- Long-running Operations: Processes engaged in long-running operations may not respond to termination signals in a timely manner.
- Open Connections: Applications with open database connections or WebSocket connections may prevent the process from exiting cleanly.
- PM2 Version Issues: Some versions of PM2 have been reported to have issues with process termination, particularly in cluster mode.
- System Resource Constraints: In rare cases, system resource constraints can interfere with PM2’s ability to manage processes effectively.
Troubleshooting ESRCH Errors in PM2
When faced with ESRCH errors in PM2, consider the following troubleshooting steps:
- Update PM2: Ensure you’re using the latest version of PM2. Many issues are resolved in newer releases.
npm install pm2@latest -g
- Check Application Signal Handling: Review your Node.js application code to ensure it properly handles termination signals. Here’s a basic example:
process.on('SIGINT', () => {
console.log('Received SIGINT. Shutting down gracefully.');
// Close database connections, finish writing to files, etc.
process.exit(0);
});
- Use PM2’s Kill Method: If standard stop commands fail, try using PM2’s kill method:
pm2 kill
- Manual Process Termination: As a last resort, manually terminate lingering processes:
pkill -f "node /path/to/your/app.js"
- Analyze PM2 Logs: Check PM2 logs for any error messages or warnings:
pm2 logs
- Monitor System Resources: Use tools like top or htop to monitor system resources and identify any constraints that might be affecting PM2’s performance.
Best Practices to Prevent ESRCH Errors in PM2
To minimize ESRCH errors when working with PM2, consider implementing these best practices:
- Graceful Shutdown Handling: Implement proper shutdown procedures in your Node.js applications. This includes closing database connections, finishing write operations, and cleaning up resources.
- Use PM2 Ecosystem File: Utilize PM2’s ecosystem file to define application-specific settings, including shutdown timeouts. Here’s an example:
module.exports = {
apps: [{
name: "my-app",
script: "./app.js",
instances: "max",
exec_mode: "cluster",
kill_timeout: 5000, // Give processes 5 seconds to clean up
wait_ready: true, // Wait for process to send 'ready' signal
listen_timeout: 3000 // Wait 3 seconds for the app to listen
}]
}
- Regular Updates: Keep both PM2 and your Node.js version up to date to benefit from the latest improvements and bug fixes.
- Monitoring and Logging: Implement comprehensive logging in your application and use PM2’s monitoring features to catch issues early.
- Careful Use of Cluster Mode: While cluster mode offers performance benefits, it can complicate process management. Ensure your application is designed to work correctly in a clustered environment.
By understanding how ESRCH errors manifest in PM2 and implementing these best practices, developers can significantly reduce the occurrence of stubborn process termination issues and create more robust Node.js applications.
For more detailed information on PM2 and its features, visit the official PM2 documentation.
In the next section, we’ll explore how ESRCH errors surface in Node.js development outside of PM2, providing a broader perspective on process management in the Node.js ecosystem.
ESRCH in Node.js Development: Beyond Process Management Tools
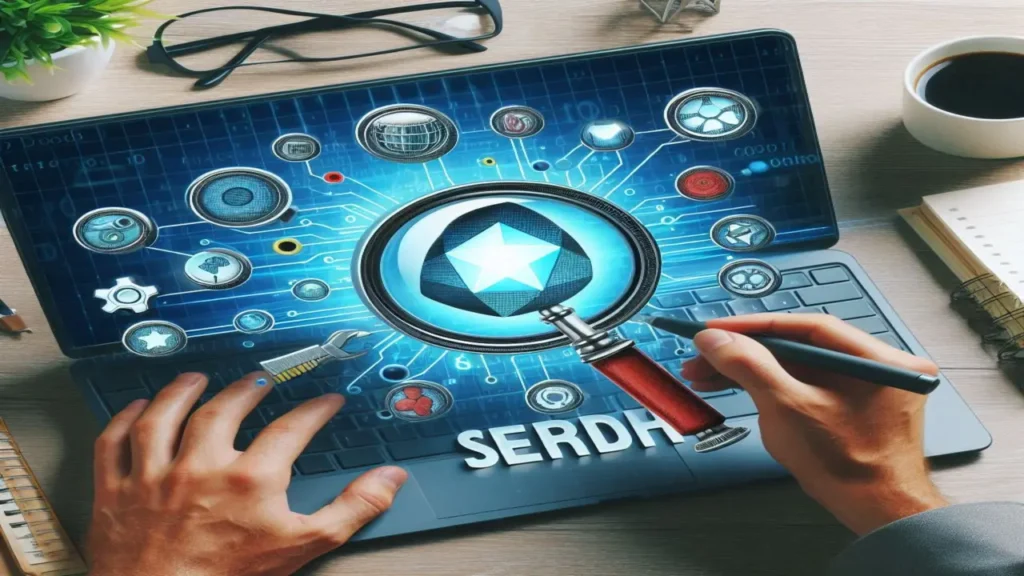
While PM2 is a common source of ESRCH encounters in the Node.js ecosystem, the error is not exclusive to process management tools. Node.js developers often face ESRCH errors in various scenarios, from simple script execution to complex application architectures. Understanding how ESRCH manifests in Node.js is crucial for writing robust, error-resistant applications.
”Error: kill ESRCH” in Node.js Applications
One of the most common ESRCH-related errors in Node.js is the “Error: kill ESRCH” message. This error typically occurs when attempting to terminate a process that no longer exists. Let’s break down the scenarios where this error might occur and how to handle it effectively.
Common Scenarios Leading to ESRCH in Node.js
- Asynchronous Process Termination: When terminating child processes asynchronously, race conditions can lead to ESRCH errors.
- Improper Error Handling in IPC: Inter-Process Communication (IPC) can sometimes result in ESRCH errors if not properly managed.
- Cluster Mode Operations: Similar to PM2 issues, Node.js cluster mode can sometimes lead to ESRCH errors during process management.
- Signal Handling in Long-Running Processes: Long-running processes that don’t properly handle signals can result in ESRCH errors when attempting to terminate them.
Here’s an example of code that might lead to an ESRCH error:
const { spawn } = require('child_process');
const child = spawn('long-running-process');
// Some time later...
setTimeout(() => {
try {
process.kill(child.pid);
} catch (error) {
if (error.code === 'ESRCH') {
console.error('Process already exited');
} else {
throw error;
}
}
}, 5000);
In this example, if the child process exits before the 5-second timeout, the process.kill() call will result in an ESRCH error.
Handling SIGINT and Connection Management
Proper signal handling and connection management are crucial for preventing ESRCH errors in Node.js applications. Let’s explore best practices for both:
SIGINT Handling in Node.js
SIGINT (Signal Interrupt) is typically sent when a user presses Ctrl+C. Properly handling this signal can prevent many ESRCH-related issues. Here’s an example of robust SIGINT handling:
process.on('SIGINT', async () => {
console.log('SIGINT received. Shutting down gracefully.');
// Close database connections
await closeDbConnections();
// Terminate any child processes
for (let child of childProcesses) {
try {
process.kill(child.pid);
} catch (error) {
if (error.code !== 'ESRCH') {
console.error(`Error terminating child process: ${error.message}`);
}
}
}
// Close any open file handles
await closeFileHandles();
process.exit(0);
});
Connection Management to Prevent ESRCH Errors
Proper connection management is essential for preventing ESRCH errors, especially in applications that maintain long-lived connections. Here’s a table summarizing best practices for different types of connections:
Connection Type | Best Practice |
Database | Use connection pooling and ensure all connections are closed before exit |
WebSockets | Implement a graceful closure mechanism for all open WebSocket connections |
File Handles | Use fs.promises for better async handling and ensure all file handles are closed |
Child Processes | Maintain a list of spawned processes and terminate them gracefully on exit |
Network Sockets | Implement proper error handling and timeout mechanisms for all network operations |
Troubleshooting ESRCH Errors in Node.js
When encountering ESRCH errors in Node.js applications, consider the following troubleshooting steps:
- Use Try-Catch Blocks: Wrap process termination code in try-catch blocks to handle ESRCH errors gracefully.
- Implement Logging: Use a robust logging solution to track process lifecycles and error occurrences.
- Utilize Process Events: Listen to process events like ‘exit’ and ‘error’ to better understand when and why processes are terminating.
- Check Process Existence: Before sending signals, check if the process exists using process.kill(pid, 0).
- Analyze Core Dumps: For complex issues, analyze core dumps to understand the state of the process when the error occurred.
Here’s an example of how to check for process existence before sending a signal:
function isProcessRunning(pid) {
try {
process.kill(pid, 0);
return true;
} catch (error) {
return error.code !== 'ESRCH';
}
}
// Usage
if (isProcessRunning(somePid)) {
process.kill(somePid, 'SIGTERM');
} else {
console.log('Process is not running');
}
ESRCH in Popular Node.js Frameworks and ORMs
ESRCH errors can also surface when working with popular Node.js frameworks and ORMs. For instance, users of Sequelize (a popular ORM for Node.js) have reported ESRCH errors when working with database connections in certain scenarios.
When using frameworks or ORMs, consider these additional best practices:
- Proper Shutdown Hooks: Implement shutdown hooks that close database connections and other resources.
- Transaction Management: Ensure all database transactions are properly committed or rolled back before process exit.
- Connection Pooling: Use connection pooling features provided by ORMs to manage database connections efficiently.
- Error Propagation: Ensure errors are properly propagated and handled at the application level.
For more information on handling process-related issues in Node.js, refer to the official Node.js documentation on process events.
By understanding the intricacies of ESRCH errors in Node.js and implementing robust error handling and process management strategies, developers can create more resilient and reliable applications. In the next section, we’ll explore how ESRCH manifests in Java development, providing a comparative perspective on error handling across different programming environments.

While ESRCH is traditionally associated with Unix-like systems and languages like C and Node.js, Java developers are not immune to its challenges. In the Java ecosystem, ESRCH errors can manifest in unique ways, particularly when dealing with native code integration and certain JDK versions. Understanding how ESRCH surfaces in Java is crucial for developers working on cross-platform applications or those leveraging Java’s native interface capabilities.
Platform Constant Error Code: ESRCH in JDK10
One notable occurrence of ESRCH in the Java world came to light with JDK10, particularly affecting libraries that interact closely with the operating system, such as lmdbjava (a Java binding for LMDB, the Lightning Memory-Mapped Database).
The lmdbjava Case Study
The issue with lmdbjava and JDK10 serves as an excellent case study for understanding how low-level system errors like ESRCH can bubble up to high-level Java applications. Here’s a breakdown of the problem:
- Error Manifestation: Users reported encountering “Platform constant error code: ESRCH No such process (3)” errors when using lmdbjava with JDK10.
- Root Cause: The error stemmed from changes in how JDK10 handled certain native method calls, particularly those involving process operations.
- Impact: This issue affected applications that relied on lmdbjava for high-performance, memory-mapped database operations.
To illustrate, consider the following Java code snippet that might trigger such an error:
import org.lmdbjava.*;
import java.nio.ByteBuffer;
public class LmdbExample {
public static void main(String[] args) {
try (Env<ByteBuffer> env = Env.create()
.setMapSize(10485760)
.open("/tmp/database")) {
// Database operations
} catch (LmdbException e) {
if (e.getMessage().contains("ESRCH")) {
System.err.println("Encountered ESRCH error. Possible JDK compatibility issue.");
}
}
}
}
Java API Changes and Their Impact on ESRCH Errors
The occurrence of ESRCH errors in Java often relates to changes in the Java API, particularly those affecting how Java interacts with the underlying operating system. Let’s explore some key areas where Java developers might encounter ESRCH-like issues:
Native Method Invocations
Java Native Interface (JNI) and Java Native Access (JNA) are common vectors for ESRCH errors to surface in Java applications. When native methods interact with system processes, they can encounter ESRCH under certain conditions.
Process Handling in Java
Java’s ProcessBuilder and Runtime.exec() methods for spawning external processes can sometimes lead to scenarios where ESRCH-like errors occur, especially when terminating processes.
Unsafe and JVM Internals
Changes to Java’s internal sun.misc.Unsafe class and other JVM internals can affect how certain low-level operations are handled, potentially leading to ESRCH-like errors in edge cases.
Best Practices for Handling ESRCH-like Errors in Java
To mitigate and handle ESRCH and similar low-level errors in Java applications, consider the following best practices:
- Version Compatibility Checks: Implement checks for JDK version compatibility, especially when using libraries that interact with native code.
- Robust Exception Handling: Use try-catch blocks to handle potential LmdbException or similar exceptions that might encapsulate ESRCH errors.
- Graceful Degradation: Design your application to gracefully degrade or provide alternative functionality when encountering ESRCH-like errors.
- Comprehensive Logging: Implement detailed logging to capture the context of ESRCH occurrences, aiding in debugging and issue resolution.
- Regular Dependency Updates: Keep your dependencies, especially those involving native code interactions, up to date to benefit from bug fixes and compatibility improvements.
Here’s an example of implementing some of these practices:
import java.util.logging.Logger;
public class RobustJavaApplication {
private static final Logger LOGGER = Logger.getLogger(RobustJavaApplication.class.getName());
public void performOperation() {
try {
// Operation that might trigger ESRCH-like error
nativeOperation();
} catch (UnsatisfiedLinkError | NoClassDefFoundError e) {
LOGGER.severe("Native operation failed. Possible ESRCH-like error: " + e.getMessage());
performAlternativeOperation();
}
}
private native void nativeOperation();
private void performAlternativeOperation() {
// Fallback logic
}
}
Comparing ESRCH Handling: Java vs. Unix-like Systems
To provide a comparative perspective, let’s look at how ESRCH handling in Java differs from traditional Unix-like systems:
Aspect | Unix-like Systems | Java |
Error Code | Directly accessible via errno | Encapsulated in exceptions or error messages |
Process Interaction | Direct through system calls | Abstracted through JVM and potentially JNI |
Signal Handling | Explicit signal handling required | Managed by JVM with limited direct access |
Error Granularity | Fine-grained error codes | Often generalized into broader exception types |
Resources for Java Developers Dealing with ESRCH
For Java developers encountering ESRCH or similar low-level errors, the following resources can be invaluable:
- Java Native Access (JNA) GitHub Repository: For understanding and troubleshooting native code interactions in Java.
- OpenJDK Bug Database: To check for known issues related to process handling and native operations in specific JDK versions.
- Java Process API Documentation: For understanding Java’s built-in process handling capabilities.
By understanding the nuances of ESRCH in the Java ecosystem and implementing robust error handling strategies, Java developers can create more resilient applications, especially when dealing with native code integrations or cross-platform development scenarios.
In the next section, we’ll explore how ESRCH concepts extend beyond traditional system programming into the realm of enhanced search technologies, providing a unique perspective on the broader applications of process and resource management principles.
ESRCH as Error Code 3 in System Programming

In the realm of system programming, particularly in Unix-like environments, error codes serve as a crucial communication mechanism between the kernel and user-space applications. Among these, ESRCH stands out as a fundamental error code that every system programmer should be intimately familiar with. Let’s dive deep into the significance of ESRCH across Unix-like systems and explore its specific manifestations in system calls like sendmsg.
Understanding ESRCH Across Unix-like Systems
ESRCH, represented by the integer value 3, is a standard error code defined in the <errno.h> header file. Its primary meaning is “No such process,” but its implications extend far beyond this simple description.
Definition and Significance in System Programming
In system programming, ESRCH typically indicates that an operation was attempted on a process or process group that does not exist. This error is crucial for several reasons:
- Resource Management: ESRCH helps prevent operations on non-existent processes, avoiding potential system instability or resource misuse.
- Error Handling: It allows applications to gracefully handle scenarios where target processes have unexpectedly terminated.
- Security: ESRCH can be part of the system’s security mechanism, preventing unauthorized access attempts to non-existent processes.
- Debugging Aid: For developers, ESRCH serves as a valuable indicator when troubleshooting issues related to inter-process communication or process management.
The significance of ESRCH becomes apparent when we consider its prevalence across various system calls. Here’s a table showcasing some common system calls that may return ESRCH:
System Call | Description | ESRCH Condition |
kill() | Send signal to a process | Target process doesn’t exist |
waitpid() | Wait for process to change state | Specified child process doesn’t exist |
setpriority() | Set process scheduling priority | Target process doesn’t exist |
ptrace() | Process trace | Target process doesn’t exist |
sched_setscheduler() | Set scheduling policy/parameters | Target process doesn’t exist |
Comparison with Related Error Codes
To fully grasp the role of ESRCH, it’s helpful to compare it with related error codes often encountered in system programming:
Error Code | Symbolic Constant | Typical Meaning | Comparison with ESRCH |
ESRCH (3) | ESRCH | No such process | Core error for non-existent processes |
EINVAL (22) | EINVAL | Invalid argument | May occur if PID is invalid, whereas ESRCH occurs if PID is valid but non-existent |
EPERM (1) | EPERM | Operation not permitted | May occur if process exists but caller lacks permission, while ESRCH indicates non-existence |
ECHILD (10) | ECHILD | No child processes | Specific to child processes, while ESRCH is more general |
EINTR (4) | EINTR | Interrupted system call | Can occur during blocking calls, unrelated to process existence |
Understanding these distinctions is crucial for accurate error handling and debugging in system-level programming.
The sendmsg System Call and ESRCH Error
The sendmsg system call is a powerful function used for sending messages through sockets, including the ability to send ancillary data. However, under certain conditions, sendmsg can fail with an ESRCH error, which might seem counterintuitive given ESRCH’s primary association with process management.
Overview of sendmsg Functionality
sendmsg is part of the socket API and is defined as follows:
ssize_t sendmsg(int sockfd, const struct msghdr *msg, int flags);
It’s used to send messages on a socket, whether or not it is connection-oriented. The msghdr structure allows for complex message composition, including scattered data segments and control information.
Key features of sendmsg:
- Supports sending data to multiple buffers (scatter/gather I/O)
- Allows sending of ancillary data (control information)
- Can be used with both SOCK_STREAM and SOCK_DGRAM socket types.
Causes of sendmsg Failures with ESRCH
The occurrence of ESRCH errors with sendmsg is less common and often more perplexing than ESRCH in process management contexts. Here are some scenarios where sendmsg might fail with ESRCH:
- Destination Process Termination: In some implementations, if the destination process of a Unix domain socket has terminated, sendmsg may return ESRCH.
- Race Conditions in Network Stack: In rare cases, race conditions in the network stack implementation can lead to ESRCH errors, particularly in high-load scenarios.
- Kernel Bugs: Certain kernel versions may have bugs that incorrectly return ESRCH for sendmsg under specific conditions.
- Security Modules Interference: Some security modules or firewalls might interfere with the normal operation of sendmsg, potentially leading to ESRCH errors.
A practical example of handling ESRCH in sendmsg:
#include <sys/socket.h>
#include <stdio.h>
#include <errno.h>
int send_message(int sockfd, const void *buf, size_t len) {
struct msghdr msg = {0};
struct iovec iov = {0};
iov.iov_base = (void *)buf;
iov.iov_len = len;
msg.msg_iov = &iov;
msg.msg_iovlen = 1;
if (sendmsg(sockfd, &msg, 0) == -1) {
if (errno == ESRCH) {
fprintf(stderr, "sendmsg failed: No such process (ESRCH)\n");
// Handle the error, possibly by reconnecting or cleaning up the socket
} else {
perror("sendmsg failed");
}
return -1;
}
return 0;
}
When dealing with ESRCH errors in sendmsg, consider the following best practices:
- Robust Error Handling: Always check for and handle ESRCH along with other potential errors.
- Logging and Monitoring: Log occurrences of ESRCH in sendmsg for further analysis.
- Retry Mechanisms: Implement intelligent retry mechanisms that can handle transient ESRCH errors.
- Keep Systems Updated: Ensure your kernel and system libraries are up-to-date to avoid known bugs.
- Network Stack Tuning: In high-performance scenarios, proper tuning of the network stack can help avoid race conditions.
For more detailed information on sendmsg and its error handling, refer to the Linux man page for sendmsg.
Understanding ESRCH in the context of system programming, especially in nuanced cases like sendmsg, is crucial for developing robust, high-performance systems. By grasping these concepts, developers can create more resilient applications that gracefully handle a wide range of error conditions.
ESRCH in Enhanced Search Technology

While ESRCH is primarily known as an error code in Unix-like systems, its principles have found an intriguing application in the realm of enhanced search technology. In this context, ESRCH takes on a new meaning: Enhanced Search and Retrieval for Content Handling. This innovative approach to search functionality draws inspiration from the efficiency and precision of Unix process management, applying similar principles to information retrieval.
Fundamentals of ESRCH in Search Systems
Enhanced Search and Retrieval for Content Handling (ESRCH) in search systems represents a paradigm shift in how we approach information retrieval and content management. At its core, ESRCH aims to provide more accurate, context-aware, and efficient search results by treating content elements as discrete, manageable entities, much like how Unix systems handle processes.
Key Features and Benefits
- Contextual Awareness: ESRCH systems understand the relationships between different pieces of content, allowing for more nuanced search results.
- Granular Content Management: Instead of treating documents as monolithic entities, ESRCH breaks content down into smaller, more manageable units.
- Real-time Updates: Similar to how Unix systems can update process states in real-time, ESRCH allows for dynamic updating of content relevance and relationships.
- Scalability: ESRCH systems are designed to handle large volumes of content efficiently, scaling horizontally much like distributed computing systems.
- Improved Relevance Scoring: By considering a wider array of contextual factors, ESRCH can provide more accurate relevance scores for search results.
- Flexible Query Handling: ESRCH systems can interpret and respond to more complex, nuanced queries that traditional search engines might struggle with.
Comparison with Traditional Search Methods
To understand the advantages of ESRCH, let’s compare it with traditional search methods:
Aspect | Traditional Search | ESRCH |
Content Unit | Typically whole documents | Granular content elements |
Context Awareness | Limited | High, considers relationships between content |
Update Frequency | Often batch-based | Real-time or near real-time |
Query Complexity | Generally simple keyword matching | Can handle complex, contextual queries |
Scalability | Can be challenging for very large datasets | Designed for high scalability |
Relevance Scoring | Based on keyword frequency and basic metrics | Considers context, relationships, and user behavior |
Implementing ESRCH for Improved Search Functionality
Integrating ESRCH into websites and applications requires a shift in how we think about content management and search functionality. Here’s a guide to implementing ESRCH for improved search capabilities:
Integration Steps for Websites and Applications
- Content Atomization:
- Break down content into smaller, meaningful units.
- Tag these units with relevant metadata.
- Example: Instead of indexing entire articles, break them down into paragraphs or concepts.
- Relationship Mapping:
- Establish connections between content units.
- Use techniques like graph databases to store these relationships.
- Tools like Neo4j can be valuable for this step.
- Enhanced Indexing:
- Develop an indexing system that captures not just keywords, but context and relationships.
- Consider using advanced indexing tools like Elasticsearch with custom plugins.
- Query Interpretation Engine:
- Implement natural language processing (NLP) to understand complex queries.
- Use libraries like spaCy or NLTK for advanced NLP capabilities.
- Real-time Update Mechanism:
- Develop a system for updating content relevance and relationships in real-time.
- Consider using stream processing tools like Apache Kafka for handling real-time updates.
- User Behavior Integration:
- Incorporate user interaction data to refine search results.
- Implement machine learning models to continuously improve relevance based on user behavior.
- API Development:
- Create a robust API that allows for complex query structures.
- Ensure the API can handle various content types and return granular results.
Optimization Techniques for ESRCH Performance
- Caching Strategies:
- Implement multi-level caching to store frequently accessed content and relationships.
- Use distributed caching systems like Redis for improved performance.
- Asynchronous Processing:
- Utilize asynchronous processing for non-critical updates to maintain system responsiveness.
- Consider using message queues like RabbitMQ for managing asynchronous tasks.
- Load Balancing:
- Implement intelligent load balancing to distribute search queries across multiple nodes.
- Tools like HAProxy can be effective for this purpose.
- Predictive Fetching:
- Develop algorithms to predict and pre-fetch likely-to-be-requested content.
- This can significantly reduce response times for common queries.
- Optimized Storage:
- Use columnar storage formats for analytical data to improve query performance.
- Consider technologies like Apache Parquet for efficient data storage.
- Incremental Updates:
- Implement incremental updating mechanisms to avoid full re-indexing.
- This is particularly important for large, frequently changing datasets.
- Query Optimization:
- Develop a query optimizer that can rewrite complex queries for improved performance.
- Utilize query execution statistics to continuously refine optimization strategies.
By implementing ESRCH principles and optimization techniques, websites and applications can offer a significantly enhanced search experience. Users benefit from more relevant, context-aware results, while content managers gain greater control and insight into how their content is discovered and utilized.
For further reading on advanced search technologies, the Apache Lucene project provides valuable insights into the underlying mechanisms of modern search engines.
As we continue to push the boundaries of search technology, ESRCH represents a promising direction, bridging the gap between traditional information retrieval and the nuanced, context-rich interactions that users increasingly expect in the digital age.
Cross-Platform ESRCH Considerations

As software development becomes increasingly cross-platform, understanding how ESRCH behaves across different operating systems and development tools is crucial. This section explores the nuances of ESRCH across various environments and provides strategies for effective testing and troubleshooting.
ESRCH Behavior Across Operating Systems and Tools
While ESRCH (Error – Search) is fundamentally a Unix concept, its manifestation and handling can vary significantly across different operating systems and development tools. Let’s explore these variations:
Comparing ESRCH on Linux, macOS, and other Unix variants
- Linux
- In Linux, ESRCH is consistently defined as error code 3 in the errno.h header.
- The Linux kernel’s process management is highly efficient, but ESRCH can still occur in complex multi-process scenarios.
- Example in C:
#include <errno.h>
#include <signal.h>
#include <stdio.h>
int main() {
if (kill(999999, 0) == -1 && errno == ESRCH) {
printf("Process does not exist (ESRCH on Linux)\n");
}
return 0;
}
- macOS (Darwin)
- macOS, being based on BSD, handles ESRCH similarly to Linux but with some subtle differences.
- The Darwin kernel may have slight variations in process management that can affect when ESRCH is raised.
- Objective-C example:
#import <Foundation/Foundation.h>
#import <errno.h>
#import <signal.h>
int main(int argc, const char * argv[]) {
@autoreleasepool {
if (kill(999999, 0) == -1 && errno == ESRCH) {
NSLog(@"Process does not exist (ESRCH on macOS)");
}
}
return 0;
}
- FreeBSD and Other Unix Variants
- FreeBSD and other Unix-like systems generally follow similar patterns, but may have unique quirks.
- Always consult the specific system’s documentation for precise behavior.
Language-specific and tool-specific ESRCH issues
- Node.js
- Node.js wraps system calls and may present ESRCH in its own way through the Error object.
- Example:
const { exec } = require('child_process');
exec('kill 999999', (error, stdout, stderr) => {
if (error && error.code === 'ESRCH') {
console.log('Process not found (ESRCH in Node.js)');
}
});
- Java
- Java abstracts away many system-level details, but ESRCH can still surface in native method calls.
- JNI (Java Native Interface) interactions may expose ESRCH errors.
- Example using:
Runtime.getRuntime().exec():
try {
Runtime.getRuntime().exec("kill 999999");
} catch (IOException e) {
if (e.getMessage().contains("No such process")) {
System.out.println("ESRCH-like error in Java");
}
}
- PM2
- As discussed earlier, PM2 can encounter ESRCH when managing Node.js processes, especially in cluster mode.
- PM2-specific command for checking process existence:
pm2 pid app_name
- Python
- Python’s os module provides access to ESRCH through OSError exceptions.
- Example:
import os
import errno
try:
os.kill(999999, 0)
except OSError as e:
if e.errno == errno.ESRCH:
print("Process not found (ESRCH in Python)")
Testing and Troubleshooting ESRCH Across Environments
Effectively managing ESRCH errors in cross-platform development requires a strategic approach to testing and troubleshooting.
Strategies for multi-platform testing
- Use Continuous Integration (CI) pipelines
- Set up CI/CD pipelines that test on multiple operating systems.
- Tools like GitHub Actions or GitLab CI/CD support multi-OS testing.
- Virtualization and containerization
- Use tools like VirtualBox or Docker to create isolated testing environments.
- Example Docker command for testing a Node.js app:
docker run --rm -v $(pwd):/app -w /app node:14 npm test
- Cross-platform development frameworks
- Utilize frameworks designed for cross-platform compatibility, like Electron for desktop apps or React Nativefor mobile.
- Automated testing tools
Resolving ESRCH errors in diverse development environments
- Environment-specific error handling
- Implement conditional logic based on the operating system:
const os = require('os');
if (os.platform() === 'darwin') {
// macOS-specific ESRCH handling
} else if (os.platform() === 'linux') {
// Linux-specific ESRCH handling
}
- Use cross-platform libraries
- Leverage libraries that abstract away OS differences, like cross-spawn for Node.js.
- Comprehensive logging
- Implement detailed logging to capture environment-specific information during ESRCH occurrences.
- Use logging frameworks like Winston for Node.js or logging for Python.
- Sandbox testing
- Create controlled environments to reproduce ESRCH errors:
# Create a test user and run process as that user
sudo useradd testuser
sudo -u testuser node your-test-script.js
- Process management best practices
- Implement robust process cleanup mechanisms.
- Use tools like node-cleanup for Node.js to ensure proper process termination.
By understanding the nuances of ESRCH across different platforms and implementing these testing and troubleshooting strategies, developers can create more robust, cross-platform applications that handle process-related errors gracefully.
For more in-depth information on cross-platform development challenges, consider exploring resources like the Node.js Cross-platform Working Group or the Java Platform Dependency Specification.
Best Practices for Handling ESRCH

As we’ve explored the intricacies of ESRCH across various contexts, from Unix systems to process management tools like PM2, it’s clear that effective handling of this error is crucial for robust software development. In this section, we’ll delve into best practices for managing ESRCH errors, focusing on system and network programming, as well as considerations for modern development environments.
Error Management in System and Network Programming
When dealing with ESRCH in system-level and network programming, implementing robust error checking and designing effective retry mechanisms are paramount. These practices not only help in gracefully handling ESRCH errors but also contribute to overall system stability and reliability.
Implementing Robust Error Checking
- Comprehensive Error Handling: Always check for ESRCH after system calls that can potentially return this error. This includes functions like kill(), waitpid(), and setpgid().
#include <errno.h>
#include <signal.h>
#include <stdio.h>
void send_signal_to_process(pid_t pid, int signal) {
if (kill(pid, signal) == -1) {
switch (errno) {
case ESRCH:
fprintf(stderr, "Process %d does not exist.\n", pid);
break;
case EPERM:
fprintf(stderr, "Permission denied to send signal to process %d.\n", pid);
break;
default:
fprintf(stderr, "Unexpected error: %s\n", strerror(errno));
}
}
}
- Use errno Correctly: Remember that errno is only set when a function returns an error indicator. Always check the return value before examining errno.
- Thread-Safe Error Handling: In multi-threaded applications, use strerror_r() instead of strerror() to avoid race conditions.
#define _GNU_SOURCE
#include <string.h>
#include <errno.h>
void log_error(int err) {
char buf[100];
char *msg = strerror_r(err, buf, sizeof(buf));
fprintf(stderr, "Error: %s\n", msg);
}
- Logging and Monitoring: Implement comprehensive logging for ESRCH occurrences. This can help in identifying patterns and potential issues in production environments.
Designing Effective Retry Mechanisms
When dealing with ESRCH errors, especially in networked or distributed systems, implementing retry mechanisms can improve reliability:
- Exponential Backoff: Implement an exponential backoff strategy for retries. This helps in avoiding unnecessary system load while still attempting to recover from transient errors.
import time
def retry_operation(operation, max_retries=5):
retries = 0
while retries < max_retries:
try:
return operation()
except OSError as e:
if e.errno != errno.ESRCH:
raise
time.sleep(2 ** retries)
retries += 1
raise Exception("Max retries exceeded")
- Conditional Retries: Not all ESRCH errors should be retried. Implement logic to distinguish between transient and permanent errors.
- Circuit Breaker Pattern: For operations that consistently fail with ESRCH, implement a circuit breaker to prevent unnecessary retries and protect system resources.
- Timeout Mechanisms: Implement timeouts for operations that might lead to ESRCH errors to prevent indefinite waiting.
For more advanced error handling techniques in system programming, the Linux Programming Interface by Michael Kerrisk is an excellent resource.
ESRCH Considerations in Modern Development
As development practices evolve, handling ESRCH errors requires adaptation to new paradigms and technologies. Here are key considerations for modern development environments:
Working with Potentially Deprecated APIs
- Stay Informed: Keep track of deprecation notices in the languages and frameworks you use. For example, in Node.js, the process.kill() method has undergone changes that affect how ESRCH errors are handled.
- Use Alternatives: When possible, use modern alternatives to deprecated APIs. For instance, in Node.js, consider using the worker_threads module for multi-processing instead of relying solely on child_process.
- Graceful Degradation: Implement fallback mechanisms for when deprecated APIs are removed. This ensures your application can still function, albeit potentially with reduced functionality.
- Feature Detection: Use feature detection techniques to determine the availability of APIs at runtime, allowing your code to adapt to different environments.
if (typeof process.kill === 'function') {
// Use process.kill
} else {
// Use alternative method
}
Ensuring Compatibility Across Language Versions, Frameworks, and Tools
- Version-Specific Error Handling: Be aware of how ESRCH behavior might change across different versions of your programming language or framework. Implement version-specific error handling where necessary.
import sys
if sys.version_info >= (3, 3):
# Use new error handling approach
else:
# Use legacy error handling
- Cross-Platform Considerations: When developing applications that run on multiple platforms, be aware of platform-specific differences in ESRCH behavior. Use conditional compilation or runtime checks to handle these differences.
- Framework-Specific Best Practices: Follow best practices for ESRCH handling specific to the frameworks you’re using. For example, in Express.js, use the error-handling middleware for consistent error management:
app.use((err, req, res, next) => {
if (err.code === 'ESRCH') {
res.status(404).send('Process not found');
} else {
next(err);
}
});
- Containerization Awareness: In containerized environments, be mindful of how process management and signaling work. ESRCH errors may manifest differently in containers compared to bare-metal or virtual machine environments.
- Microservices Considerations: In microservices architectures, handle ESRCH errors at the service boundary. Implement resilience patterns like retries, circuit breakers, and fallbacks to manage process-related errors between services.
By implementing these best practices and considerations, developers can create more robust, maintainable, and adaptable applications that gracefully handle ESRCH errors across a wide range of environments and scenarios.
For further reading on modern error handling practices, the Node.js Error Handling documentation and the Twelve-Factor App methodology provide valuable insights into building resilient applications in contemporary development landscapes.
Future-Proofing Against ESRCH Issues
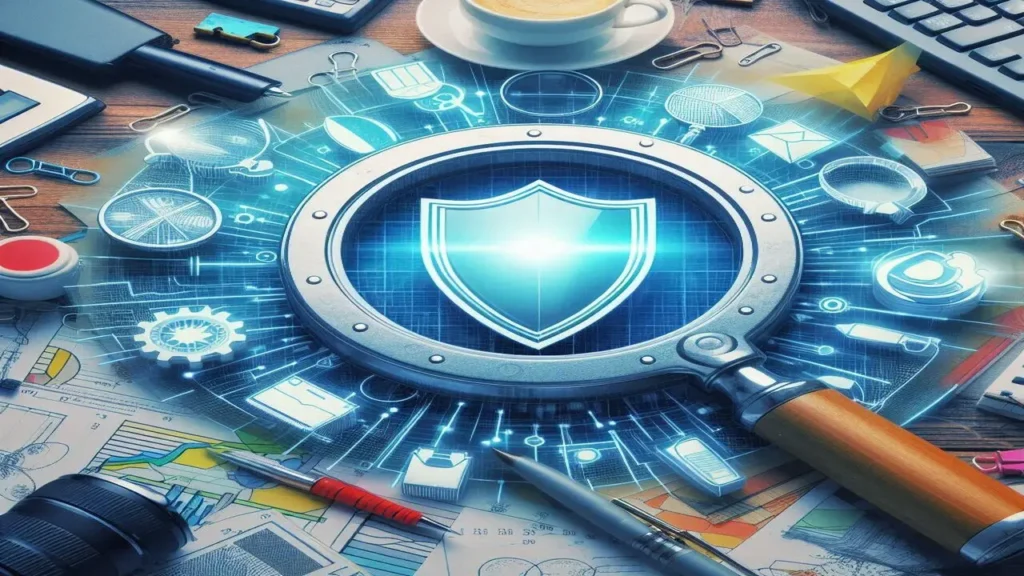
As technology evolves at a rapid pace, it’s crucial for developers and system administrators to stay ahead of potential ESRCH-related challenges. This section explores upcoming changes in various technologies and provides strategies for adapting to future ESRCH issues.
Upcoming Changes in Kernel, Node.js, Java, and Process Management Tools
The landscape of operating systems, programming languages, and process management tools is constantly shifting. Staying informed about proposed changes and community efforts is key to maintaining robust, ESRCH-resistant systems.
Kernel Development
Linux kernel development continues to refine process management and signal handling. Recent proposals and patches include:
- Improved Process Group Management: Enhancements to process group handling aim to reduce race conditions that can lead to ESRCH errors.
- Extended Process Information: Proposals for more detailed process state information could help tools like PM2 make better decisions about process termination.
- Unified Signal Handling: Efforts to standardize signal handling across different architectures may lead to more predictable ESRCH behavior.
For the latest kernel development news, check the Linux Kernel Mailing List Archives.
Node.js Evolution
Node.js continues to evolve, with changes that could impact ESRCH handling:
- Enhanced Worker Threads: Improved worker thread management may reduce ESRCH occurrences in multi-threaded Node.js applications.
- Async Hooks Refinements: Updates to the Async Hooks API could provide better insights into asynchronous operations, helping developers identify potential ESRCH triggers.
- Native Addons API Changes: Modifications to the N-API may affect how native addons interact with process management, potentially influencing ESRCH scenarios.
Stay updated with Node.js developments on the official Node.js blog.
Java Development
Java continues to evolve with each release, bringing changes that could affect ESRCH handling:
- Project Loom: This project aims to introduce lightweight threads to Java, potentially changing how Java applications handle concurrency and process management.
- JVM Improvements: Ongoing enhancements to the JVM may alter how it interacts with the underlying OS, potentially affecting ESRCH behavior.
- API Deprecations: As Java continues to modernize, some older APIs used for process management may be deprecated, requiring updates to existing code.
For Java update information, refer to the OpenJDK project page.
Process Management Tools
Tools like PM2 are also evolving to better handle ESRCH scenarios:
- Improved Graceful Shutdown: Enhanced algorithms for graceful shutdown aim to reduce instances of lingering processes.
- Better Integration with Container Technologies: As containerization becomes more prevalent, process managers are adapting to work more seamlessly in containerized environments.
- Advanced Monitoring Capabilities: Improved monitoring features could help identify potential ESRCH issues before they become critical.
Check the PM2 GitHub repository for the latest updates and feature proposals.
Preparing for API Changes and Deprecations
To future-proof your applications against ESRCH issues:
- Stay Informed: Regularly check documentation and release notes for your tech stack.
- Participate in Beta Testing: Engage in beta programs to test your applications against upcoming changes.
- Maintain a Flexible Architecture: Design your applications with modularity in mind to easily adapt to API changes.
- Implement Comprehensive Logging: Robust logging can help quickly identify and diagnose ESRCH issues as APIs evolve.
- Regular Code Audits: Periodically review your codebase for deprecated methods or APIs related to process management.
Emerging Technologies and ESRCH
As new technologies emerge, they bring both challenges and opportunities in managing ESRCH errors.
Potential Impacts on New Protocols and Systems
- Serverless Architectures: The rise of serverless computing may change how we think about process management, potentially reducing direct exposure to ESRCH errors.
- Edge Computing: As computation moves closer to the edge, new patterns of process management may emerge, requiring adapted ESRCH handling strategies.
- Quantum Computing: While still in its infancy, quantum computing may introduce entirely new paradigms of process management and error handling.
- AI-Driven Systems: Machine learning models could be employed to predict and prevent conditions leading to ESRCH errors.
Strategies for Adapting to Future ESRCH Challenges
- Embrace Containerization: Technologies like Docker can provide more predictable environments, potentially reducing ESRCH occurrences.
docker run --name my-node-app -d my-node-image
- Explore Unikernels: Unikernels, which combine the OS and application into a single, specialized machine image, may offer new approaches to process management.
- Adopt Infrastructure as Code: Tools like Terraform can help maintain consistent environments, reducing environment-specific ESRCH issues.
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "ESRCH-resistant-instance"
}
}
- Implement Chaos Engineering: Proactively test your systems’ resilience to ESRCH and other errors using chaos engineering principles.
- Explore Event-Driven Architectures: Event-driven systems may offer more resilient alternatives to traditional process-based architectures.
By staying informed about technological trends and adopting forward-thinking strategies, developers and system administrators can build more resilient systems that are better equipped to handle ESRCH challenges in the ever-evolving technological landscape.
For more insights on future trends in software development, check out the ThoughtWorks Technology Radar.
As we conclude this comprehensive guide to ESRCH, remember that the key to managing this persistent error lies in understanding its roots, staying informed about technological changes, and adopting best practices across your development and operational processes. With this knowledge, you’re well-equipped to tackle ESRCH errors both now and in the future.
Conclusion: Mastering ESRCH Across the Technology Spectrum
As we conclude our comprehensive exploration of ESRCH, it’s clear that this seemingly simple error code plays a crucial role across a wide range of technologies and development environments. From its roots in Unix systems to its manifestations in modern tools like PM2, programming languages such as Node.js and Java, and even in advanced search technologies, ESRCH serves as a critical indicator of process management and system health.
Recapping ESRCH Across Technologies
Let’s summarize the key points we’ve covered about ESRCH in various contexts:
- Unix Systems:
- ESRCH (Error Code 3) fundamentally means “No such process”
- Commonly encountered in system calls like kill(), waitpid(), and setpgid()
- Critical for low-level process management and signal handling
- PM2 (Process Manager 2):
- ESRCH errors often manifest as stubborn processes that refuse to terminate
- Can lead to resource leaks and deployment issues in Node.js applications
- Proper signal handling and graceful shutdown procedures are crucial
- Node.js:
- ESRCH can occur when terminating child processes or handling inter-process communication
- Proper error handling and understanding of Node.js’s asynchronous nature are essential
- Java:
- JDK10 and above may encounter ESRCH-like issues, especially with native libraries like lmdbjava
- API changes and deprecations (e.g., Unsafe removal) can indirectly lead to ESRCH-related problems
- Search Technology:
- In advanced search systems, ESRCH concepts are applied to optimize query processing and resource management
- Understanding ESRCH principles can lead to more efficient and robust search algorithms
- System Calls:
- ESRCH is a fundamental part of the error handling mechanism in system programming
- Proper checking for ESRCH is crucial in writing robust, system-level code
To visualize the pervasiveness of ESRCH across these technologies, consider the following table:
Technology | ESRCH Manifestation | Key Consideration |
Unix Systems | Direct error code from system calls | Fundamental to all process management operations |
PM2 | Difficult-to-terminate Node.js processes | Proper signal handling in Node.js applications |
Node.js | Child process and IPC issues | Asynchronous nature of Node.js |
Java | Native library interactions, API changes | JDK version compatibility |
Search Tech | Resource management in query processing | Applying ESRCH concepts to search optimization |
System Calls | Core error handling mechanism | Essential for robust system-level programming |
The Importance of Staying Informed
As technology continues to evolve, the way we interact with processes and handle errors like ESRCH is likely to change. Staying informed about ESRCH-related developments is crucial for several reasons:
- Evolving Best Practices: As new versions of operating systems, programming languages, and tools are released, best practices for handling ESRCH may change.
- Security Implications: Proper handling of ESRCH can prevent certain types of security vulnerabilities related to process management.
- Performance Optimization: Understanding ESRCH can lead to more efficient process management strategies, improving overall system performance.
- Cross-Platform Development: As development increasingly spans multiple platforms, a deep understanding of ESRCH helps in creating more portable and robust applications.
- Debugging Efficiency: Familiarity with ESRCH across different contexts can significantly speed up debugging processes in complex systems.
To stay updated on ESRCH-related developments:
- Regularly check official documentation for your operating system, programming languages, and tools.
- Follow relevant tech blogs and forums. For example:
- Participate in open-source communities related to process management tools and libraries.
- Attend or watch recordings of tech conferences focusing on system programming and process management.
Final Thoughts
ESRCH, while seemingly simple, touches on fundamental concepts in computing – process management, error handling, and system resource allocation. By developing a comprehensive understanding of ESRCH across various technologies, developers and system administrators can create more robust, efficient, and reliable systems.
As we continue to push the boundaries of what’s possible in software development and system administration, let’s not forget the importance of mastering these fundamental concepts. ESRCH may be just one error code, but it opens the door to a deeper understanding of how our complex computing systems function at their core.
Remember, in the world of technology, knowledge of the fundamentals like ESRCH is what separates good developers from great ones. Keep learning, stay curious, and never underestimate the power of understanding the deeper workings of our systems.
Frequently Asked Questions (FAQs) About ESRCH
To help you navigate the complexities of ESRCH across various environments, we’ve compiled a comprehensive list of frequently asked questions. These questions cover a wide range of topics related to ESRCH, from its basic definition to advanced troubleshooting techniques.
What does ESRCH stand for, and what are its different meanings?
ESRCH stands for “Error – Search.” In the context of Unix and Unix-like operating systems, it’s an error code typically represented by the integer 3. The meanings of ESRCH can vary slightly depending on the context:
- In Unix system calls: It generally means “No such process” when a process-related operation fails to find the specified process.
- In enhanced search technology: While not an error per se, ESRCH can refer to Enhanced Search algorithms or methodologies.
It’s crucial to note that the meaning is highly context-dependent, and in most programming scenarios, it refers to the “No such process” error.
How does “SRCH” in ESRCH relate to “No such process”?
The “SRCH” in ESRCH is an abbreviation for “search.” This directly relates to the error’s meaning of “No such process” in the following way:
- When a system call or operation attempts to interact with a process (e.g., sending a signal),
- The system performs a search for the specified process ID (PID),
- If this search fails (i.e., no process with the given PID is found),
- The system returns the ESRCH error, indicating that the search for the process was unsuccessful.
Thus, “SRCH” encapsulates the action of searching for a process, and the error indicates that this search failed to find the target process.
What causes ESRCH errors in Unix systems and process management tools like PM2?
ESRCH errors can occur due to various reasons in different environments. Here’s an overview of common causes:
In Unix systems:
- Attempting to send a signal to a non-existent process
- Race conditions where a process exits between checking its existence and performing an operation on it
- Permissions issues preventing access to process information
In PM2 and similar process management tools:
- Improper signal handling in the managed application
- Long-running operations preventing timely process termination
- Open connections (e.g., database, WebSocket) keeping processes alive
- Version-specific bugs in the process management tool
- System resource constraints interfering with proper process management
Understanding these causes is crucial for effective troubleshooting and prevention of ESRCH errors.
How can I troubleshoot process termination issues in PM2 that lead to ESRCH errors?
When facing ESRCH errors in PM2, especially in cluster mode, consider the following troubleshooting steps:
- Update PM2: Ensure you’re using the latest version:
npm install pm2@latest -g
- Check PM2 logs: Look for error messages or warnings:
pm2 logs
- Verify process status: Use system tools to check if processes are actually running:
ps aux | grep node
- Force kill PM2 processes: If normal stop commands fail:
pm2 kill
- Review application code: Ensure proper signal handling in your Node.js application.
- Analyze system resources: Use top or htop to check for resource constraints.
- Test with a simple app: Create a basic Node.js app to isolate PM2-specific issues from application-specific problems.
By systematically applying these steps, you can identify and resolve most ESRCH-related issues in PM2.
Why am I getting the “Error: kill ESRCH” in Node.js when exiting a program?
The “Error: kill ESRCH” in Node.js typically occurs when:
- An attempt is made to terminate a process that no longer exists.
- There’s a race condition between checking a process’s existence and attempting to terminate it.
- The application doesn’t properly handle termination signals (e.g., SIGINT from Ctrl+C).
Here’s a common scenario:
process.on('SIGINT', () => {
console.log('Received SIGINT. Shutting down gracefully.');
// Perform cleanup operations here
process.exit(0);
});
// Some long-running operation
setInterval(() => {
console.log('Working...');
}, 1000);
If the cleanup operations take too long, you might see the ESRCH error as the process tries to exit.
How can I properly handle SIGINT signals in my Node.js application to prevent ESRCH errors?
To properly handle SIGINT signals and prevent ESRCH errors:
- Register a SIGINT handler:
process.on('SIGINT', async () => {
console.log('Received SIGINT. Shutting down gracefully.');
await gracefulShutdown();
process.exit(0);
});
- Implement graceful shutdown:
async function gracefulShutdown() {
console.log('Closing database connections...');
await db.close();
console.log('Finishing remaining requests...');
await server.close();
console.log('Cleanup complete.');
}
- Set a timeout for shutdown:
const shutdownTimeout = setTimeout(() => {
console.error('Could not close connections in time, forcefully shutting down');
process.exit(1);
}, 10000);
shutdownTimeout.unref();
- Handle unhandled promise rejections:
process.on('unhandledRejection', (reason, promise) => {
console.error('Unhandled Rejection at:', promise, 'reason:', reason);
// Application specific logging, throwing an error, or other logic here
});
What causes the ESRCH “No such process (3)” error under JDK10 with lmdbjava?
The ESRCH error in the context of JDK10 and lmdbjava often stems from version-specific incompatibilities and API changes. Here are the key points to consider:
- JNI (Java Native Interface) Interactions: lmdbjava, being a Java wrapper for LMDB (Lightning Memory-Mapped Database), relies heavily on JNI. Changes in JDK10’s JNI implementation can lead to unexpected behaviors, including ESRCH errors.
- Unsafe API Changes: JDK10 introduced changes to the internal Unsafe API, which lmdbjava might be using for memory management. These changes can result in process-related errors that manifest as ESRCH.
- Native Library Loading: Issues with loading native libraries in JDK10 can sometimes be misinterpreted as process errors, leading to ESRCH.
To mitigate these issues:
- Ensure you’re using the latest version of lmdbjava compatible with JDK10.
- Check for any known issues or workarounds in the lmdbjava GitHub repository.
- Consider using JDK11 or later, which have further stabilized many of the changes introduced in JDK10.
The landscape of ESRCH-related issues is constantly evolving with new releases and updates. Here’s a overview of recent and upcoming changes:
Node.js:
- Worker Threads: Introduction of Worker Threads API in Node.js 10+ provides better handling of concurrent operations, potentially reducing scenarios leading to ESRCH errors.
- Improved Signal Handling: Recent versions of Node.js have enhanced signal handling, making it easier to manage process termination gracefully.
Java:
- Project Loom: Upcoming changes in Java concurrency model with Project Loom may affect how processes and threads are managed, potentially impacting ESRCH scenarios.
- Foreign Function & Memory API: This new API, evolving through JDK 17 and beyond, may change how Java interacts with native code, affecting libraries like lmdbjava.
Process Management Tools (e.g., PM2):
- Enhanced Monitoring: Tools like PM2 are continuously improving their monitoring capabilities, making it easier to detect and prevent situations that might lead to ESRCH errors.
- Container Integration: Better integration with containerization technologies is helping to isolate processes more effectively, reducing cross-process interference.
To stay updated:
- Regularly check the official documentation and release notes for Node.js, Java, and PM2.
- Follow relevant GitHub repositories and community forums for early insights into upcoming changes.
What are the best practices for preventing ESRCH errors in software development across different languages and tools?
Preventing ESRCH errors requires a multi-faceted approach across different programming environments. Here are some best practices:
- Robust Error Handling:
- Implement comprehensive try-catch blocks in Java and try-catch-finally in Node.js.
- Use conditional checks before process operations in system-level programming.
- Graceful Shutdown Mechanisms:
- Implement shutdown hooks in Java applications.
- Use process.on(‘SIGTERM’, …) handlers in Node.js.
- Resource Management:
- Properly close all resources (files, database connections) during shutdown.
- Utilize try-with-resources in Java and async/await patterns in Node.js for automatic resource management.
- Version Compatibility:
- Regularly update dependencies and test against the latest stable versions of your runtime environment.
- Use tools like npm-check-updates for Node.js and Maven Versions Plugin for Java to manage dependency versions.
- Monitoring and Logging:
- Implement comprehensive logging, especially around process management operations.
- Use APM (Application Performance Monitoring) tools to track process health and detect anomalies.
- Cross-Platform Testing:
- Test your applications on different operating systems to catch platform-specific ESRCH issues.
- Utilize CI/CD pipelines to automate cross-platform testing.
- Containerization:
- Use containerization technologies like Docker to ensure consistent environments across development and production.
How does ESRCH in enhanced search technology differ from ESRCH as a system error code?
While sharing the same acronym, ESRCH in enhanced search technology and as a system error code have distinct meanings and applications:
Aspect | ESRCH in System Programming | ESRCH in Enhanced Search |
Full Form | Error – Search (No such process) | Enhanced Search |
Primary Context | Process management and system calls | Information retrieval and search algorithms |
Occurrence | When a specified process doesn’t exist | When implementing advanced search functionalities |
Handling Mechanism | Through error codes and errno | Through algorithmic implementations |
Impact | System-level operations and process control | User-facing search results and performance |
In enhanced search technology, ESRCH often refers to techniques that improve search accuracy and efficiency. These may include:
- Advanced indexing methods
- Semantic analysis of search queries
- Machine learning algorithms for result ranking
Unlike the system-level ESRCH, which indicates an error condition, ESRCH in search technology is a feature set aimed at enhancing user experience.
For more information on enhanced search technologies, resources like Apache Lucene provide excellent starting points.
Are there specific configurations or dependencies that commonly trigger ESRCH errors in Node.js, Java, or PM2 applications?
Certain configurations and dependencies are more prone to causing ESRCH errors. Here’s a breakdown by environment:
Node.js:
- Child Process Module: Improper use of child_process.spawn() or child_process.fork() can lead to orphaned processes and ESRCH errors.
- PM2 Configuration: Incorrect settings in PM2 ecosystem files, particularly related to restart and kill timeouts.
Java:
- JNI Libraries: Native libraries not compatible with the JVM version can cause process-related errors.
- Security Managers: Overly restrictive security policies can prevent proper process management.
PM2:
- Cluster Mode: Applications not designed for clustering can face ESRCH issues when scaled.
- Watch Mode: Aggressive file watching settings can lead to frequent restarts and potential process orphaning.
Best Practices:
- Regularly audit your dependencies using tools like npm audit for Node.js and OWASP Dependency-Check for Java.
- Use LTS (Long Term Support) versions of Node.js and Java in production environments.
- Thoroughly test PM2 configurations in staging environments before deploying to production.
How can developers ensure proper handling of ESRCH errors in production environments using tools like PM2?
Ensuring proper handling of ESRCH errors in production requires a comprehensive approach:
- Implement Robust Logging:
- Use logging frameworks like Winston for Node.js or Log4j for Java.
- Configure PM2 to capture and rotate logs effectively.
// Example PM2 ecosystem file with logging configuration
module.exports = {
apps: [{
name: "my-app",
script: "./app.js",
error_file: "/var/log/my-app/err.log",
out_file: "/var/log/my-app/out.log",
log_date_format: "YYYY-MM-DD HH:mm Z"
}]
}
- Set Up Alerts and Monitoring:
- Use PM2’s built-in monitoring features or integrate with external monitoring services.
- Set up alerts for repeated ESRCH occurrences or unusual process behaviors.
- Implement Graceful Shutdown Procedures:
- Ensure your application handles SIGTERM signals properly.
- Use PM2’s wait_ready feature to ensure your app is fully initialized before accepting traffic.
- Regular Health Checks:
- Implement /health endpoints in your applications.
- Configure PM2 to periodically check these endpoints.
- Automate Recovery Procedures:
- Use PM2’s restart policies to automatically recover from crashes.
- Implement circuit breakers for external dependencies to prevent cascading failures.
- Conduct Regular Audits:
- Periodically review PM2 logs and metrics to identify patterns leading to ESRCH errors.
- Conduct post-mortem analyses after significant incidents involving ESRCH errors.
How can developers ensure proper handling of ESRCH errors in production environments using tools like PM2?
Handling ESRCH errors effectively in production environments, especially when using process managers like PM2, requires a multi-faceted approach:
- Implement Robust Logging:
- Use a logging framework like Winston or Bunyan in Node.js applications.
- Log all ESRCH occurrences with detailed context, including process IDs and the operation that triggered the error.
- Example logging setup:
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
defaultMeta: { service: 'user-service' },
transports: [
new winston.transports.File({ filename: 'error.log', level: 'error' }),
new winston.transports.File({ filename: 'combined.log' })
]
});
// Log ESRCH errors
process.on('uncaughtException', (error) => {
if (error.code === 'ESRCH') {
logger.error('ESRCH error occurred', {
errorMessage: error.message,
stack: error.stack,
pid: process.pid
});
}
});
- Set Up Comprehensive Monitoring:
- Utilize PM2’s built-in monitoring features or integrate with external monitoring services like New Relic or Datadog.
- Set up alerts for frequent ESRCH occurrences or patterns indicating potential issues.
- Implement Graceful Degradation:
- Design your application to handle ESRCH errors without crashing.
- Use try-catch blocks around operations that might result in ESRCH errors.
- Implement retry mechanisms for critical operations.
- Regular Health Checks:
- Implement health check endpoints in your application.
- Configure PM2 to restart processes that fail health checks.
- Proper Signal Handling:
- Ensure your application properly handles SIGTERM and SIGINT signals.
- Implement cleanup routines to close connections and free resources.
By implementing these practices, developers can significantly improve their application’s resilience to ESRCH errors in production environments.
What steps can be taken to troubleshoot ESRCH issues across different platforms, programming languages, and process management tools?
Troubleshooting ESRCH issues requires a systematic approach that can be adapted to various environments:
- Identify the Context:
- Determine the exact operation causing the ESRCH error.
- Note the platform, language, and tools involved.
- Check Process Status:
- Use platform-specific commands to verify process existence:
- Unix/Linux: ps aux | grep [process_name]
- Windows: tasklist | findstr [process_name]
- Use platform-specific commands to verify process existence:
- Analyze Logs:
- Review application logs, system logs, and tool-specific logs (e.g., PM2 logs).
- Look for patterns or events preceding the ESRCH error.
- Use Debugging Tools:
- For Node.js: Use the built-in debugger or tools like Visual Studio Code’s debugger.
- For Java: Utilize JConsole or VisualVM for process inspection.
- For system-level issues: Use strace (Linux) or Process Monitor (Windows).
- Check for Race Conditions:
- Review code for potential race conditions in process management.
- Use synchronization primitives where necessary.
- Version Compatibility:
- Verify compatibility between your application, runtime environment, and management tools.
- Check for known issues in the current versions of your tools and languages.
- Test in Isolated Environment:
- Reproduce the issue in a controlled, isolated environment to rule out system-wide interference.
- Language-Specific Approaches:
- Node.js: Use process.on(‘ESRCH‘, callback) to catch and handle ESRCH errors.
- Java: Implement try-catch blocks for ProcessHandle operations.
- PM2: Use pm2 describe [app_name] for detailed process information.
By following these steps, developers can effectively diagnose and resolve ESRCH issues across various platforms and technologies.
How does ESRCH impact user experience in search applications versus system-level operations and process management?
The impact of ESRCH errors varies significantly between search applications and system-level operations:
Context | Impact | Mitigation Strategies |
Search Applications | – Potential search result inaccuracies – Delayed response times – Incomplete search results | – Implement fault-tolerant search algorithms – Use caching mechanisms – Design for graceful degradation of search functionality |
System-Level Operations | – Process termination failures – Resource leaks – System instability | – Robust error handling in system scripts – Regular system health checks – Automated recovery procedures |
Process Management | – Orphaned processes – Inconsistent application state – Deployment failures | – Implement proper signal handling – Use process managers with built-in recovery mechanisms – Regular audits of running processes |
In search applications, ESRCH errors are less common but can lead to subtle issues in result accuracy or completeness. The impact on user experience is often less severe but can accumulate over time if not addressed.
For system-level operations and process management, ESRCH errors can have more immediate and noticeable impacts, potentially affecting system stability and application availability.
What are the recommended ways to handle existing connections when terminating processes to avoid ESRCH errors?
Properly handling existing connections during process termination is crucial to avoid ESRCH errors and ensure clean shutdowns. Here are recommended strategies for different environments:
- Node.js Applications:
- Use the server.close() method to stop accepting new connections and close existing ones gracefully.
- Implement a timeout for long-running connections:
const server = app.listen(port);
process.on('SIGTERM', () => {
console.log('SIGTERM received. Closing HTTP server.');
server.close(() => {
console.log('HTTP server closed.');
process.exit(0);
});
// Force close after 10 seconds
setTimeout(() => {
console.error('Could not close connections in time, forcefully shutting down');
process.exit(1);
}, 10000);
});
- Java Applications:
- Use : Runtime.getRuntime().addShutdownHook() to register shutdown procedures.
- Implement connection pooling with proper shutdown methods:
Runtime.getRuntime().addShutdownHook(new Thread() {
public void run() {
System.out.println("Shutting down...");
try {
// Close your connection pool here
connectionPool.close();
} catch (Exception e) {
e.printStackTrace();
}
}
});
- Database Connections:
- Implement connection pooling and ensure pools are closed during shutdown.
- Use try-with-resources (in Java) or equivalent constructs to automatically close connections.
- WebSocket Connections:
- Implement a broadcast mechanism to notify clients of impending shutdown.
- Set a timeout for clients to disconnect gracefully before forcing closure.
- Microservices Architecture:
- Implement circuit breakers to handle scenarios where dependent services are shutting down.
- Use service discovery mechanisms to handle dynamic service availability.
One thought on “ESRCH Error Code 3: Causes, Fixes, Best Practices”