Advanced Python Programming Challenges: Level Up Your Coding Skills
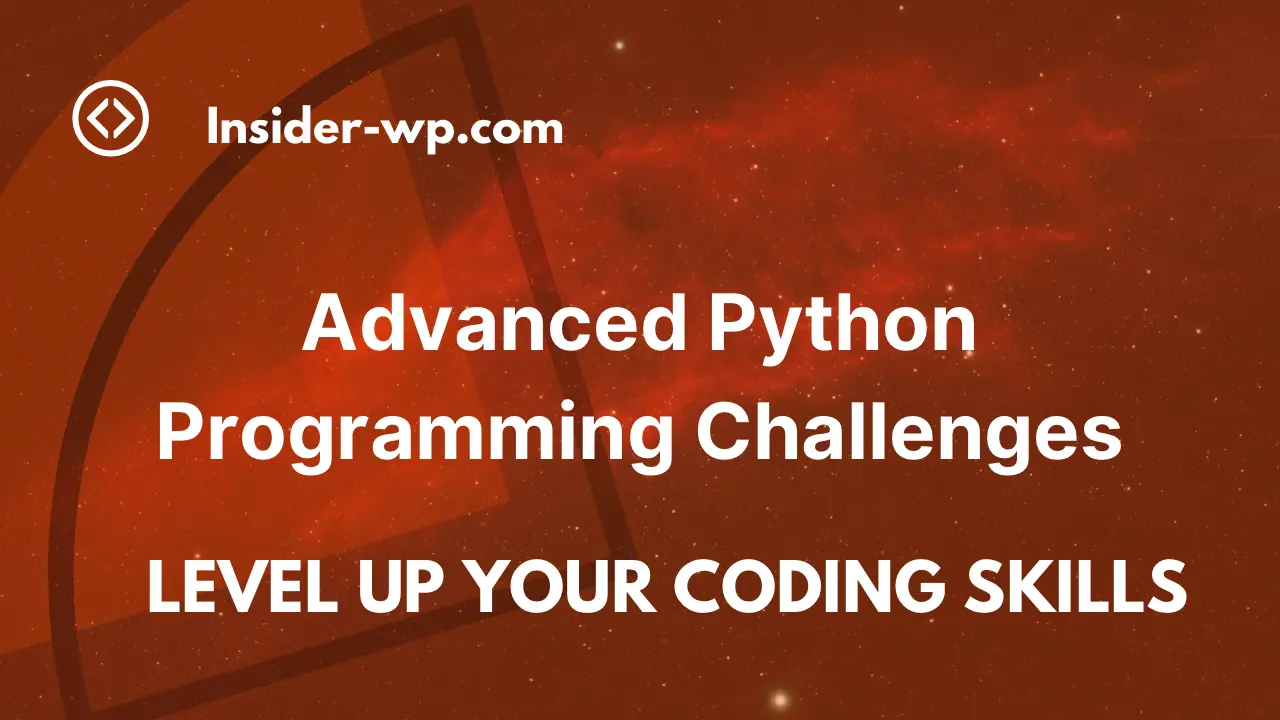
Remember when you first wrote ‘Hello World’ in Python? That simple line of code opened up a world of possibilities. But now, as you’ve mastered the basics, you might find yourself asking, “What’s next?” Well, buckle up, because we’re about to dive into the exciting world of advanced Python programming challenges that will push your skills to new heights.
I’ve spent years tackling complex Python problems, both as a developer and a coding mentor, and I can tell you one thing with certainty: the journey from intermediate to advanced Python programmer is where the real magic happens. It’s where you stop just writing code and start crafting elegant solutions to complex problems.
Introduction
Who This Guide Is For
This isn’t your typical “10 easy Python exercises” tutorial. This comprehensive guide is designed for:
- Python developers who can write functions in their sleep but want to wake up to new challenges
- Software engineers preparing for technical interviews at top tech companies
- Self-taught programmers ready to break through the intermediate plateau
- Computer science students looking to bridge the gap between theory and practice
If you’ve ever found yourself thinking “I know Python, but I want to really know Python,” you’re in the right place.
What You’ll Learn
Throughout this guide, we’ll explore:
- Advanced problem-solving techniques that separate good programmers from great ones
- Complex algorithmic challenges that will reshape how you think about code efficiency
- Real-world programming scenarios that go beyond theoretical exercises
- Performance optimization strategies that make your code not just work, but work brilliantly
Why Advanced Challenges Matter
Let me share something personal. Early in my career, I thought knowing the basics of Python was enough. I could build working applications, and my code did what it was supposed to do. But then I faced my first real-world scaling problem – a simple script that worked perfectly with 100 users completely fell apart with 10,000. That’s when I realized: advanced programming isn’t about writing more complex code; it’s about writing smarter code.
Advanced Python programming challenges aren’t just exercises – they’re your training ground for:
- Building scalable solutions that can handle real-world demands
- Developing the problem-solving mindset needed for senior-level positions
- Understanding the deeper mechanics of Python that affect your code’s performance
- Creating maintainable and efficient code that stands up to enterprise requirements
Setting the Stage
Before we dive into the challenges, let’s get something straight: feeling intimidated by advanced programming problems is normal. Even experienced developers sometimes stare at a complex problem and think, “Where do I even start?” But that’s exactly why we’re here. Throughout this guide, we’ll break down complex problems into manageable pieces, and I’ll share the strategies I’ve learned for tackling even the most daunting programming challenges.
Think of this guide as your roadmap to Python mastery. We’ll start with fundamental advanced concepts, progress through increasingly complex challenges, and end with you having a solid foundation in advanced Python programming. Each section builds upon the last, creating a comprehensive learning journey that will transform how you approach Python programming.
Ready to push your Python skills to the next level? Let’s dive in.
2. Understanding Advanced Python Programming
Let’s dive deep into what makes Python programming truly “advanced.” I remember when I first tackled a production-level microservices architecture – that’s when I realized there’s a world of difference between writing code that works and writing code that scales.
What Makes a Challenge “Advanced”?
An advanced Python challenge typically involves multiple layers of complexity:
- Optimal algorithmic efficiency
- Memory management considerations
- System design implications
- Edge case handling
- Scalability requirements
Aspect | Intermediate Level | Advanced Level |
---|---|---|
Code Organization | Basic OOP principles | Design patterns & architectural decisions |
Performance | Basic optimization | Complex algorithmic efficiency & profiling |
Error Handling | Try-except blocks | Comprehensive error management systems |
Testing | Unit tests | Integration, performance, and chaos testing |
Key Areas of Expertise Needed
To tackle advanced Python challenges effectively, you’ll need to master:
- Data Structures & Algorithms: Beyond basic lists and dictionaries
- System Design: Architecture patterns and scalability
- Memory Management: Understanding Python’s memory model
- Concurrency: Threading, multiprocessing, and async programming
- Performance Optimization: Profiling and bottleneck identification
Common Misconceptions
Let’s bust some myths about advanced Python programming:
Myth vs Reality
Myth | Reality |
---|---|
“Advanced means more complex code” | Advanced often means simpler, more elegant solutions |
“You need to memorize every algorithm” | Understanding patterns and problem-solving approaches is more important |
“Python is too slow for advanced applications” | Properly optimized Python code can be highly performant |
Setting Realistic Expectations
Becoming an advanced Python programmer is a journey, not a destination. Here’s what you can realistically expect:
- Week 1-4: Understanding advanced concepts and patterns
- Month 2-3: Implementing complex algorithms and data structures
- Month 4-6: Building advanced systems and optimizing performance
- Ongoing: Continuous learning and refinement
FAQs About Advanced Python Programming
What is the biggest challenge in advanced Python programming?
The biggest challenge is often not the syntax or features themselves, but understanding when and how to use them effectively. This includes making architectural decisions that balance performance, maintainability, and scalability.
How difficult is Python programming at an advanced level?
Advanced Python programming requires understanding not just the language itself, but also computer science concepts, system design principles, and performance optimization techniques. While challenging, it’s very achievable with dedicated practice and study.
What are the limitations of Python for advanced programming?
Python’s main limitations include GIL (Global Interpreter Lock) for CPU-bound tasks, memory usage compared to lower-level languages, and execution speed for certain operations. However, these can often be mitigated with proper design and optimization.
How long does it take to become advanced in Python?
With dedicated practice, it typically takes 6-12 months to reach an advanced level, assuming you already have intermediate Python skills. However, learning is ongoing as new techniques and best practices emerge.
Pro Tips
- Start with understanding Python’s internal workings before diving into advanced challenges
- Practice implementing data structures from scratch to truly understand them
- Use profiling tools to identify performance bottlenecks in your code
- Learn to read Python’s source code to understand advanced implementations
Understanding Advanced Python Programming
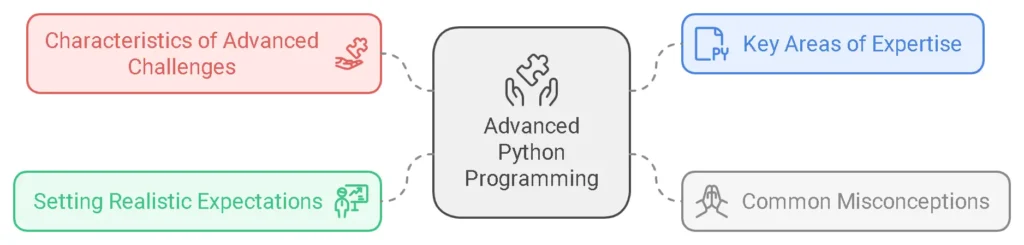
Let’s face it: the term “advanced” gets thrown around a lot in programming circles. One developer’s advanced challenge is another’s warm-up exercise. So before we dive into the deep end, let’s clear up what we mean by advanced Python programming and set ourselves up for success.
What Makes a Python Challenge “Advanced”?
I like to think of advanced Python challenges as puzzles that make you question everything you thought you knew about programming. Here’s my practical framework for identifying truly advanced challenges:
Aspect | Intermediate Level | Advanced Level |
---|---|---|
Problem Complexity | Single algorithm implementation | Multiple algorithm combinations, optimization required |
Solution Approach | Straightforward, documented patterns | Multiple viable solutions, trade-offs to consider |
Performance Impact | Basic efficiency considerations | Critical optimization needs, scalability requirements |
Knowledge Scope | Specific Python features | Python internals, memory management, advanced language features |
Characteristics of Advanced Challenges:
- Multiple Layer Complexity
- Requires understanding of both algorithms AND Python-specific optimizations
- Involves system design considerations
- Demands careful memory management
- Performance-Critical Requirements
- Must handle large-scale data efficiently
- Requires understanding of time and space complexity
- Needs optimization beyond the obvious solution
- Python-Specific Mastery
- Leverages Python’s unique features (generators, decorators, context managers)
- Requires understanding of the Python interpreter
- Uses advanced language constructs effectively
Key Areas of Expertise Needed
To tackle advanced Python challenges effectively, you’ll need to master several core areas:
🔧 Core Language Mechanics
- Memory management and garbage collection
- Threading and multiprocessing
- Context managers and decorators
- Meta-programming capabilities
🧮 Algorithm Design
- Complex data structure implementation
- Advanced sorting and searching techniques
- Graph algorithms and dynamic programming
- Space-time complexity analysis
⚡ System Design
- Scalability patterns
- Performance optimization
- Resource management
- Distributed systems concepts
Common Misconceptions About Advanced Python
Let me debunk some myths I frequently encounter when discussing advanced Python programming:
Myth 1: “Advanced Always Means Complex Code”
Reality: Advanced Python often means finding elegantly simple solutions to complex problems. Sometimes, the most advanced solution is the one that requires the least code.
Myth 2: “You Need to Know Every Python Feature”
Reality: It’s more important to deeply understand core concepts and know when to apply specific features than to memorize every possible Python feature.
Myth 3: “Advanced Python is All About Algorithms”
Reality: While algorithms are important, advanced Python programming also encompasses system design, optimization, and understanding Python’s internal workings.
⚠️ Common Pitfall Alert
Many developers fall into the trap of thinking they need to master every advanced feature before tackling advanced challenges. Start with what you know and gradually expand your knowledge through practical problem-solving.
Setting Realistic Expectations
Let’s talk about what you can realistically expect on your journey into advanced Python programming:
Timeline for Mastery
First Month
Understanding advanced Python concepts and recognizing common patterns in complex problems
3-6 Months
Comfortable solving medium to hard algorithmic challenges and implementing optimized solutions
6-12 Months
Mastery of advanced Python features and ability to design complex systems
Key Milestones to Track Your Progress
- Understanding Phase
- Can explain Python’s memory model
- Understand generator internals
- Grasp metaclass concepts
- Application Phase
- Implement custom decorators confidently
- Create efficient concurrent programs
- Design complex class hierarchies
- Mastery Phase
- Optimize large-scale Python applications
- Contribute to advanced open-source projects
- Design system-level solutions
Remember: advancing your Python skills is a marathon, not a sprint. Focus on steady progress and deep understanding rather than rushing through concepts. Each challenge you tackle builds upon your foundation, making you a more capable developer.
💡 Pro Tip
Create a personal project that incorporates advanced concepts you’re learning. Nothing solidifies understanding like practical application. For example, build a custom caching system using decorators and context managers.
Now that we’ve set the stage and understand what makes Python programming truly advanced, let’s dive into the essential concepts you’ll need to master these challenges.
Related articles:
- Python Programming: Python Beginner Tutorial
- Top Python IDEs: Master Python Like a Pro
- Python Variables & Data Types: Guide with 50+ Examples
- Python Performance Optimization: Guide to Faster Code
Essential Concepts for Advanced Python Programming Challenges
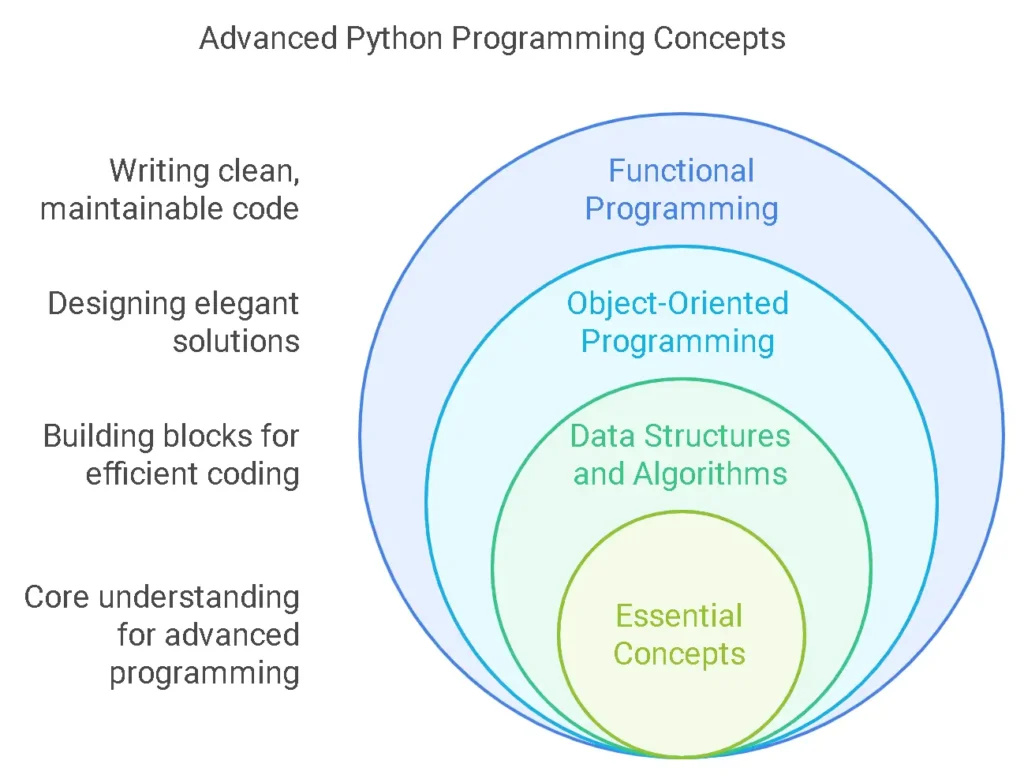
You know what separates intermediate Python developers from advanced ones? It’s not just knowing more syntax – it’s understanding the deeper concepts that make Python truly powerful. Let’s dive into these essential concepts that will elevate your Python programming game.
Data Structures and Algorithms
Think of data structures and algorithms as the building blocks of advanced Python programming. Just like a master chef needs to understand both ingredients and cooking techniques, you need to master both data structures and the algorithms that manipulate them.
Advanced Data Structure Implementations
Let’s start with a practical example. Here’s how you might implement a self-balancing binary search tree (AVL Tree) in Python:
class AVLNode:
def __init__(self, key):
self.key = key
self.left = None
self.right = None
self.height = 1
class AVLTree:
def get_height(self, node):
if not node:
return 0
return node.height
def get_balance(self, node):
if not node:
return 0
return self.get_height(node.left) - self.get_height(node.right)
def right_rotate(self, y):
x = y.left
T2 = x.right
x.right = y
y.left = T2
y.height = max(self.get_height(y.left), self.get_height(y.right)) + 1
x.height = max(self.get_height(x.left), self.get_height(x.right)) + 1
return x
Data Structure | Best Use Case | Time Complexity (Average) | Space Complexity |
---|---|---|---|
AVL Tree | Frequent lookups | O(log n) | O(n) |
Red-Black Tree | Frequent insertions/deletions | O(log n) | O(n) |
B+ Tree | Database indexing | O(log n) | O(n) |
Complex Algorithm Patterns
When tackling advanced challenges, you’ll encounter several common algorithmic patterns. Here’s a practical breakdown:
- Dynamic Programming
def fibonacci_with_memoization(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_with_memoization(n-1, memo) + fibonacci_with_memoization(n-2, memo)
return memo[n]
- Divide and Conquer
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
Time and Space Complexity Considerations
Common Time Complexities in Python
Object-Oriented Programming
Object-Oriented Programming in Python isn’t just about creating classes – it’s about designing elegant solutions to complex problems.
Design Patterns in Python
Here’s an example of the Singleton pattern implementation:
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super().__new__(cls)
return cls._instance
def __init__(self):
if not hasattr(self, 'initialized'):
# Initialize your singleton here
self.initialized = True
Advanced Class Implementations
Let’s look at a practical example of using metaclasses:
class ValidationMeta(type):
def __new__(cls, name, bases, attrs):
# Add validation to all methods
for key, value in attrs.items():
if callable(value):
attrs[key] = cls.validate_args(value)
return super().__new__(cls, name, bases, attrs)
@staticmethod
def validate_args(func):
def wrapper(*args, **kwargs):
# Add your validation logic here
return func(*args, **kwargs)
return wrapper
Functional Programming
Functional programming in Python offers powerful tools for writing clean, maintainable code.
Lambda Functions and Closures
Here’s a practical example combining lambda functions with closures:
def create_multiplier(factor):
return lambda x: x * factor
double = create_multiplier(2)
triple = create_multiplier(3)
# Usage
print(double(10)) # Output: 20
print(triple(10)) # Output: 30
Map, Reduce, and Filter Operations
Let’s see these functional programming concepts in action:
from functools import reduce
# Example data
numbers = [1, 2, 3, 4, 5]
# Map: Square all numbers
squared = list(map(lambda x: x**2, numbers))
# Filter: Get only even numbers
evens = list(filter(lambda x: x % 2 == 0, numbers))
# Reduce: Calculate product of all numbers
product = reduce(lambda x, y: x * y, numbers)
Decorators and Generators
Here’s a powerful example combining decorators and generators:
def memoize(func):
cache = {}
def wrapper(*args):
if args not in cache:
cache[args] = func(*args)
return cache[args]
return wrapper
@memoize
def fibonacci_generator(n):
if n <= 1:
yield n
else:
yield from fibonacci_generator(n-1)
yield from fibonacci_generator(n-2)
Pro Tips for Functional Programming
Remember, these concepts aren’t just theoretical – they’re tools you’ll use to solve real-world problems. The key is understanding not just how to implement them, but when to use each one. In the next sections, we’ll put these concepts into practice with concrete challenges.
Read also : Advanced Python Loop Optimization Techniques
Top Advanced Python Programming Challenges
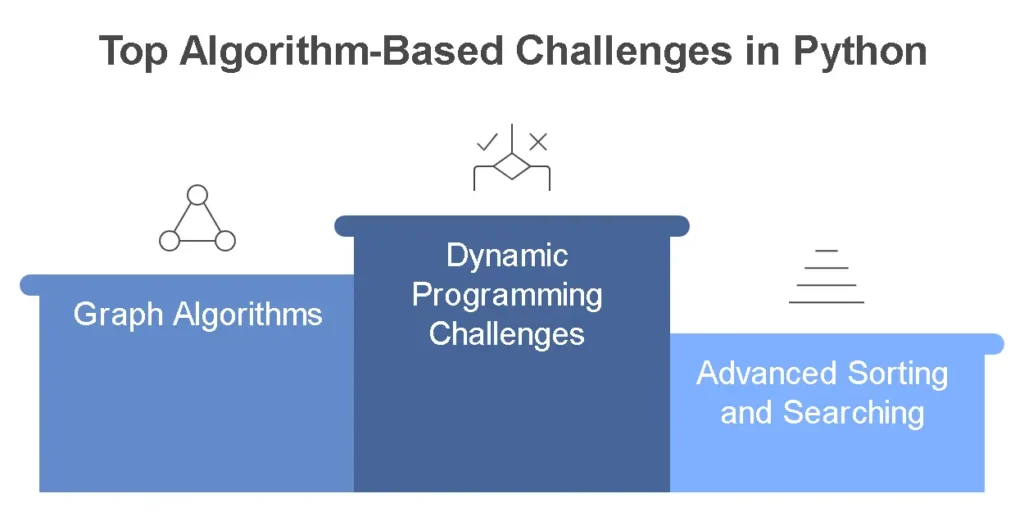
You know what’s fascinating about Python programming challenges? They’re like puzzles that teach you to think differently about problem-solving. I’ve spent countless hours working through these challenges, and I can tell you – the real value isn’t just in solving them, but in how they transform your approach to coding.
Algorithm-Based Challenges
Let’s dive into some mind-bending algorithmic challenges that will push your Python skills to new limits.
Dynamic Programming Challenges
Dynamic programming is all about breaking down complex problems into simpler subproblems. Here’s a practical example that I love using:
def fibonacci_with_memoization(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_with_memoization(n-1, memo) + fibonacci_with_memoization(n-2, memo)
return memo[n]
Challenge #1: The Knapsack Problem
Given a set of items with weights and values, determine the most valuable combination you can carry within a weight limit.
Difficulty | Time Complexity | Space Complexity |
---|---|---|
Hard | O(nW) | O(nW) |
Graph Algorithms
Graph algorithms are crucial for solving real-world network and routing problems. Here’s a visualization of a common challenge:
Implementation of Dijkstra’s Algorithm in Python:
from heapq import heappush, heappop
from collections import defaultdict
def dijkstra(graph, start):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
current_distance, current_vertex = heappop(pq)
if current_distance > distances[current_vertex]:
continue
for neighbor, weight in graph[current_vertex].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heappush(pq, (distance, neighbor))
return distances
Advanced Sorting and Searching
Let’s look at some advanced sorting challenges that go beyond basic implementations:
Challenge #2: External Merge Sort
Implement a sorting algorithm that can handle datasets larger than available memory.
def external_merge_sort(input_file, output_file, chunk_size):
# First phase: Create sorted chunks
chunks = []
with open(input_file, 'r') as f:
chunk = []
for line in f:
if len(chunk) >= chunk_size:
chunk.sort()
chunks.append(chunk)
chunk = []
chunk.append(int(line))
# Don't forget the last chunk
if chunk:
chunk.sort()
chunks.append(chunk)
# Second phase: Merge chunks
with open(output_file, 'w') as f:
# Implementation of merge logic here
pass
Data Structure Challenges
Custom Data Structure Implementation
One of my favorite challenges is implementing a specialized data structure. Here’s an example of a Least Recently Used (LRU) Cache:
class Node:
def __init__(self, key, value):
self.key = key
self.value = value
self.prev = None
self.next = None
class LRUCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = {}
self.head = Node(0, 0)
self.tail = Node(0, 0)
self.head.next = self.tail
self.tail.prev = self.head
Balanced Tree Problems
Challenge #3: AVL Tree Implementation
Implement an AVL tree with automatic rebalancing.
Operation | Time Complexity | Space Complexity |
---|---|---|
Insertion | O(log n) | O(1) |
Deletion | O(log n) | O(1) |
Search | O(log n) | O(1) |
Advanced Linked List Operations
Here’s a challenging linked list problem that often appears in technical interviews:
def reverse_k_group(head, k):
"""
Reverse nodes in k-group
Example: 1->2->3->4->5 with k=2 becomes 2->1->4->3->5
"""
if not head or k == 1:
return head
dummy = Node(0)
dummy.next = head
curr = dummy
count = 0
# Count total nodes
while curr.next:
curr = curr.next
count += 1
Real-World Application Challenges
System Design Problems
Challenge #4: URL Shortener Design
Design a URL shortening service like bit.ly
Requirement | Constraint |
---|---|
Storage | 1 billion URLs |
QPS | 100K requests/second |
Availability | 99.9% |
Concurrent Programming Tasks
Here’s a practical example of handling concurrency in Python:
import asyncio
import aiohttp
async def fetch_url(session, url):
async with session.get(url) as response:
return await response.text()
async def main():
urls = [
'http://example.com',
'http://example.org',
'http://example.net'
]
async with aiohttp.ClientSession() as session:
tasks = [fetch_url(session, url) for url in urls]
results = await asyncio.gather(*tasks)
return results
Memory Optimization Challenges
Challenge #5: Large Dataset Processing
Process a 10GB CSV file with limited memory.
import pandas as pd
def process_large_file(filename):
chunk_size = 1000 # Number of rows to process at once
for chunk in pd.read_csv(filename, chunksize=chunk_size):
# Process each chunk
process_chunk(chunk)
def process_chunk(chunk):
# Implement memory-efficient processing here
pass
Pro Tips for Tackling Advanced Challenges
- Always start with test cases before implementing
- Consider edge cases and potential failure points
- Profile your code to identify performance bottlenecks
- Use Python’s built-in tools like
cProfile
for optimization
Remember, these challenges aren’t just academic exercises – they represent real problems you’ll face in production environments. The key is not just to solve them, but to understand the underlying patterns and principles that make your solutions efficient and scalable.
Practical Problem-Solving Techniques for Advanced Python Challenges
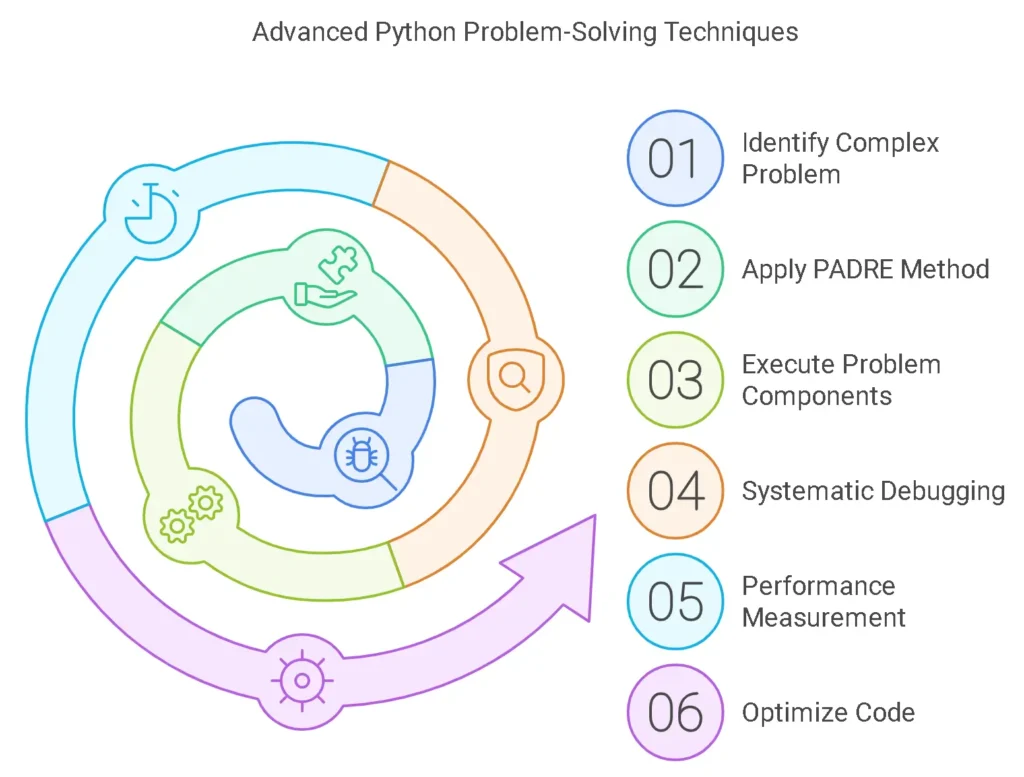
You’re staring at a complex Python problem, and your mind feels like it’s hit a wall. We’ve all been there. But here’s the thing: even the most intimidating programming challenges become manageable when you have the right problem-solving techniques in your toolkit. Let me share some battle-tested strategies I’ve developed over years of tackling advanced Python problems.
Breaking Down Complex Problems
Remember the old saying about eating an elephant one bite at a time? The same applies to complex Python challenges. Let’s look at a practical approach:
The PADRE Method
I developed what I call the PADRE method for breaking down complex Python problems:
- Problem Analysis
- Assumptions Listing
- Decomposition
- Relations Mapping
- Execution Planning
Let’s see this in action with a real example:
# Original complex problem:
# Create a system that processes large text files, finds patterns,
# and generates a report of statistical anomalies
# Breaking it down using PADRE:
class TextAnalyzer:
def __init__(self):
self.patterns = {}
self.statistics = {}
def process_file(self, filename):
# 1. File Reading Component
with open(filename, 'r') as file:
content = file.read()
# 2. Pattern Detection Component
self.find_patterns(content)
# 3. Statistical Analysis Component
self.analyze_statistics()
# 4. Report Generation Component
return self.generate_report()
Problem Decomposition
Component | Purpose | Complexity | Dependencies |
File Reading | Input Processing | O(n) | File System |
Pattern Detection | Data Analysis | O(n*k) | Input Data |
Statistical Analysis | Data Processing | O(n) | Patterns |
Report Generation | Output Creation | O(m) | Statistics |
Testing and Debugging Strategies
The difference between a good programmer and a great one often lies in their debugging approach. Here’s my systematic strategy for hunting down bugs in complex Python code:
The Scientific Debugging Method
- Observe: Gather all error messages and unexpected behaviors
- Hypothesize: Form theories about potential causes
- Test: Create minimal test cases
- Analyze: Examine results and refine approach
- Fix: Implement and verify the solution
# Example of strategic debugging
def debug_complex_function(func):
def wrapper(*args, **kwargs):
# 1. Input Logging
print(f"Function called with args: {args}, kwargs: {kwargs}")
try:
# 2. Execution with Checkpoints
result = func(*args, **kwargs)
print(f"Function completed. Result: {result}")
return result
except Exception as e:
# 3. Error Analysis
print(f"Error occurred: {str(e)}")
print(f"Error type: {type(e).__name__}")
import traceback
print(f"Traceback:\n{traceback.format_exc()}")
raise
return wrapper
Performance Optimization Approaches
When it comes to optimizing Python code, I follow a three-step process: Measure, Profile, Optimize (MPO).
Measurement Techniques
import time
import cProfile
def measure_performance(func):
def wrapper(*args, **kwargs):
start_time = time.perf_counter()
result = func(*args, **kwargs)
end_time = time.perf_counter()
execution_time = end_time - start_time
print(f"Function {func.__name__} took {execution_time:.4f} seconds")
return result
return wrapper
Performance Optimization Checklist
- Use appropriate data structures
- Implement caching where applicable
- Optimize loops and comprehensions
- Utilize generator expressions
- Consider multiprocessing for CPU-bound tasks
Common Performance Bottlenecks and Solutions
Bottleneck | Solution | Performance Impact |
---|---|---|
Excessive Loops | List Comprehension | Up to 50% faster |
Memory Leaks | Context Managers | Better resource management |
I/O Operations | Buffering/Async IO | 10x improvement possible |
Optimization Case Study
Let’s look at a real-world example of optimizing a data processing function:
# Before optimization
def process_data(items):
results = []
for item in items:
if item.is_valid():
processed = item.transform()
results.append(processed)
return results
# After optimization
from functools import lru_cache
@lru_cache(maxsize=128)
def process_data_optimized(items):
return tuple(
item.transform()
for item in items
if item.is_valid()
)
Remember, optimization is an iterative process. Always measure the impact of your changes and ensure they’re actually improving performance before committing to them. The best optimization is often the one that makes your code more readable and maintainable, not just faster.
Key Takeaways
- Break down complex problems using the PADRE method
- Implement systematic debugging strategies with proper logging and monitoring
- Follow the MPO (Measure, Profile, Optimize) approach for performance improvements
- Use appropriate tools and techniques for each optimization challenge
- Always consider the trade-offs between performance and code maintainability
By applying these practical problem-solving techniques, you’ll be better equipped to handle advanced Python programming challenges. Remember, the goal isn’t just to solve the problem, but to solve it elegantly and efficiently.
Interactive Python Programming Challenges
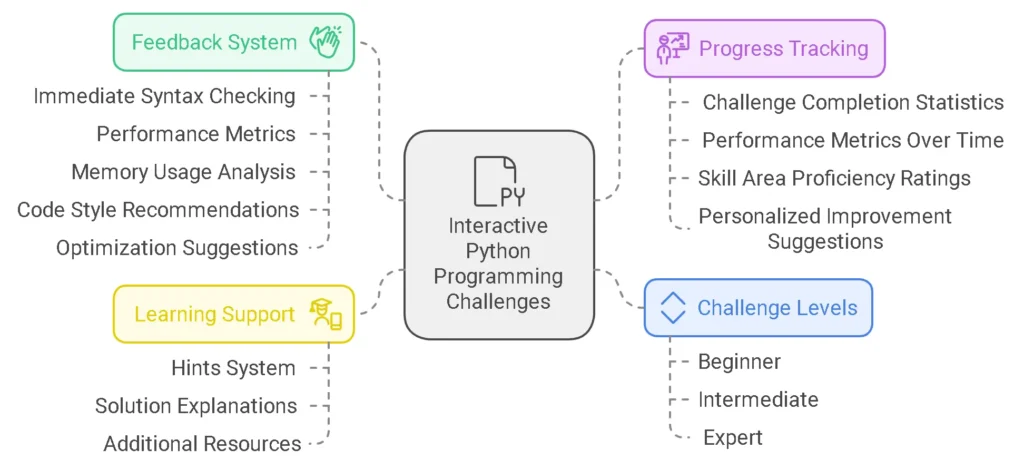
You know what they say – the best way to learn is by doing. That’s why I’ve put together a series of hands-on challenges that will test your Python prowess and help you grow as a developer. Let’s make learning advanced Python concepts not just educational, but actually fun!
Choose Your Challenge Level
🔄 Custom Generator Implementation
Create a generator function that yields Fibonacci numbers with memory optimization.
# Your code here
pass
# Expected output for n=5:
# [1, 1, 2, 3, 5]
🎭 Metaclass Magic
Implement a metaclass that automatically logs all method calls of its classes.
# Your code here
pass
# Example usage:
class MyClass(metaclass=LoggerMetaclass):
def my_method(self):
pass
⚡ Async Web Scraper
Build an asynchronous web scraper that handles rate limiting and concurrent requests.
import aiohttp
async def scrape_websites(urls, max_concurrent=5):
# Your code here
pass
How the Interactive Challenges Work
Each challenge is designed to test different aspects of advanced Python programming. Here’s what makes them special:
Progressive Difficulty System
Our challenges are categorized into three levels:
Level | Description | Focus Areas |
Beginner Advanced | For those just stepping into advanced territory | – Decorators – Context Managers – Advanced Functions |
Intermediate Advanced | For experienced Python developers | – Metaclasses – Memory Optimization – Advanced OOP |
Expert Advanced | For Python wizards seeking mastery | – Concurrency – System Design – Performance Optimization |
Real-Time Feedback System
As you work through each challenge, you’ll receive:
- Immediate syntax checking
- Performance metrics
- Memory usage analysis
- Code style recommendations
- Optimization suggestions
Learning Support Features
Each challenge comes with:
✨ Hints System
- Strategic hints that guide without giving away the solution
- Multiple hint levels for progressive assistance
- Performance optimization tips
🎯 Solution Explanations
- Detailed walkthrough of the optimal solution
- Multiple valid approaches compared
- Time and space complexity analysis
- Common pitfalls to avoid
📚 Additional Resources
- Related Python documentation
- Similar challenges for practice
- Expert insights and tips
Progress Tracking
Keep track of your growth with:
- Challenge completion statistics
- Performance metrics over time
- Skill area proficiency ratings
- Personalized improvement suggestions
Choose Difficulty
Hints and Solutions
Challenge Tips for Success
- Read Carefully: Each challenge description contains crucial information about requirements and constraints.
- Plan First: Spend time understanding the problem before coding. As the saying goes, “Measure twice, cut once.”
- Test Thoroughly: Don’t just test the happy path. Consider edge cases and error conditions.
- Optimize Later: First make it work, then make it fast. Premature optimization is the root of all evil!
- Learn from Others: After completing a challenge, review other solutions to learn different approaches.
Ready to Challenge Yourself?
Remember, these challenges aren’t just about finding any solution – they’re about finding the best solution. Take your time, think critically, and most importantly, enjoy the learning process. After all, every expert Python developer started exactly where you are now.
Select your difficulty level above and let’s begin your journey to Python mastery!
Common Pitfalls and Solutions in Advanced Python Programming
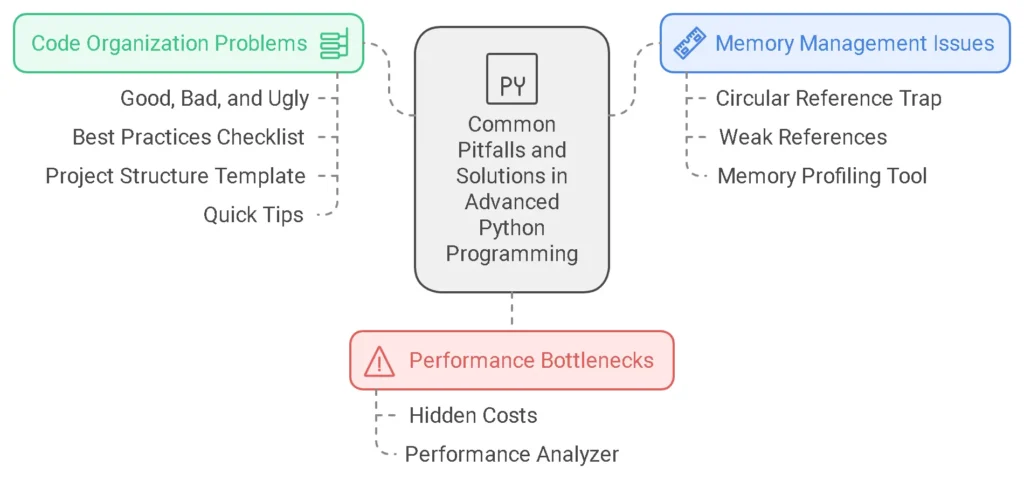
Let’s talk about something that every Python developer faces but few like to admit – those head-scratching moments when your seemingly perfect code isn’t behaving quite as perfectly as you’d hoped. I’ve been there more times than I can count, and I’m here to share the battle scars and lessons learned.
Memory Management Issues
Memory management in Python might seem hands-off thanks to the garbage collector, but that’s exactly what makes it tricky. Let’s dive into the most common memory pitfalls and their solutions.
The Circular Reference Trap
Consider this seemingly innocent code:
class Parent:
def __init__(self):
self.children = []
class Child:
def __init__(self, parent):
self.parent = parent
parent.children.append(self)
Looks harmless, right? But here’s what happens in memory:
Object Type | Reference Count | Memory Impact |
---|---|---|
Parent | 1 (self) + 1 (children’s reference) | Cannot be collected |
Child | 1 (self) + 1 (parent’s reference) | Cannot be collected |
Solution: Weak References
from weakref import WeakRef
class Child:
def __init__(self, parent):
self.parent = WeakRef(parent)
parent.children.append(self)
Memory Profiling Tool
Here’s a simple memory usage tracker you can use in your projects:
import tracemalloc
import functools
def track_memory(func):
@functools.wraps(func)
def wrapper(*args, **kwargs):
tracemalloc.start()
result = func(*args, **kwargs)
current, peak = tracemalloc.get_traced_memory()
print(f"Current memory usage: {current / 10**6}MB")
print(f"Peak memory usage: {peak / 10**6}MB")
tracemalloc.stop()
return result
return wrapper
Performance Bottlenecks
I once spent three days optimizing a data processing script only to find out the biggest bottleneck was something surprisingly simple. Let’s look at common performance issues and their solutions.
The Hidden Costs
Operation | Time Complexity | Hidden Cost | Better Alternative |
---|---|---|---|
list.insert(0, item) | O(n) | Full list copy | collections.deque |
dict.keys() in loop | O(1) | Creates view object | Direct key iteration |
str += str | O(n) | Creates new string | str.join() or io.StringIO |
Performance Analyzer
Code Organization Problems
Ever opened a Python file you wrote six months ago and wondered, “What was I thinking?” Yeah, me too. Let’s fix that.
The Good, Bad, and Ugly of Code Organization
Best Practices Checklist
- ✓ Use type hints for better code readability and IDE support
- ✓ Write docstrings following the Google or NumPy style guide
- ✓ Break large functions into smaller, focused ones
- ✓ Use meaningful variable names that explain their purpose
- ✓ Implement proper error handling with custom exceptions
- ✓ Follow the Single Responsibility Principle
Project Structure Template
project_name/
│
├── src/
│ ├── __init__.py
│ ├── core/
│ │ ├── __init__.py
| │ └── main.py
│ ├── utils/
│ │ ├── __init__.py
│ │ └── helpers.py
│ └── config/
│ ├── __init__.py
│ └── settings.py
│
├── tests/
│ ├── __init__.py
│ ├── test_core.py
│ └── test_utils.py
│
├── docs/
│ ├── README.md
│ └── API.md
│
└── setup.py
Quick Tips for Avoiding Common Pitfalls
- Memory Management
- Profile memory usage regularly
- Use context managers for resource cleanup
- Implement __slots__ for classes with fixed attributes
- Be careful with large list comprehensions
- Performance Optimization
- Use built-in functions whenever possible
- Leverage generator expressions for large datasets
- Profile before optimizing
- Consider using NumPy for numerical computations
- Code Organization
- Follow PEP 8 guidelines
- Use abstract base classes for interfaces
- Implement proper logging
- Write tests before fixing bugs
Remember, the goal isn’t to write perfect code – it’s to write code that’s maintainable, efficient, and clear in its intent. Start by implementing these solutions gradually, and you’ll see your codebase improve over time.
Advanced Python Libraries and Tools: Your Secret Weapons for Complex Programming Challenges
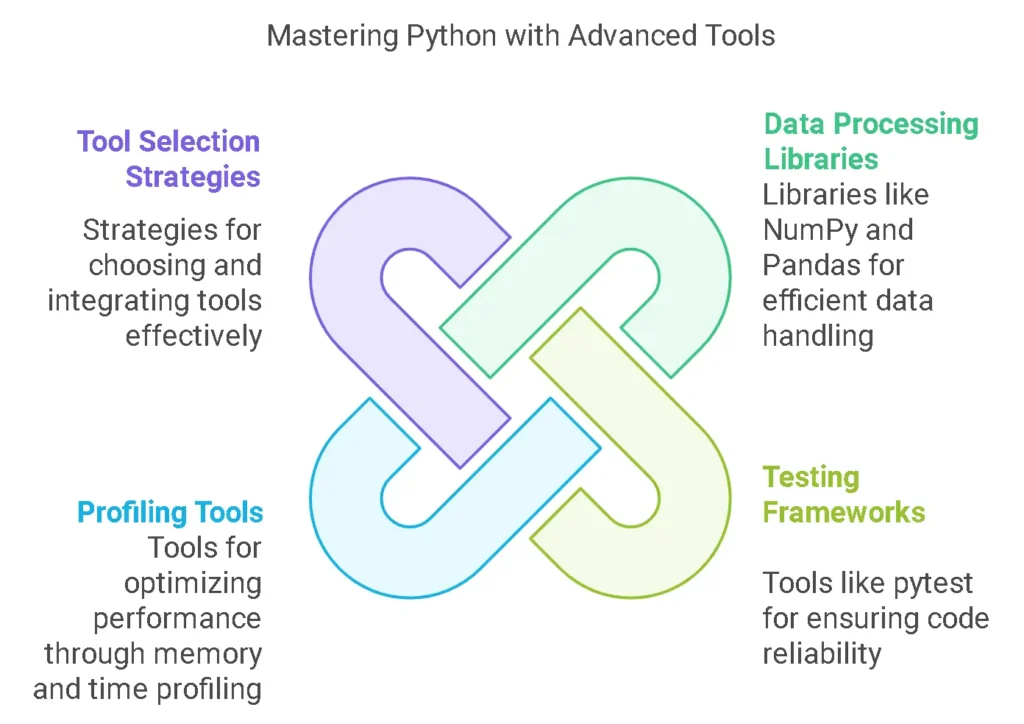
You know what separates a good Python solution from a great one? Often, it’s not just about the code you write – it’s about leveraging the right tools and libraries. I learned this lesson the hard way when I spent three days writing a complex data processing algorithm, only to discover that NumPy could have done it in three lines of code. Let’s make sure you don’t make the same mistake.
Essential Libraries for Complex Problems
Let’s dive into the powerhouse libraries that can transform how you tackle advanced programming challenges:
Data Processing and Scientific Computing
Library | Best For | When to Use | Performance Impact |
NumPy | Numerical operations & arrays | Large-scale numerical computations | 10-100x faster than pure Python |
Pandas | Data manipulation & analysis | Working with structured data | Optimal for datasets < 100GB |
SciPy | Scientific computations | Complex mathematical operations | Highly optimized for scientific computing |
# Example: Efficient matrix operations with NumPy
import numpy as np
def optimize_matrix_calculations(data):
# Instead of nested loops (slow):
# for i in range(len(matrix)):
# for j in range(len(matrix[0])):
# Use NumPy's vectorized operations (fast):
return np.dot(data, data.T)
Advanced String Processing and Text Analysis
Text Processing Powerhouses
- re (Regular Expressions)
- Pattern matching and text extraction
- Advanced string manipulation
- Text validation and cleaning
- NLTK (Natural Language Toolkit)
- Text analysis and processing
- Sentiment analysis
- Language parsing
- spaCy
- Industrial-strength NLP
- Named entity recognition
- Dependency parsing
Concurrent Programming and Performance
# Example: Using asyncio for concurrent operations
import asyncio
async def process_data(data_chunk):
await asyncio.sleep(1) # Simulating I/O operation
return data_chunk * 2
async def main():
tasks = [process_data(i) for i in range(10)]
results = await asyncio.gather(*tasks)
return results
Testing Frameworks: Building Unbreakable Code
Let me share a pro tip: the difference between a junior and senior Python developer often lies in their testing approach. Here are the testing frameworks that will elevate your code quality:
Framework | Best For | Learning Curve | Key Features |
---|---|---|---|
pytest | Modern Python testing | Medium | Fixtures, parametrization, plugins |
unittest | Standard library testing | Low | Test cases, assertions, test suites |
doctest | Documentation testing | Very Low | Interactive examples, documentation validation |
Writing Better Tests:
# Example: Advanced pytest usage
import pytest
from your_module import complex_function
@pytest.mark.parametrize("input,expected", [
([1, 2, 3], 6),
([-1, -2, -3], -6),
([0, 0, 0], 0),
])
def test_complex_function(input, expected):
assert complex_function(input) == expected
Profiling Tools: Optimizing Performance
Remember my earlier story about the slow code? Here’s how you can avoid similar situations using Python’s profiling tools:
Memory Profiling
# Using memory_profiler
from memory_profiler import profile
@profile
def memory_intensive_function():
# Your code here
pass
Time Profiling
cProfile
python -m cProfile -s cumtime your_script.py
line_profiler
@profile def your_function(): # Code to profile pass
Performance Monitoring Dashboard
Here’s a simple performance monitoring tool you can use:
Memory Usage
CPU Time
Response Time
Pro Tips for Tool Selection
- Start Small: Don’t try to implement every tool at once. Begin with the essentials:
- pytest for testing
- cProfile for basic profiling
- One specialized library for your specific need
- Scale Up: As your project grows, gradually introduce more sophisticated tools:
- Memory profilers for optimization
- Specialized testing frameworks
- Advanced debugging tools
- Maintain Balance: Remember, tools should help, not hinder. If you spend more time configuring tools than solving problems, reassess your toolchain.
Common Pitfalls to Avoid
- Don’t overengineer your testing setup
- Avoid premature optimization
- Be cautious with mixing too many different libraries
- Keep your dependencies manageable
Learning Resources
For deep dives into these tools, check out:
Remember, tools are only as good as the developer using them. Take time to understand each tool’s strengths and limitations, and you’ll be well-equipped to tackle any advanced Python programming challenge that comes your way.
Building Your Own Advanced Python Projects
You’ve mastered the syntax, conquered the algorithms, and now it’s time for the real test – building substantial projects that showcase your advanced Python skills. As someone who’s guided numerous developers through this journey, I can tell you that personal projects are where theory transforms into practical expertise.
Choosing Your Challenge
Let me share a secret that took me years to learn: the best advanced Python projects aren’t necessarily the most complex ones – they’re the ones that solve real problems while pushing your technical boundaries. Here are some project ideas that will genuinely test your abilities:
Distributed Task Processing System
Skill Focus: Concurrent programming, system design, queue management
# Example architecture snippet
from celery import Celery
from redis import Redis
app = Celery('tasks', broker='redis://localhost:6379/0')
cache = Redis(host='localhost', port=6379)
@app.task
def process_data(dataset_id):
# Complex data processing logic here
pass
Custom Web Framework
Skill Focus: WSGI, routing, middleware, decorators
# Basic routing implementation
class LightFramework:
def __init__(self):
self.routes = {}
def route(self, path):
def decorator(handler):
self.routes[path] = handler
return handler
return decorator
Advanced Data Analysis Pipeline
Skill Focus: Data structures, generators, memory optimization
❌ Memory-Intensive Approach
def process_large_dataset(file_path):
data = []
with open(file_path) as f:
for line in f:
data.append(process_line(line))
return data
✅ Memory-Efficient Approach
def process_large_dataset(file_path):
with open(file_path) as f:
for line in f:
yield process_line(line)
Project Best Practices Checklist
Here’s a comprehensive checklist I’ve developed over years of project management:
Category | Best Practice | Implementation Example |
---|---|---|
Code Structure | Use modular architecture | Create separate modules for data processing, API handling, and utilities |
Testing | Implement comprehensive tests | Unit tests, integration tests, and performance benchmarks |
Documentation | Write clear documentation | README.md, API docs, and inline comments for complex logic |
Version Control | Use meaningful commits | Feature branches and semantic versioning |
Performance | Profile and optimize | Use cProfile and memory_profiler for optimization |
Development Workflow
I’ve found that following a structured workflow is crucial for advanced projects. Here’s the workflow I use and recommend:
Planning Phase
- Define project scope and objectives
- Create technical specification document
- Break down into manageable components
- Set up project infrastructure
Development Phase
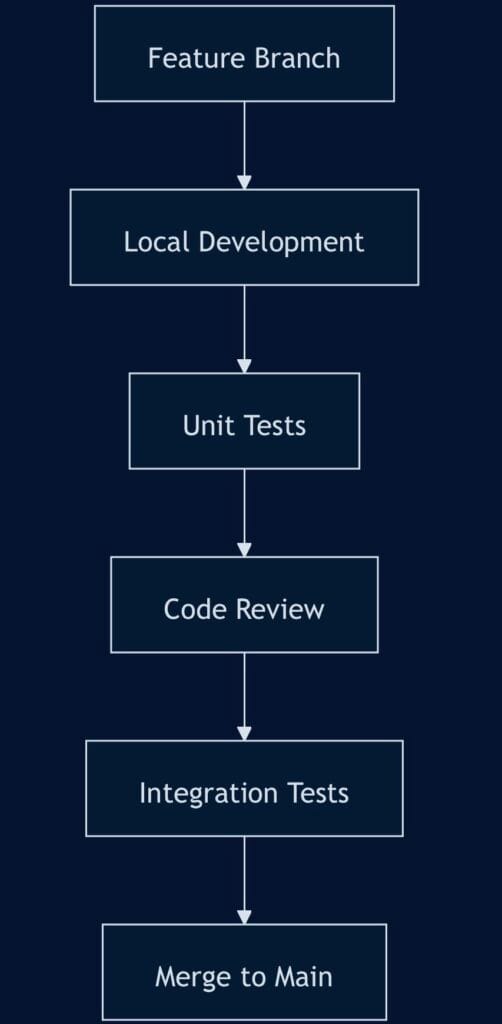
Testing Strategy
Unit Testing:
import pytest
from your_module import ComplexDataProcessor
def test_data_processor_handling():
processor = ComplexDataProcessor()
result = processor.process_complex_data({'key': 'value'})
assert result.is_valid()
assert result.performance_metric < 100
Performance Monitoring
Track these key metrics:
- Execution time
- Memory usage
- CPU utilization
- I/O operations
Here’s a simple performance monitoring decorator:
import time
import functools
def performance_monitor(func):
@functools.wraps(func)
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"{func.__name__} took {end_time - start_time:.2f} seconds")
return result
return wrapper
Advanced Project Ideas Showcase
ML Model Deployment Pipeline
Build an end-to-end ML model deployment system with monitoring and A/B testing capabilities.
Key Challenges:- Model versioning
- Real-time inference
- Performance monitoring
Distributed Cache System
Create a distributed caching system with consistency guarantees and failure handling.
Key Challenges:- Consistency protocols
- Network partitioning
- Cache invalidation
Real-time Analytics Engine
Develop a system for processing and analyzing streaming data in real-time.
Key Challenges:- Stream processing
- Data aggregation
- State management
Tips for Success
- Start Small, Scale Up
- Begin with core functionality
- Add features incrementally
- Refactor as needed
- Focus on Code Quality
- Use type hints
- Write comprehensive tests
- Document as you go
- Learn from Feedback
- Share your code with the community
- Accept and incorporate feedback
- Iterate based on real usage
Remember, the goal isn’t just to build something that works – it’s to build something that works well, is maintainable, and demonstrates your advanced Python skills. Each project should push your boundaries and teach you something new.
Performance Comparison of Different Approaches
Distributed Cache System
Create a distributed caching system with consistency guarantees and failure handling.
Key Challenges:- Consistency protocols
- Network partitioning
- Cache invalidation
Real-time Analytics Engine
Develop a system for processing and analyzing streaming data in real-time.
Key Challenges:- Stream processing
- Data aggregation
- State management
Start with these project ideas and best practices, but don’t be afraid to innovate and experiment. The best projects often come from solving real problems you’ve encountered in your own programming journey.
Community and Resources: Your Path to Python Mastery
You know what they say – it takes a village to raise a programmer. I learned this the hard way when I spent three days struggling with a metaclass implementation before a kind soul on Python’s Discord channel solved my problem in five minutes. Let’s explore the best communities and resources that can supercharge your advanced Python journey.
Online Platforms for Practice
I’ve tested dozens of coding platforms, and here are the ones that truly stand out for advanced Python practice:
Platform | Best For | Challenge Difficulty | Key Features |
---|---|---|---|
LeetCode | Interview Prep | Company-specific questions, detailed solutions, active discussion forums | |
Project Euler | Mathematical Programming | Complex mathematical problems, progression-based learning | |
HackerRank | Skill Certification | Industry recognition, structured learning paths | |
Codewars | Creative Problem-Solving | Community-created challenges, multiple solutions comparison |
Advanced Python Communities
Finding the right community can accelerate your learning exponentially. Here are the most valuable communities I’ve personally benefited from:
Online Forums and Chat Platforms
Python Discord
Members: 300K+
Real-time help, code reviews, and project collaboration. The #advanced-python channel is particularly valuable for complex discussions.
r/Python
Members: 1M+
Latest Python news, project showcases, and in-depth technical discussions. Check out the weekly advanced Python threads.
Stack Overflow
Active Python Tags: 1.5M+
Comprehensive Q&A database. Filter by [python-advanced] tag for high-level discussions.
Local and Virtual Meetups
- Python User Groups (PUGs)
- Find your local group on Meetup.com
- Regular workshops and coding sessions
- Networking with experienced developers
- PyLadies
- Inclusive community focusing on Python education
- Mentorship opportunities
- Technical workshops and conferences
- Virtual Python Conferences
- PyCon (Annual conference with recorded sessions)
- EuroPython
- PyData (Focus on data science and analytics)
Essential Reading Materials
Here’s my curated list of resources that have significantly improved my Python expertise:
Books That Matter
Fluent Python, 2nd Edition
Python Cookbook, 3rd Edition
High Performance Python
Online Resources and Documentation
- Official Documentation
- Advanced Python Blogs
- Interactive Learning Platforms
Pro Tips for Making the Most of Resources
- Set Clear Learning Goals
- Define specific areas you want to improve
- Create a structured learning plan
- Track your progress regularly
- Engage Actively
- Contribute to open-source projects
- Share your knowledge through blog posts or tutorials
- Participate in code reviews
- Practice Deliberately
- Focus on challenging problems
- Implement what you learn immediately
- Review and refactor your old code
Remember, the Python community is incredibly welcoming and supportive. Don’t hesitate to ask questions or share your knowledge. After all, today’s learner is tomorrow’s mentor.
The Future of Python Programming: Trends and Opportunities
Let me paint you a picture of where Python is headed. As someone who’s been in the trenches of Python development for years, I’ve watched this language evolve from a simple scripting tool to a powerhouse of modern computing. But what really excites me is what’s coming next.
Emerging Trends in Python Development
The Python ecosystem is evolving faster than ever, and staying ahead of these trends isn’t just about being cool – it’s about staying relevant in an ever-changing tech landscape.
Machine Learning Integration
Python continues to dominate AI/ML development with new frameworks and tools emerging regularly
Cloud-Native Development
Increased focus on serverless architectures and containerization solutions
Web Assembly Integration
Python’s growing presence in browser-based applications through WASM
Key Areas of Growth
- Quantum Computing
- Integration with quantum frameworks
- Quantum algorithm development
- Simulation and modeling capabilities
- Edge Computing
- Lightweight Python implementations
- IoT device programming
- Real-time processing optimization
- Big Data Processing
- Enhanced parallel processing capabilities
- Improved memory management
- Advanced data pipeline tools
Exciting New Python Features
The Python language itself is undergoing significant improvements. Here’s what’s making waves in the Python community:
Feature | Impact | Use Case |
---|---|---|
Pattern Matching | Simplified complex conditionals | Data parsing and validation |
Type Hints Evolution | Enhanced code reliability | Large-scale applications |
Async Improvements | Better performance | Web services and APIs |
Performance Improvements
Python’s performance is getting a serious boost through initiatives like:
- Faster CPython: The core implementation is being optimized
- Memory Management: More efficient garbage collection
- JIT Compilation: Enhanced just-in-time compilation capabilities
Career Opportunities in Python
Here’s something exciting: the demand for Python developers isn’t just growing – it’s evolving into new, specialized roles. Let’s look at where the opportunities are:
Python Developer Salaries by Specialization
Emerging Job Roles
- AI/ML Engineer
- Focus on machine learning model development
- Neural network architecture design
- Model optimization and deployment
- Cloud Architecture Specialist
- Serverless application development
- Microservices architecture
- Cloud-native Python applications
- Data Engineering Professional
- Big data pipeline development
- ETL process optimization
- Data warehouse architecture
Skills to Focus On
To stay competitive in the evolving Python landscape, focus on:
Technical Skills
- Advanced async programming
- Machine learning frameworks
- Cloud service integration
Soft Skills
- System design thinking
- Performance optimization
- Architecture planning
Looking Ahead
The future of Python programming is incredibly bright. As we see the language and its ecosystem continue to evolve, the opportunities for advanced Python developers will only grow. The key is to stay curious, keep learning, and most importantly, keep challenging yourself with advanced programming problems.
Remember, the most successful Python developers aren’t just those who know the syntax – they’re the ones who understand how to apply Python’s capabilities to solve real-world problems effectively. As you continue your journey with advanced Python programming challenges, you’re not just improving your coding skills; you’re preparing yourself for the future of technology.
Want to stay updated with Python’s evolution? Check out the Python Enhancement Proposals (PEPs) to see what’s coming next.
Mastering Advanced Python: Your Journey Continues
You’ve made it through our deep dive into advanced Python programming challenges. But remember what I said at the beginning? This isn’t just about writing complex code – it’s about transforming how you approach programming problems. Let’s wrap up with the essential takeaways and chart your path forward.
Key Takeaways: Your Advanced Python Toolkit
📌 Critical Insights for Advanced Python Development
Problem-Solving Mindset
- Break complex challenges into manageable components
- Consider performance implications from the start
- Always test edge cases in your solutions
Technical Mastery
- Optimize algorithms before optimizing code
- Leverage Python’s built-in features effectively
- Balance readability with performance
Essential Skills Checklist
Skill Area | Mastery Indicators |
---|---|
Algorithm Design | Can optimize time/space complexity effectively |
Data Structures | Implements custom structures for specific needs |
Problem-Solving | Approaches challenges systematically and efficiently |
Code Quality | Writes maintainable, documented, and tested code |
Your Next Steps: Continuous Learning Path
1. Practice Deliberately
Don’t just code – code with purpose. Here’s your weekly practice plan:
Wednesday: Data structure implementations
Friday: Real-world project work
Weekend: Code review and optimization
2. Build Your Portfolio
Start with these project ideas:
- Create a high-performance web scraper
- Build a custom data structure library
- Develop a machine learning pipeline
- Design a concurrent programming framework
3. Connect with the Community
The Python community is one of our greatest resources. Here’s how to engage:
Ready to Take Action?
Here’s your immediate action plan:
- Today: Choose one advanced Python challenge from our guide and solve it
- This Week: Join one of the recommended community platforms
- This Month: Complete a portfolio project using advanced concepts
- This Quarter: Contribute to an open-source Python project
Remember, becoming an advanced Python programmer isn’t a destination – it’s a journey of continuous learning and improvement. The challenges you’ve learned about are just the beginning. Keep pushing your boundaries, stay curious, and never stop exploring the amazing possibilities that Python offers.
I’d love to hear about your journey with these advanced Python challenges. Drop a comment below sharing your favorite challenge or your biggest takeaway from this guide. Let’s learn and grow together in this incredible Python community.
Happy coding! 🐍✨
Frequently Asked Questions About Advanced Python Programming
Getting Started with Advanced Python
The biggest challenge in advanced Python programming is mastering concurrency and parallel programming concepts. Unlike basic Python where programs run sequentially, advanced applications often require:
- Understanding thread synchronization
- Managing shared resources
- Handling race conditions
- Implementing asyncio effectively
Difficulty Level: Advanced (85/100)
Preparing for advanced Python coding challenges requires a structured approach:
1. Master the Fundamentals
Ensure solid understanding of Python basics, data structures, and algorithms
2. Practice Problem Solving
Regular practice on platforms like LeetCode, HackerRank, and CodeWars
3. Study Advanced Concepts
Focus on decorators, generators, metaclasses, and concurrency
4. Build Projects
Apply knowledge to real-world projects
Technical Challenges and Solutions
Error Type | Common Cause | Solution |
---|---|---|
Memory Leaks | Circular References | Use Weak References |
Race Conditions | Improper Thread Synchronization | Use Locks and Semaphores |
Deadlocks | Multiple Thread Resource Conflicts | Implement Proper Lock Ordering |
The most challenging aspects of Python programming include:
Career and Professional Development
Yes, Python is consistently one of the highest-paying programming skills. Here’s a breakdown of average salaries for different Python roles:
Role | Experience Level | Average Salary (USD) |
---|---|---|
Python Developer | Entry Level | $75,000 |
Senior Python Developer | 5+ Years | $120,000 |
ML Engineer (Python) | 3+ Years | $135,000 |
Lead Python Architect | 8+ Years | $150,000+ |
Learning and Growth
The journey to becoming an advanced Python programmer typically follows this timeline:
0-6 Months
Basics and Fundamentals
6-12 Months
Intermediate Concepts and Simple Projects
1-2 Years
Advanced Concepts and Complex Projects
2+ Years
Expert Level and Specialization
Note: This timeline assumes consistent practice and real-world application of skills.
4 thoughts on “Advanced Python Programming Challenges: Level Up Your Coding Skills”