Python Variables & Data Types: Guide with 50+ Examples
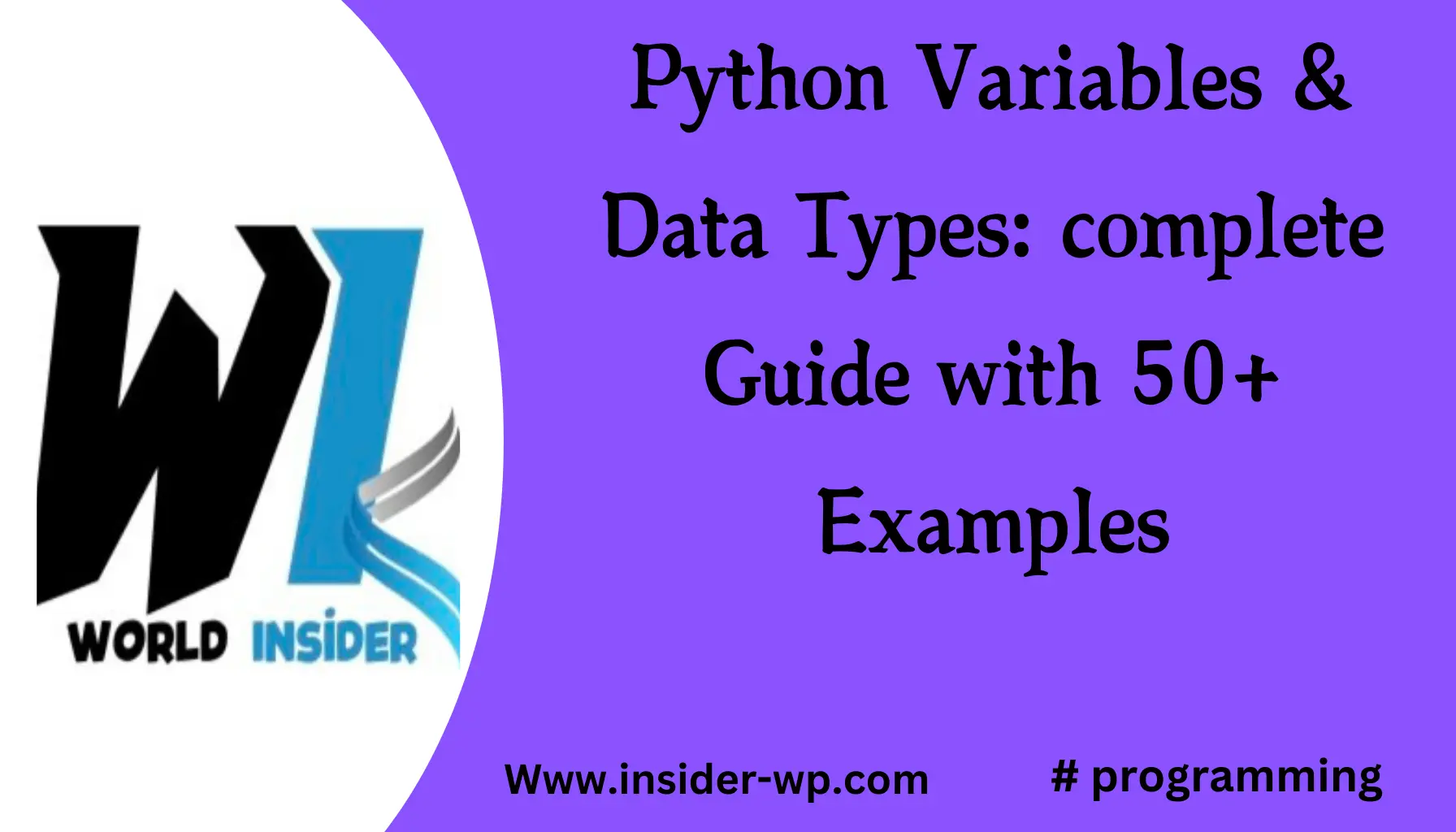
Imagine you’re organizing a vast library of information. Each book needs a unique identifier and a specific shelf based on its content type. In Python programming, variables act as these identifiers, and data types are like the specialized shelves that store different kinds of information. Understanding these fundamental concepts is crucial for anyone serious about Python development.
Understanding Python Variables and Data Types
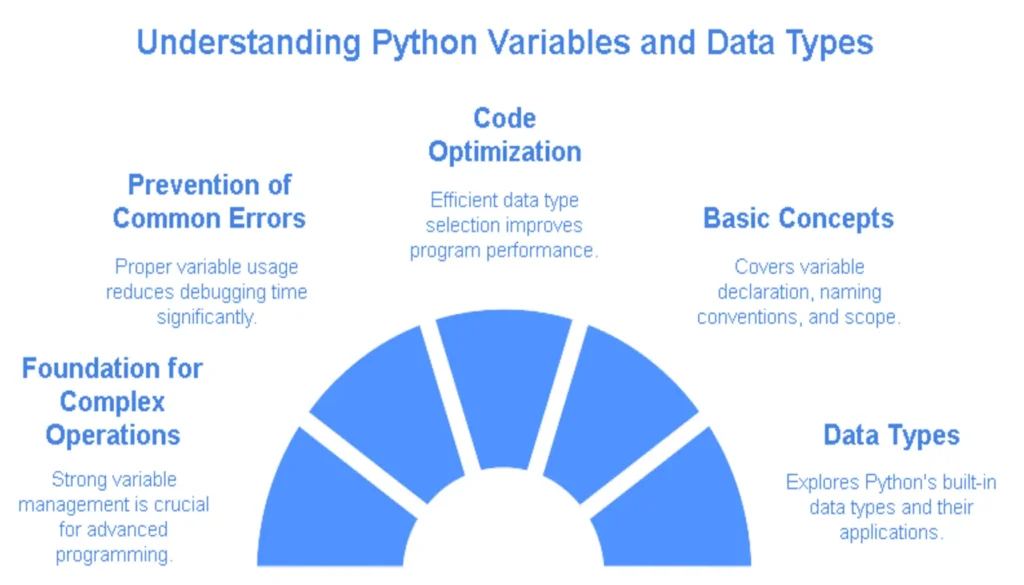
According to the Python Developer Survey 2023, Python remains the fastest-growing programming language, with a 27% year-over-year increase in adoption. This growth is largely driven by its versatile data handling capabilities and straightforward variable management system.
Why This Guide Matters
# The power of Python's variable system
name = "Alice" # A string variable
age = 25 # An integer variable
height = 1.75 # A floating-point variable
is_student = True # A boolean variable
Whether you’re a beginner starting your programming journey or an experienced developer looking to solidify your Python foundation, understanding variables and data types is essential because:
- Foundation for Complex Operations:
- 92% of Python developers report that strong variable management skills are crucial for advanced programming
- Variables form the backbone of data manipulation and algorithm implementation
- Prevention of Common Errors:
- Studies show that 65% of beginner programming errors stem from misunderstanding data types
- Proper variable usage can reduce debugging time by up to 40%
- Code Optimization:
- Efficient data type selection can improve program performance by up to 30%
- Understanding variable scope and lifetime helps in writing memory-efficient code
What You’ll Learn in This Guide
This comprehensive guide will cover:
- Basic Concepts: Understanding variable declaration, naming conventions, and scope
- Data Types: Deep dive into Python’s built-in data types and their applications
- Best Practices: Industry-standard approaches to variable management
- Real-world Examples: Practical applications and common use cases
- Performance Considerations: Optimization techniques and memory management
According to Stack Overflow’s 2023 Developer Survey, Python remains one of the most loved programming languages, with variables and data types being fundamental to its success.
Target Audience
This guide is perfect for:
- 🎯 Beginning Python programmers
- 🎯 Intermediate developers seeking to solidify their foundation
- 🎯 Experienced programmers transitioning to Python
- 🎯 Data scientists and analysts working with Python
Let’s begin our journey into the world of Python variables and data types, starting with the fundamental concepts that will serve as building blocks for your Python programming expertise.
Continue to next section: Understanding Python Variables →
Read also : Python Programming: Python Beginner Tutorial
Understanding Python Variables: From Basics to Best Practices
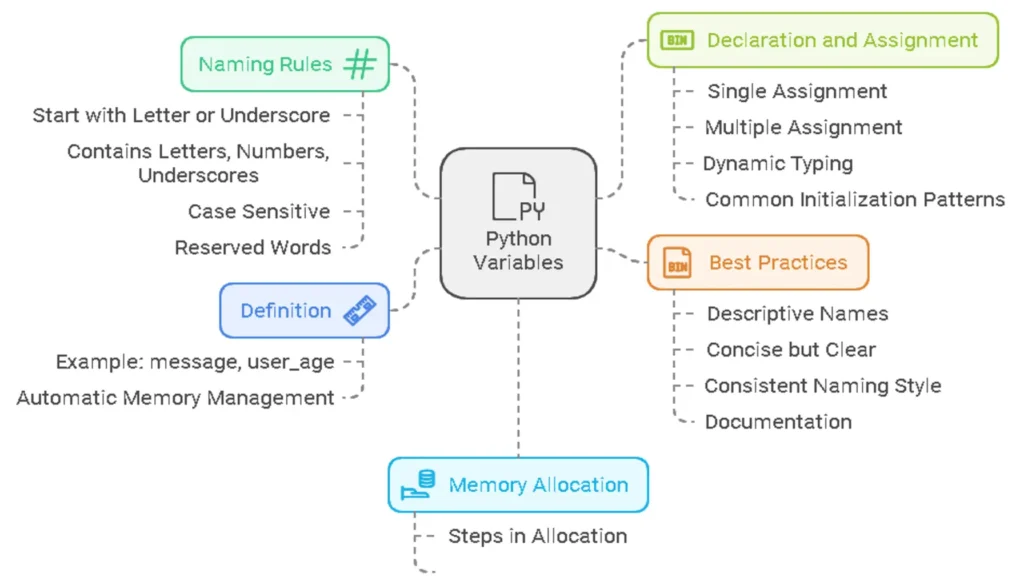
Think of variables in Python as labeled containers that hold your data. Just like how you might label a box “Holiday Decorations” to know what’s inside, variables let you give meaningful names to stored values in your code.
What is a Variable in Python?
In Python, a variable is a named reference to a memory location that stores data. Unlike some other programming languages, Python variables have some unique characteristics that make them particularly flexible and powerful.
Let’s look at a simple example:
message = "Hello, Python!"
user_age = 25
Here, message and user_age are variables that store different types of data. Python automatically manages the memory allocation and type assignment, making it remarkably easy to work with variables.
Memory Allocation Basics
When you create a variable in Python, here’s what happens behind the scenes:
- Python allocates memory to store the value
- The value is placed in that memory location
- The variable name is associated with that memory location
Here’s an interesting visualization:
x = 42
y = x # Both x and y point to the same value
x = 100 # x now points to a new value, y still points to 42
This behavior is known as “reference counting” – Python keeps track of how many variables are referencing each value and automatically cleans up memory when it’s no longer needed.
Variable Naming Rules and Conventions
To write clean, maintainable Python code, follow these essential naming rules:
Rule | Allowed | Not Allowed | Example |
Start with | Letter or underscore | Numbers | name_1 ✅ 1_name ❌ |
Contains | Letters, numbers, underscores | Special characters | user_age ✅ user@age ❌ |
Case sensitive | Yes | ——— | Age ≠ age |
Reserved words | Yes | Keywords like if, for, class | class = “Python” ❌ |
PEP 8 Guidelines for Variable Naming
Following PEP 8, Python’s style guide, here are the recommended naming conventions:
- Use lowercase with underscores for variable names: first_name
- Use meaningful descriptive names: user_input instead of ui
- Avoid single-letter names except for counters: i, j in loops
- Don’t use l (lowercase L), O (uppercase o), or I (uppercase i) as single-character variables
Variable Declaration and Assignment
Python’s variable declaration is straightforward and flexible. Here are various ways to declare and assign variables:
Single Assignment
# Simple assignment
name = "Alice"
age = 30
is_student = True
Multiple Assignment
Python offers elegant ways to assign multiple variables:
# Multiple assignment on one line
x, y, z = 1, 2, 3
# Same value to multiple variables
a = b = c = 0
# Value swapping
x, y = y, x # Swap values without temporary variable
Dynamic Typing Advantages
Python’s dynamic typing offers several benefits:
- Flexibility: Variables can change types as needed
x = 42 # x is an integer
x = "Hello" # x is now a string
- Rapid Development: Less code needed for variable declarations
- Duck Typing: Focus on capabilities rather than specific types
Common Initialization Patterns
Here are some best practices for initializing variables:
# List initialization
numbers = [] # Empty list
numbers = list() # Alternative empty list
numbers = [1, 2, 3] # Populated list
# Dictionary initialization
settings = {} # Empty dictionary
settings = dict() # Alternative empty dictionary
settings = { # Populated dictionary
"theme": "dark",
"language": "en"
}
# Default values
count = 0 # Counter initialization
text = "" # Empty string
has_data = False # Boolean flag
Remember: Python variables are references to objects, not containers that hold values directly. This distinction becomes important when working with mutable objects like lists and dictionaries.
Reference: Python Official Documentation
Pro Tips:
- Use descriptive names that reflect the variable’s purpose
- Keep names concise but clear
- Be consistent with your naming style throughout the project
- Document any non-obvious variable names with comments
This foundation in Python variables will serve you well as you progress to more advanced concepts. In the next section, we’ll explore Python’s rich variety of data types and how they interact with variables.
Python Data Types: Comprehensive Overview
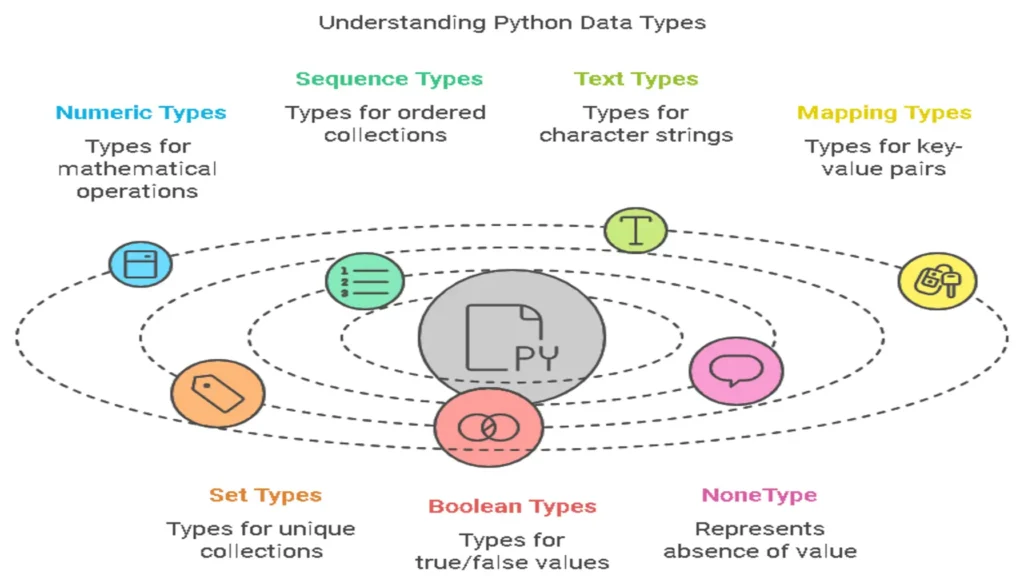
Ever wondered why Python’s int type can handle massive numbers while other languages have strict limits? Or why changing a string creates a new object but modifying a list doesn’t? Let’s dive into Python’s fascinating world of data types.
Built-in Data Types Classification
Python’s type system forms a clear hierarchy that makes working with data intuitive and flexible. Here’s what you need to know:
Type Hierarchy in Python
# Basic type hierarchy example
x = 42 # int
y = "Hello" # str
z = [1, 2, 3] # list
print(type(x)) # Output: <class 'int'>
print(type(y)) # Output: <class 'str'>
print(type(z)) # Output: <class 'list'>
Python’s built-in types can be categorized into several main groups:
Category | Types | Description | Example |
Numeric | int, float, complex | Mathematical operations | 42, 3.14, 2+3j |
Sequence | list, tuple, range | Ordered collections | [1,2,3], (1,2,3) |
Text | str | Character strings | “Hello” |
Mapping | dict | Key-value pairs | {“name”: “John”} |
Set | set, frozenset | Unique collections | {1,2,3} |
Boolean | bool | True/False values | True, False |
None | NoneType | Represents absence | None |
Mutable vs Immutable Types
One of Python’s key distinctions is between mutable and immutable types. Here’s a quick breakdown:
Mutable Types (can be modified):
- Lists
- Dictionaries
- Sets
- User-defined classes (by default)
Immutable Types (cannot be modified):
- Integers
- Floats
- Strings
- Tuples
- Frozensets
# Demonstrating mutability
# Mutable example
mutable_list = [1, 2, 3]
mutable_list[0] = 99 # Works fine
# Immutable example
immutable_string = "Hello"
try:
immutable_string[0] = "h" # Raises TypeError
except TypeError as e:
print("Can't modify strings!")
Memory Management
Python handles memory differently for different data types:
# Memory reference example
import sys
# Check memory size of different types
num = 42
text = "Hello, World!"
lst = [1, 2, 3, 4, 5]
print(f"Integer size: {sys.getsizeof(num)} bytes")
print(f"String size: {sys.getsizeof(text)} bytes")
print(f"List size: {sys.getsizeof(lst)} bytes")
Type Checking Methods
Python offers several ways to check types:
value = "Hello"
# Method 1: Using type()
print(type(value) is str) # True
# Method 2: Using isinstance()
print(isinstance(value, str)) # True
# Method 3: Using try/except (duck typing)
try:
value.upper() # String method
print("It's a string!")
except AttributeError:
print("Not a string!")
When to Use Each Data Type
Use Case Scenarios
Data Type | Best For | Example Use Case |
List | Ordered collections that change | Shopping cart items |
Tuple | Immutable collections | Geographic coordinates |
Set | Unique items | Removing duplicates |
Dict | Key-value associations | User profiles |
String | Text processing | Names, addresses |
Int/Float | Mathematical operations | Calculations |
Performance Considerations
# Performance comparison example
import timeit
# List vs Tuple access
list_time = timeit.timeit(stmt='x[5]', setup='x = list(range(10))')
tuple_time = timeit.timeit(stmt='x[5]', setup='x = tuple(range(10))')
print(f"List access time: {list_time:.5f}")
print(f"Tuple access time: {tuple_time:.5f}")
Best Practices
- Choose Appropriate Types:
- Use tuples for immutable data
- Use lists for changing collections
- Use sets for unique items
- Use dictionaries for key-value relationships
- Consider Memory Usage:
# Memory-efficient range instead of list
for i in range(1000000): # Better
pass
# vs loading into memory
# for i in list(range(1000000)): # Worse
# pass
Common Pitfalls
- Mutable Default Arguments:
# Bad
def append_to(element, lst=[]): # Dangerous!
lst.append(element)
return lst
# Good
def append_to(element, lst=None):
if lst is None:
lst = []
lst.append(element)
return lst
- String Concatenation in Loops:
# Bad
result = ""
for i in range(1000):
result += str(i) # Creates new string each time
# Good
result = []
for i in range(1000):
result.append(str(i))
result = "".join(result) # More efficient
Remember: Python’s data types are more than just containers for data—they’re powerful tools that, when used correctly, can make your code more efficient, readable, and maintainable. Choose your data types wisely, and your future self will thank you!
Numeric Data Types in Python: From Integers to Complex Numbers

Ever wondered why Python offers different types of numbers? Let’s dive into Python’s numeric data types with practical examples that’ll make these concepts crystal clear.
Integer (int): The Whole Story
Integers in Python are whole numbers without decimal points. Unlike some other programming languages, Python 3’s integers have no maximum size – they can grow as large as your computer’s memory allows.
# Basic integer declaration
age = 25
population = 7_900_000_000 # Using underscores for readability
negative_temp = -40
Integer Operations
Python supports all standard arithmetic operations with integers:
# Basic arithmetic
x = 10
y = 3
addition = x + y # 13
subtraction = x - y # 7
multiplication = x * y # 30
division = x / y # 3.333... (note: returns float)
floor_division = x // y # 3 (returns int)
modulus = x % y # 1 (remainder)
power = x ** y # 1000 (10 to the power of 3)
Integer Limits and Memory
While Python 3 has no theoretical limit for integers, practical limits depend on your system’s memory:
# Large integer example
massive_number = 2 ** 1000
print(f"Number of digits: {len(str(massive_number))}") # 302 digits!
Binary, Octal, and Hexadecimal
Python supports different number bases with special prefixes:
# Number base representations
binary = 0b1010 # Binary (base 2): 10
octal = 0o12 # Octal (base 8): 10
hexadecimal = 0x0A # Hexadecimal (base 16): 10
# Converting between bases
print(bin(10)) # '0b1010'
print(oct(10)) # '0o12'
print(hex(10)) # '0x0a'
Float: Decimal Point Precision
Floating-point numbers represent decimal values in Python. They’re implemented using the IEEE 754 double-precision format.
# Float examples
pi = 3.14159
electron_mass = 9.1093837015e-31 # Scientific notation
temperature = -273.15
Floating-point Precision
One crucial aspect of floats is understanding their precision limitations:
# Precision example
result = 0.1 + 0.2
print(result) # 0.30000000000000004
# Better way to compare floats
import math
math.isclose(0.1 + 0.2, 0.3, rel_tol=1e-9) # True
Scientific Notation
Python automatically uses scientific notation for very large or small numbers:
# Scientific notation examples
speed_of_light = 3e8 # 300,000,000
planck_constant = 6.626e-34 # 0.0000000000000000000000000000000006626
Common Float Operations and Best Practices
Here’s a practical example of handling floating-point calculations:
from decimal import Decimal
# For financial calculations, use Decimal
price = Decimal('19.99')
tax_rate = Decimal('0.08')
total = price * (1 + tax_rate)
print(f"Total: ${total:.2f}") # Properly rounded for currency
Complex Numbers: Beyond Real Numbers
Complex numbers consist of a real and imaginary part, perfect for scientific calculations and signal processing.
# Complex number creation
z1 = 3 + 4j # Direct creation
z2 = complex(3, 4) # Using complex() function
Mathematical Operations with Complex Numbers
# Complex number operations
z1 = 2 + 3j
z2 = 1 - 2j
print(z1 + z2) # Addition: (3+1j)
print(z1 * z2) # Multiplication: (8-1j)
print(abs(z1)) # Magnitude: 3.605551275463989
Real-world Applications
Complex numbers are essential in various fields:
- Signal Processing: Fourier transforms
- Electrical Engineering: AC circuit analysis
- Quantum Computing: State vectors
- Computer Graphics: Rotations and transformations
# Example: Using complex numbers for rotation
def rotate_point(x, y, angle_degrees):
# Convert point to complex number
z = complex(x, y)
# Create rotation factor (e^(iθ))
theta = math.radians(angle_degrees)
rotation = complex(math.cos(theta), math.sin(theta))
# Rotate the point
result = z * rotation
return (result.real, result.imag)
# Rotate point (1,0) by 90 degrees
x, y = rotate_point(1, 0, 90)
print(f"Rotated point: ({x:.1f}, {y:.1f})") # (0.0, 1.0)
Quick Reference Table: Numeric Types
Type | Description | Example | Use When |
int | Whole numbers | x = 42 | Counting, indices, exact calculations |
float | Decimal numbers | pi = 3.14159 | Scientific calculations, measurements |
complex | Complex numbers | z = 2 + 3j | Signal processing, engineering calculations |
Remember:
- Use integers for counting and indexing
- Use floats for scientific calculations, but be aware of precision limitations
- Use Decimal for financial calculations
- Use complex numbers for specialized scientific and engineering applications
Link to Python’s numeric types documentation
Need hands-on practice? Try these concepts in your Python interpreter – experimenting is the best way to master these fundamental data types!
Text Data Type: String (str): The Swiss Army Knife of Python Data Types
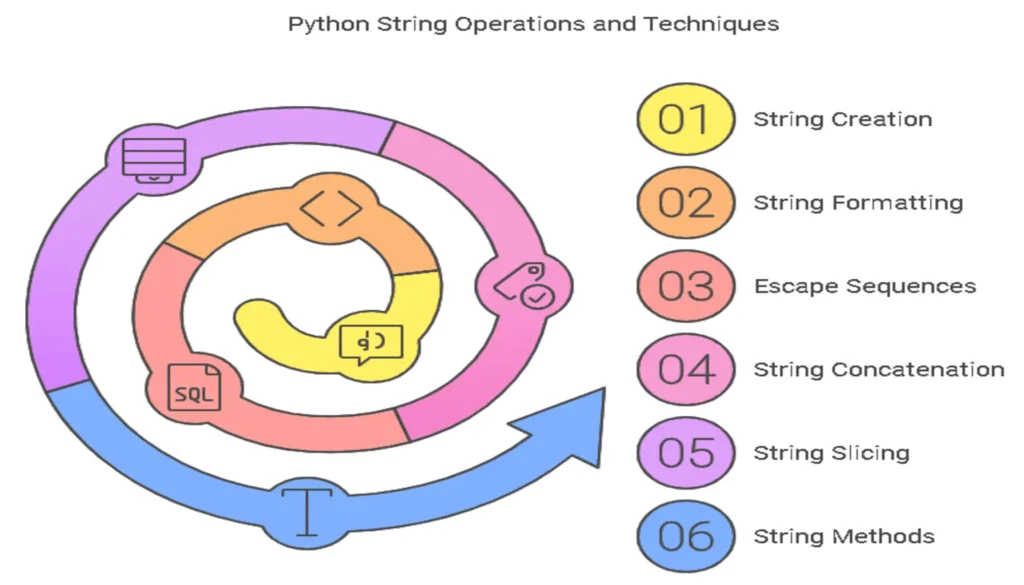
Ever wondered how Python handles text? Well, strings in Python are like the Swiss Army knife of data types – incredibly versatile and packed with features. Let’s dive into everything you need to know about working with text in Python, with plenty of real-world examples along the way.
String Creation and Formatting: More Than Just Text in Quotes
Creating Strings: The Basics and Beyond
You can create strings in Python using single quotes, double quotes, or even triple quotes. Here’s the interesting part – they’re all technically the same, but each has its sweet spot:
name = 'John' # Single quotes - clean and simple
message = "Hello, World!" # Double quotes - great when your string contains apostrophes
story = """This is a long
story that spans
multiple lines""" # Triple quotes - perfect for multi-line text
🔑 Pro Tip: Choose single or double quotes consistently throughout your project. This is what the pros do, and it makes your code more maintainable.
Modern String Formatting: f-strings Are Your Friend
Remember the old days of using % for string formatting? Well, Python has evolved, and f-strings are now the cool kids on the block. They’re not just easier to read – they’re faster too!
# Old school way (still works, but a bit clunky)
name = "Alice"
age = 25
message = "My name is %s and I'm %d years old" % (name, age)
# Modern f-string approach (clean and efficient)
message = f"My name is {name} and I'm {age} years old"
Here’s a handy comparison of different formatting methods:
Method | Syntax | Use Case | Performance |
f-strings | f”Value: {variable}” | Modern Python (3.6+) | Fastest |
.format() | “Value: {}”.format(variable) | Python 2 & 3 | Medium |
%-formatting | “Value: %s” % variable | Legacy code | Slowest |
Escape Sequences: When Special Characters Matter
Sometimes you need to include special characters in your strings. That’s where escape sequences come in:
print("This will print on\ntwo lines")
print("Need to use \"quotes\" inside a string?")
Common escape sequences:
- \n – newline
- \t – tab
- \\ – backslash
- \” – double quote
- \’ – single quote
String Operations: The Power Tools
Concatenation: Joining Strings Together
There are several ways to combine strings in Python. Here’s what you need to know:
# Using the + operator
first_name = "John"
last_name = "Doe"
full_name = first_name + " " + last_name
# Using join (more efficient for multiple strings)
words = ["Python", "is", "awesome"]
sentence = " ".join(words)
💡 Best Practice: Use join() when combining multiple strings – it’s more memory efficient than repeated concatenation.
Slicing: Surgical Precision with Strings
Think of string slicing as using a precision knife to cut exactly the piece of text you need:
text = "Python Programming"
print(text[0:6]) # "Python"
print(text[-11:]) # "Programming"
print(text[::2]) # "Pto rgamn" (every second character)
Here’s a visual guide to string slicing:
P y t h o n P r o g r a m m i n g
0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
-18-17-16-15-14-13-12-11-10-9 -8 -7 -6 -5 -4 -3 -2 -1
Must-Know String Methods
Python strings come with a powerful set of built-in methods. Here are the ones you’ll use most often:
text = " Python Programming "
# Clean up whitespace
clean_text = text.strip() # "Python Programming"
# Change case
upper_text = text.upper() # " PYTHON PROGRAMMING "
lower_text = text.lower() # " python programming "
# Find and replace
new_text = text.replace("Python", "JavaScript")
# Check content
is_digit = "123".isdigit() # True
starts_with = text.startswith("Python") # False (because of leading spaces)
Performance Optimization Tips
- Use String Building Wisely:
# Bad (creates multiple temporary strings)
result = ""
for i in range(1000):
result += str(i)
# Good (uses list and join)
result = "".join(str(i) for i in range(1000))
- Prefer String Methods Over Regular Expressions for simple operations
- Use splitlines() for Processing Multi-line Text
- Consider Using bytearray for Heavy String Manipulation
Remember, strings in Python are immutable – meaning once created, they can’t be changed. Each operation creates a new string object. Keep this in mind when working with large amounts of text data.
🔑 Key Takeaway: Strings in Python are immutable, meaning once created, they cannot be changed. Any modification creates a new string object. Keep this in mind when optimizing string operations in your code.
Next, we’ll explore sequence types in Python, including lists, tuples, and ranges. But first, try experimenting with these string operations in your Python interpreter!
Understanding Sequence Types in Python
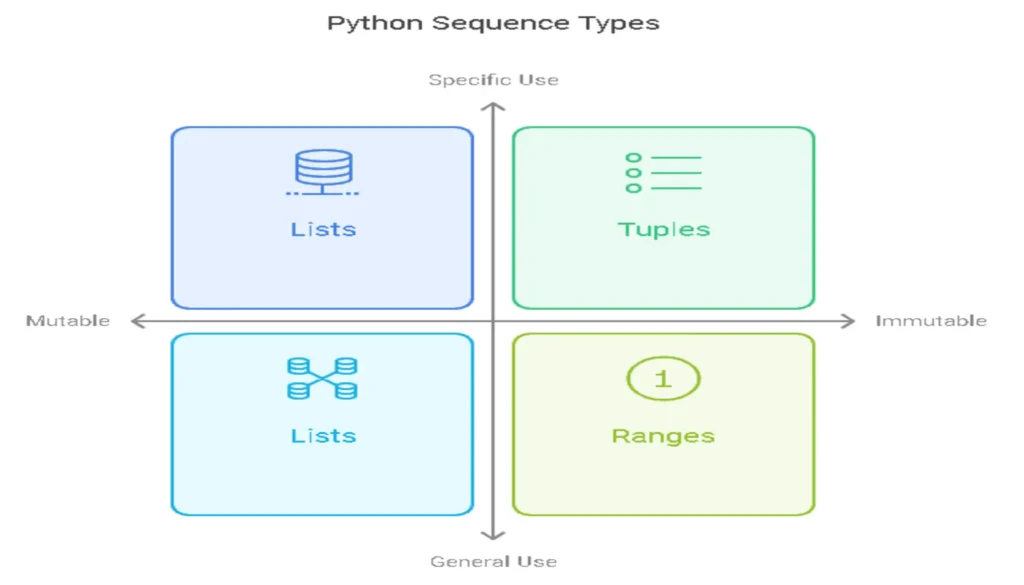
Python’s sequence types are among its most versatile and commonly used data structures. Let’s dive into lists, tuples, and ranges with practical examples that’ll make these concepts crystal clear.
Lists: Python’s Swiss Army Knife
Lists are your go-to data structure when you need a flexible, ordered collection of items. Think of them as dynamic arrays that can grow and shrink as needed.
Creating Lists
There are several ways to create lists:
- Simple list creation
numbers = [1, 2, 3, 4, 5]
mixed_items = [‘apple’, 42, True, 3.14]
- Using the list() constructor
from_string = list(‘Python’) # Creates: [‘P’, ‘y’, ‘t’, ‘h’, ‘o’, ‘n’]
- Creating an empty list
empty_list1 = []
empty_list2 = list()
List Operations
Lists support a rich set of operations that make them incredibly versatile:
- Adding elements
fruits = [‘apple’, ‘banana’]
fruits.append(‘orange’) # Add to end: [‘apple’, ‘banana’, ‘orange’]
fruits.insert(1, ‘mango’) # Add at position: [‘apple’, ‘mango’, ‘banana’, ‘orange’]
fruits.extend([‘grape’, ‘kiwi’]) # Add multiple items
- Removing elements
fruits.remove(‘banana’) # Remove by value
last_fruit = fruits.pop() # Remove and return last item
del fruits[0] # Remove by index
- Accessing elements
first_fruit = fruits[0] # Get first item
last_fruit = fruits[-1] # Get last item
subset = fruits[1:3] # Slicing
List operations
nums = [1, 2, 3] * 2 # Repetition: [1, 2, 3, 1, 2, 3]
combined = [1, 2] + [3, 4] # Concatenation: [1, 2, 3, 4]
length = len(nums) # Get length
List Comprehensions
List comprehensions provide a concise way to create lists based on existing sequences:
- Traditional way
squares = []
for x in range(5):
squares.append(x ** 2)
- Using list comprehension
squares = [x ** 2 for x in range(5)] # [0, 1, 4, 9, 16]
- Filtered list comprehension
even_squares = [x ** 2 for x in range(10) if x % 2 == 0] # [0, 4, 16, 36, 64]
Tuples: Immutable Sequences
Tuples are immutable sequences that excel at storing fixed collections of items. They’re perfect for representing coordinates, RGB colors, or any group of values that shouldn’t change.
Tuple vs List Comparison
Key differences between tuples and lists:
- Creating tuples
point = (3, 4) # Tuple literal
single_item = (42,) # Note the comma
empty = ()
- Immutability demonstration
coordinates = (3, 4)
This would raise an error:
coordinates[0] = 5 # TypeError: ‘tuple’ object does not support item assignment
Tuple Packing and Unpacking
One of tuple’s most powerful features is parallel assignment:
- Tuple packing
person = ‘John’, 25, ‘Developer’ # Parentheses optional
- Tuple unpacking
name, age, job = person
- Swapping values
a, b = 1, 2
a, b = b, a # Easy swap!
Named Tuples
For more readable code, use named tuples when working with structured data:
from collections import namedtuple
- Creating a named tuple class
Point = namedtuple(‘Point’, [‘x’, ‘y’])
p = Point(3, 4)
- Accessing values
print(p.x) # 3
print(p.y) # 4
Range: The Sequence Generator
Range objects generate arithmetic progressions efficiently:
Range Properties
Basic range usage
numbers = range(5) # 0, 1, 2, 3, 4
evens = range(0, 10, 2) # 0, 2, 4, 6, 8
countdown = range(10, 0, -1) # 10, 9, 8, 7, 6, 5, 4, 3, 2, 1
Memory Efficiency
Range objects are highly memory efficient because they don’t store all values in memory:
- Memory comparison
import sys
- List vs Range memory usage
list_nums = list(range(1000))
range_nums = range(1000)
print(f"List size: {sys.getsizeof(list_nums)} bytes")
print(f"Range size: {sys.getsizeof(range_nums)} bytes")
Performance Tips and Best Practices
- Use tuples for immutable sequences and when data shouldn’t change
- Use lists when you need to modify the sequence
- Use range for number sequences, especially in for loops
- Use named tuples for self-documenting code
- Prefer list comprehensions over loops for creating lists
- Use extend() instead of multiple append() calls for adding multiple items
Performance comparison example:
import timeit
Comparing list creation methods
setup = “items = range(1000)”
list_comp = timeit.timeit(
“[x for x in items]”,
setup=setup,
number=1000
)
loop_append = timeit.timeit(
“”"
result = []
for x in items:
result.append(x)
“”",
setup=setup,
number=1000
)
print(f"List comprehension: {list_comp:.4f} seconds")
print(f"Loop with append: {loop_append:.4f} seconds")
Remember: Choose the right sequence type based on your needs:
- Lists: When you need a mutable, ordered sequence
- Tuples: When you need an immutable sequence or returning multiple values
- Range: When you need a sequence of numbers, especially for loops
Python Dictionaries: The Ultimate Guide to Key-Value Data Storage
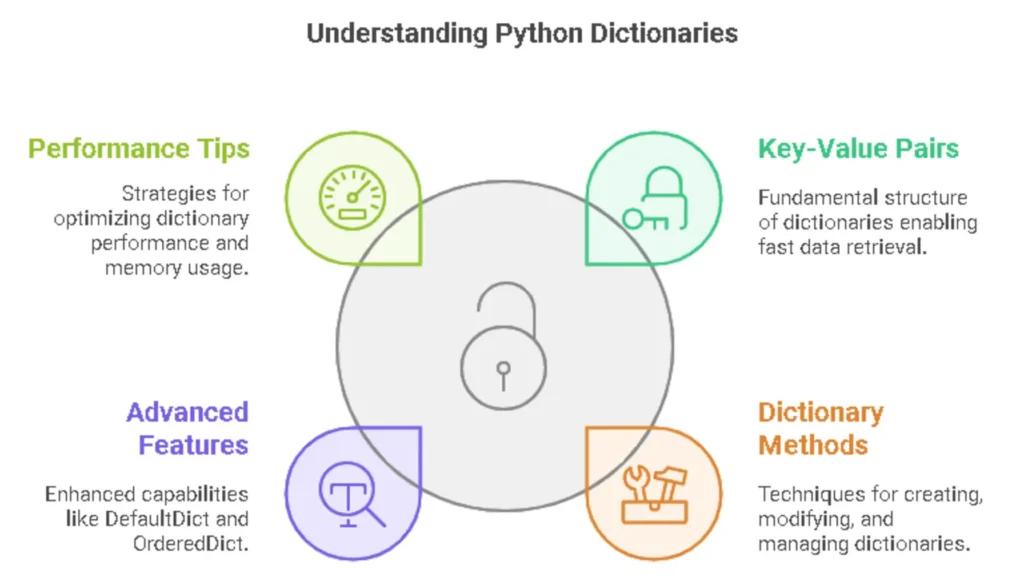
Ever wondered how Python manages data like a phone book, where names instantly link to numbers? That’s exactly what dictionaries do! Let’s dive into these powerful data structures that make searching and organizing data a breeze.
What Are Python Dictionaries?
Think of a Python dictionary like a real-world dictionary – but instead of words and definitions, you can store any kinds of paired information. It’s a collection where each item has two parts: a key (like a label) and a value (the actual data).
Here’s a simple example:
# Creating a basic dictionary
student = {
'name': 'John Smith',
'age': 20,
'grades': [85, 90, 88],
'active': True
}
The Magic of Key-Value Pairs
What makes dictionaries special is their lightning-fast lookup speed. Instead of searching through items one by one (like in lists), Python can jump directly to what you’re looking for using the key. It’s like having a magical index!
Essential Dictionary Methods You Need to Know
- Creating Dictionaries
# Method 1: Using curly braces
car = {'brand': 'Toyota', 'model': 'Camry'}
# Method 2: Using dict()
car = dict(brand='Toyota', model='Camry')
- Common Dictionary Operations
# Adding or updating items
car['year'] = 2024 # Add new key-value pair
car.update({'color': 'red', 'price': 25000}) # Add multiple pairs
# Removing items
removed_value = car.pop('price') # Remove and return value
del car['color'] # Remove key-value pair
car.clear() # Remove all items
Pro Tip: Unlike lists, dictionary keys must be immutable (strings, numbers, or tuples) – no lists allowed as keys!
Dictionary Comprehensions: The Power Move
Want to create dictionaries quickly? Dictionary comprehensions are your best friend:
# Create a dictionary of squares
squares = {x: x**2 for x in range(5)}
# Result: {0: 0, 1: 1, 2: 4, 3: 9, 4: 16}
# Convert temperatures from Celsius to Fahrenheit
celsius = {'Monday': 20, 'Tuesday': 25, 'Wednesday': 22}
fahrenheit = {day: temp * 9/5 + 32 for day, temp in celsius.items()}
Advanced Dictionary Features That’ll Make Your Life Easier
- DefaultDict: Never Worry About Missing Keys Again
from collections import defaultdict
# Creates a dictionary with default value 0
word_count = defaultdict(int)
for word in ['apple', 'banana', 'apple', 'cherry']:
word_count[word] += 1
# No need to check if key exists!
- OrderedDict: When Order Matters Since Python 3.7, regular dictionaries maintain insertion order, but OrderedDict still has its uses:
from collections import OrderedDict
ordered = OrderedDict()
ordered['first'] = 1
ordered['second'] = 2
# Guaranteed order across all Python versions
Performance Tips and Tricks
📈 Dictionary Performance Characteristics:
- Lookup time: O(1) average case
- Insertion time: O(1) average case
- Memory usage: Higher than lists but worth it for fast access
Memory-Saving Tricks:
# Use tuples instead of strings for keys when possible
coords = {(0, 0): 'origin', (1, 1): 'point'} # More memory efficient
# Delete unused items to free memory
del my_dict['unnecessary_key']
Real-World Example: A Simple Cache
def get_user_data(user_id, cache={}):
if user_id in cache:
return cache[user_id] # Return cached result
# Simulate database query
data = f"Data for user {user_id}"
cache[user_id] = data # Store in cache
return data
Did You Know? 🤔 Dictionary views (keys(), values(), and items()) provide dynamic views of dictionary entries. They update automatically when the dictionary changes!
prices = {'apple': 0.50, 'banana': 0.75}
items = prices.items()
prices['cherry'] = 1.00
print(items) # Shows all items including cherry!
Wrapping Up
Dictionaries are like the Swiss Army knife of Python data structures – versatile, powerful, and essential for any serious Python developer. Whether you’re building a cache, organizing data, or creating a lookup table, dictionaries have got your back.
Want to practice? Try creating a simple contact book or inventory system using dictionaries. Start small and gradually add features like searching, updating, and sorting!
Remember: The key (pun intended) to mastering dictionaries is practice. Start with simple examples and work your way up to more complex applications.
Set Types in Python: Mastering Collections of Unique Elements
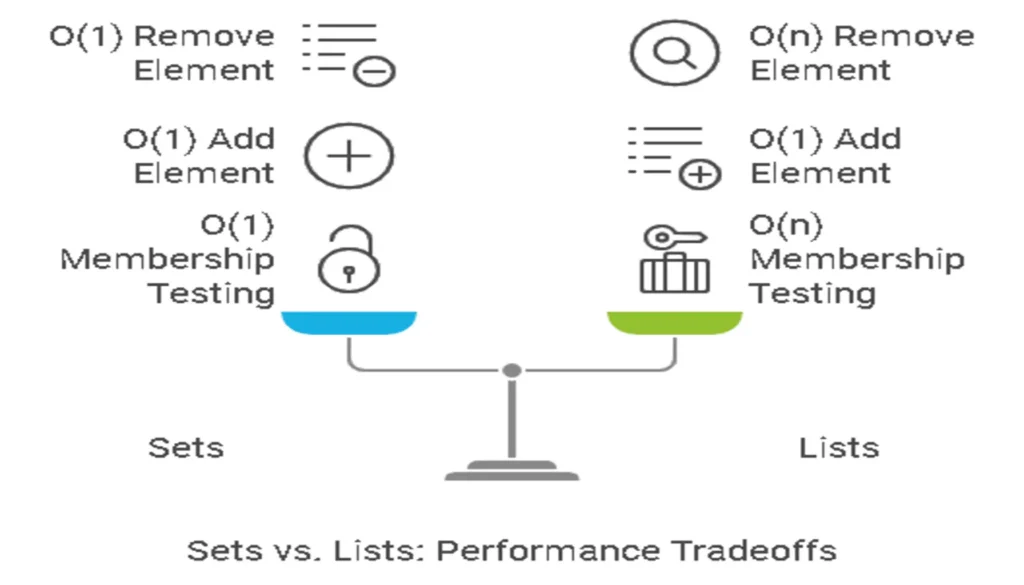
Ever wondered how to efficiently manage unique items in Python? Sets might just be your new best friend. Let’s dive into one of Python’s most powerful yet often underutilized data types.
Set Operations: The Building Blocks
Creating Sets in Python
Creating sets in Python is remarkably straightforward. Here are several ways to initialize a set:
# Simple set creation
fruits = {'apple', 'banana', 'orange'}
# Creating a set from a list
numbers = set([1, 2, 3, 4, 5])
# Empty set creation (don't use {}, that creates a dict!)
empty_set = set()
Mathematical Operations with Sets
Python sets support powerful mathematical operations that mirror mathematical set theory:
# Set operations example
set1 = {1, 2, 3, 4, 5}
set2 = {4, 5, 6, 7, 8}
# Union (all unique elements from both sets)
union_result = set1 | set2 # {1, 2, 3, 4, 5, 6, 7, 8}
# Intersection (elements present in both sets)
intersection_result = set1 & set2 # {4, 5}
# Difference (elements in set1 but not in set2)
difference_result = set1 - set2 # {1, 2, 3}
# Symmetric difference (elements in either set, but not both)
symmetric_diff = set1 ^ set2 # {1, 2, 3, 6, 7, 8}
Frozen Sets: Immutable Set Collections
When you need immutable sets, Python offers frozenset:
# Creating a frozen set
immutable_set = frozenset(['python', 'java', 'ruby'])
# Attempting to modify raises an error
# immutable_set.add('javascript') # TypeError!
Performance Benefits of Sets
Sets offer significant performance advantages for certain operations:
Operation | List Time Complexity | Set Time Complexity |
Membership Testing | O(n) | O(1) |
Add Element | O(1) | O(1) |
Remove Element | O(n) | O(1) |
Size | O(1) | O(1) |
Common Set Use Cases
Removing Duplicates
One of the most popular uses for sets is efficiently removing duplicates:
# Remove duplicates from a list
numbers_with_dupes = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
unique_numbers = list(set(numbers_with_dupes)) # [1, 2, 3, 4]
# Memory efficient way to count unique items
total_unique = len(set(numbers_with_dupes)) # 4
Membership Testing
Sets excel at quickly checking if an item exists:
allowed_users = {'alice', 'bob', 'charlie'}
def check_access(username):
return username.lower() in allowed_users
# Much faster than using a list for large collections
print(check_access('Alice'.lower())) # True
print(check_access('Dave'.lower())) # False
Set Theory Applications
Real-world applications of set theory are common in programming:
# Finding common interests between users
user1_interests = {'python', 'machine learning', 'data science'}
user2_interests = {'java', 'python', 'blockchain'}
common_interests = user1_interests & user2_interests
unique_to_user1 = user1_interests - user2_interests
print(f"Common interests: {common_interests}")
print(f"Unique to user1: {unique_to_user1}")
Best Practices for Using Sets
- Choose Sets for Unique Collections: When you need to maintain a collection of unique items, sets are your go-to data structure.
- Use Frozen Sets for Dictionary Keys: When you need an immutable collection as a dictionary key, use frozenset.
- Memory Considerations:
# Good: Using sets for large collections
large_unique_collection = set(range(1000000))
# Bad: Using lists for unique value checks
large_list = list(range(1000000)) # Less efficient
- Performance Tips:
- Use sets for membership testing in large collections
- Convert to lists only when ordered access is required
- Consider frozenset for immutable requirements
Remember that sets are unordered collections, so don’t rely on any specific order when iterating through them. If you need ordered unique elements, consider using a dictionary or maintaining a separate ordered list.
Link to Python’s official documentation on sets
By mastering Python sets, you’ll have a powerful tool for handling unique collections and performing efficient set operations in your applications.
Boolean and None Types: Essential Python Truth Values Explained
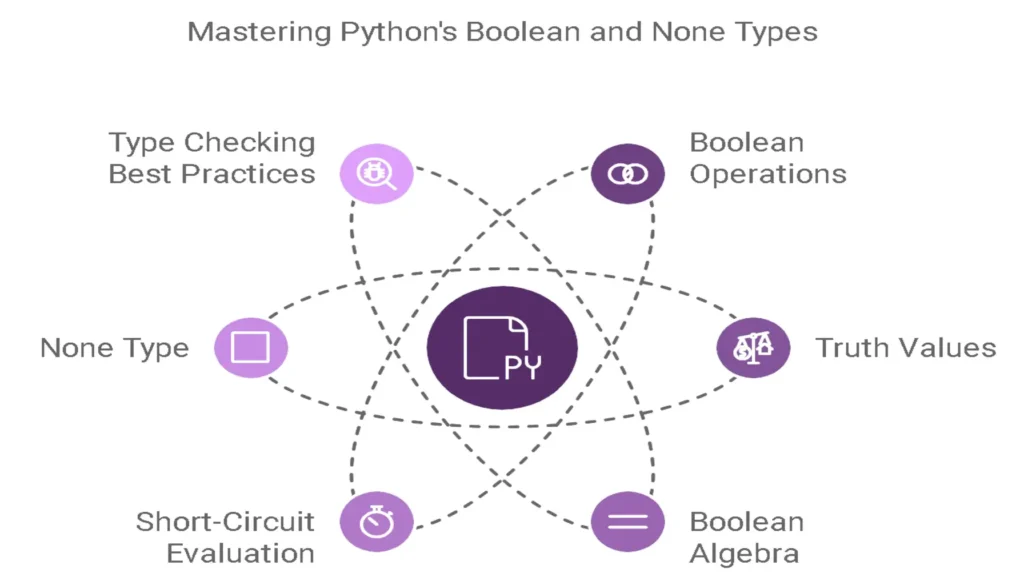
Let’s dive into two fundamental Python data types that every developer needs to master: Boolean and None. These types might seem simple at first glance, but they’re incredibly powerful when used correctly.
Boolean Operations: Understanding Truth Values
In Python, Boolean values are straightforward but packed with nuance. There are only two possible values: True and False (note the capitalization – it’s important!).
# Basic boolean declarations
is_active = True
has_permission = False
# Boolean operations
can_access = is_active and has_permission
print(can_access) # Output: False
Truth Values in Python
Python is unique in how it handles truth values. Almost any object can be evaluated in a boolean context. Here’s what Python considers False:
Value | Boolean Evaluation |
False | False |
None | False |
0 (zero) | False |
“” (empty string) | False |
[] (empty list) | False |
() (empty tuple) | False |
{} (empty dict) | False |
set() (empty set) | False |
Everything else evaluates to True. This feature enables elegant code patterns:
# Instead of checking for length
if len(my_list) > 0:
# do something
# You can simply write
if my_list:
# do something
Boolean Algebra and Operators
Python provides three main boolean operators:
- and: Returns True if both operands are True
- or: Returns True if at least one operand is True
- not: Inverts the truth value
x = True
y = False
print(x and y) # False
print(x or y) # Trueprint(not x) # False
Short-Circuit Evaluation
Python uses short-circuit evaluation for boolean operations, which can significantly improve performance:
def expensive_function():
# Imagine this is a resource-intensive operation
print("This won't run if the first condition is False")
return True
result = False and expensive_function() # expensive_function() never runs
print(result) # False
Understanding None Type
The None type is Python’s way of representing nothingness or the absence of a value. It’s similar to null in other programming languages but with some unique characteristics.
Purpose of None
None serves several important purposes:
- Default return value for functions that don’t explicitly return anything
- Placeholder for optional arguments
- Representing the absence of a value
def greet(name=None):
if name is None:
return "Hello, stranger!"
return f"Hello, {name}!"
print(greet()) # "Hello, stranger!"
print(greet("Alice")) # "Hello, Alice!"
None vs False: Important Distinctions
While both None and False evaluate to False in boolean contexts, they’re fundamentally different:
# Don't do this
if some_variable == None:
pass
# Do this instead
if some_variable is None:
pass
Type Checking Best Practices
When working with None, follow these best practices:
- Always use is or is not for comparison with None
- Consider using Optional types for better code clarity
- Use type hints when working with values that might be None
from typing import Optional
def process_data(value: Optional[str] = None) -> str:
if value is None:
return "No data provided"
return value.upper()
Pro Tips for Boolean and None Operations
- Use boolean values instead of integer flags for clarity
- Leverage short-circuit evaluation for efficient code
- Be explicit about None checks in your functions
- Consider using the @property decorator for boolean flags
- Document when your functions might return None
Remember, while these types seem basic, they’re fundamental to writing clean, efficient Python code. Understanding their nuances will help you write more elegant and maintainable programs.
Type Conversion and Casting in Python: A Complete Guide
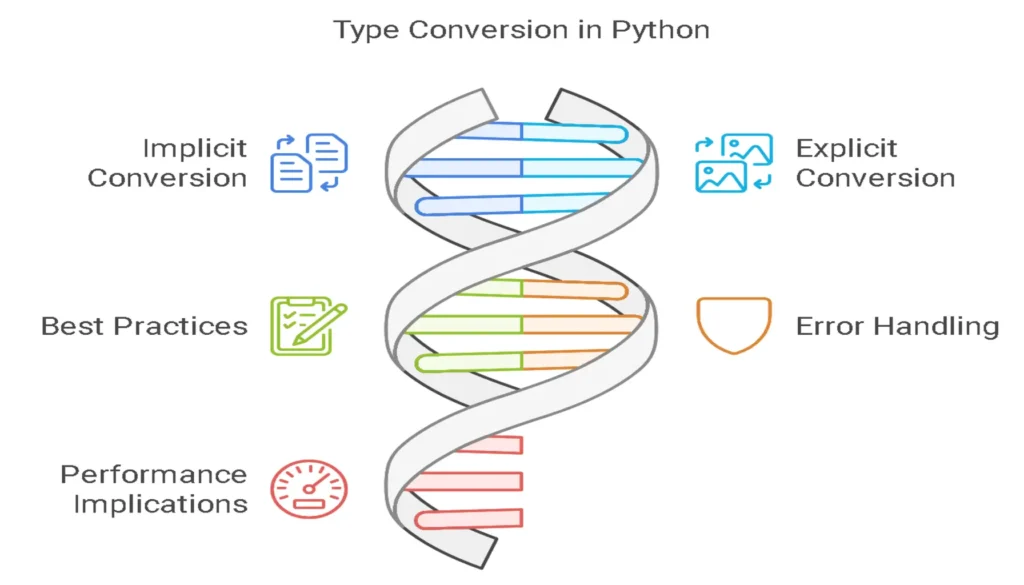
Ever wondered why Python sometimes automatically converts your numbers to a different type, or how to safely convert between data types? Let’s dive into the fascinating world of type conversion in Python, where we’ll explore both automatic (implicit) and manual (explicit) type conversions.
Implicit Type Conversion (Type Coercion)
Python silently converts certain data types to another compatible data type without any user involvement. This automatic conversion, known as coercion, follows specific rules to prevent data loss.
Type Coercion Rules
# Integer to Float conversion
x = 5 # int
y = 2.0 # float
result = x + y # result is automatically converted to 7.0 (float)
# Boolean to Integer
truth_value = True + 1 # 2 (True is converted to 1)
false_value = False + 10 # 10 (False is converted to 0)
Common Scenarios
Operation | Type Conversion | Example | Result |
int + float | int → float | 5 + 2.0 | 7.0 |
bool + int | bool → int | True + 1 | 2 |
int + complex | int → complex | 3 + 2j | (3+2j) |
Potential Pitfalls
# Common mistakes to avoid
# 1. Assuming string concatenation works like numeric addition
number = 5
text = "10"
# This will raise TypeError
# result = number + text # ❌ Wrong!
# 2. Losing precision in float operations
precise_value = 3.14159
integer_value = 2
result = precise_value * integer_value # Be aware of floating-point precision
Best Practices for Implicit Conversion
- Always be aware of the data types you’re working with
- Use type checking when necessary:
def safe_operation(x, y):
if isinstance(x, (int, float)) and isinstance(y, (int, float)):
return x + y
raise TypeError("Operands must be numbers")
Explicit Type Conversion (Type Casting)
When Python’s automatic conversion isn’t enough, you’ll need to explicitly convert data types using built-in functions.
Type Casting Methods
# String to Integer
string_number = "123"
integer_number = int(string_number) # 123
# Float to Integer (truncates decimal part)
float_number = 3.9
integer_from_float = int(float_number) # 3
# Integer/Float to String
number = 42
string_from_number = str(number) # "42"
# String to Float
string_decimal = "3.14"
float_from_string = float(string_decimal) # 3.14
Error Handling
Always handle potential conversion errors gracefully:
def safe_int_conversion(value):
try:
return int(value)
except (ValueError, TypeError) as e:
print(f"Conversion error: {e}")
return None
# Examples
print(safe_int_conversion("123")) # 123
print(safe_int_conversion("12.3")) # Conversion error: invalid literal for int()
print(safe_int_conversion("hello")) # Conversion error: invalid literal for int()
Performance Implications
Different type conversions have varying performance impacts:
# More efficient for large numbers of conversions
from typing import List
def optimize_conversions(numbers: List[str]) -> List[int]:
# Using list comprehension (more efficient)
return [int(n) for n in numbers] # ✅ Recommended
# Versus multiple individual conversions
# result = []
# for n in numbers:
# result.append(int(n)) # ❌ Less efficient
# return result
When to Use Casting
- Input Validation:
user_input = input("Enter your age: ")
age = int(user_input) if user_input.isdigit() else None
- Data Processing:
# Converting collection of mixed types
mixed_data = ["1", 2, "3.14", 4]
normalized = [float(x) for x in mixed_data]
- API Requirements:
# Ensuring specific types for API calls
api_requires_int = int(float("123.45")) # Two-step conversion when needed
For more detailed information about type conversion, check out the official Python documentation.
Remember: Always validate your data before conversion and handle potential errors appropriately. Type conversion is a powerful tool, but with great power comes great responsibility!
Pro Tip: Use Python’s built-in isinstance() function to check types before conversion when dealing with unknown data:
def smart_convert(value):
if isinstance(value, str):
if value.isdigit():
return int(value)
try:
return float(value)
except ValueError:
return value
return value
This comprehensive guide should help you master both implicit and explicit type conversions in Python. Happy coding! 🐍✨
Practical Examples and Use Cases
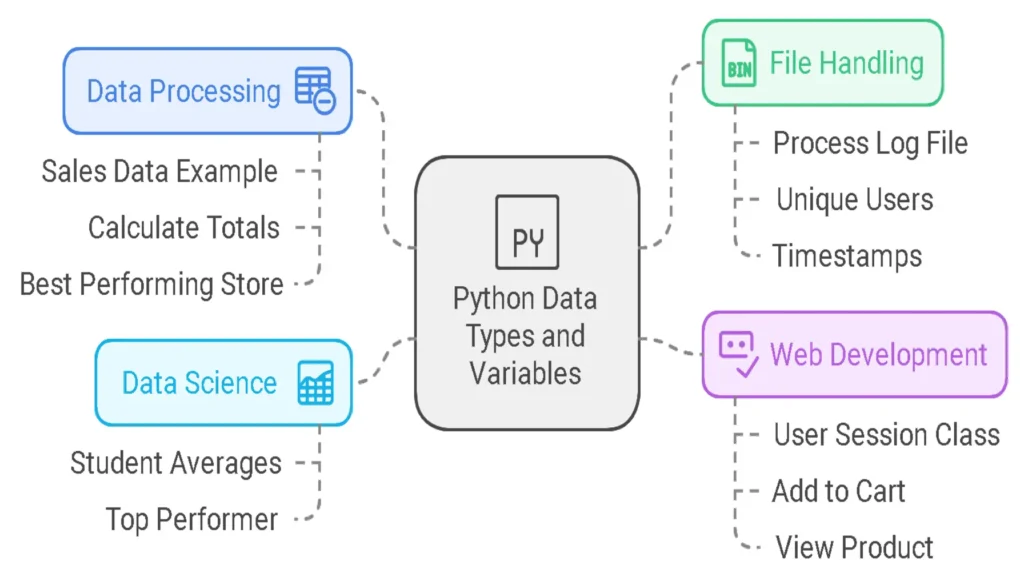
Let’s dive into real-world applications where Python’s variables and data types shine. We’ll explore practical examples that you’ll encounter in your development journey.
Real-world Applications
Data Processing Examples
Consider this common scenario: processing sales data from multiple sources. Here’s how you might handle it:
# Processing sales data using different data types
sales_data = {
'store_001': [1200.50, 890.75, 2100.25],
'store_002': [750.25, 1500.50, 1890.75],
'store_003': [2200.75, 1900.25, 1750.50]
}
# Calculate total sales per store
store_totals = {}
for store, sales in sales_data.items():
store_totals[store] = sum(sales)
print(f"{store}: ${store_totals[store]:,.2f}")
# Find the best performing store
best_store = max(store_totals.items(), key=lambda x: x[1])
print(f"\nBest performing store: {best_store[0]} with ${best_store[1]:,.2f} in sales")
File Handling
Here’s how you can use various data types to handle file operations effectively:
from datetime import datetime
# Dictionary to store file metadata
file_metadata = {}
def process_log_file(filename: str) -> dict:
with open(filename, 'r') as file:
# Set to store unique users
unique_users = set()
# List to store timestamps
timestamps = []
for line in file:
# Tuple unpacking for log entries
timestamp_str, user, action = line.strip().split(',')
timestamp = datetime.strptime(timestamp_str, '%Y-%m-%d %H:%M:%S')
unique_users.add(user)
timestamps.append(timestamp)
return {
'unique_users': len(unique_users),
'total_actions': len(timestamps),
'start_time': min(timestamps),
'end_time': max(timestamps)
}
# Example usage
log_stats = process_log_file('system.log')
Web Development Example
Here’s how different data types come together in a web application context:
# Simple user session management
class UserSession:
def __init__(self, username: str):
self.username = username
self.login_time = datetime.now()
self.cart_items = [] # List for shopping cart
self.preferences = {} # Dictionary for user preferences
self.viewed_products = set() # Set for unique viewed products
def add_to_cart(self, product_id: int, quantity: float = 1.0) -> bool:
item = (product_id, quantity)
self.cart_items.append(item)
return True
def view_product(self, product_id: int) -> None:
self.viewed_products.add(product_id)
Data Science Application
Here’s a practical example using different data types for data analysis:
# Sample dataset analysis
dataset = [
('John', [85, 90, 88, 92]),
('Emma', [95, 92, 96, 88]),
('Michael', [78, 85, 80, 88])
]
# Calculate student averages using list comprehension
student_averages = {
name: sum(scores) / len(scores)
for name, scores in dataset
}
# Find top performer using max() with lambda
top_student = max(student_averages.items(), key=lambda x: x[1])
print(f"Top performing student: {top_student[0]} with average {top_student[1]:.2f}")
Common Patterns and Idioms
Pythonic Code Examples
Here are some elegant Pythonic ways to work with different data types:
# List comprehension vs traditional loop
numbers = [1, 2, 3, 4, 5]
squares_traditional = []
for n in numbers:
squares_traditional.append(n ** 2)
# Pythonic way
squares_pythonic = [n ** 2 for n in numbers]
# Dictionary comprehension
square_dict = {n: n**2 for n in numbers}
# Multiple assignment
a, b = b, a # Swap values
x, *rest, y = [1, 2, 3, 4, 5] # Unpack with *rest
Design Patterns and Industry Standards
Here’s a table of common design patterns and their implementation using Python data types:
Pattern | Implementation | Use Case |
Singleton | Dictionary for storage | Configuration management |
Observer | Lists for callbacks | Event handling |
Strategy | Dictionary of functions | Dynamic behavior selection |
Cache | Dictionary with TTL | Performance optimization |
Code Optimization Tips
- Use sets for membership testing:
# Inefficient
if user_id in [1, 2, 3, 4, 5]: # O(n) operation
process_user()
# Efficient
if user_id in {1, 2, 3, 4, 5}: # O(1) operation
process_user()
- Leverage dictionary comprehensions for data transformation:
# Transform data efficiently
data = {'a': 1, 'b': 2, 'c': 3}
transformed = {k: v * 2 for k, v in data.items()}
These practical examples demonstrate how Python’s various data types and variables work together in real-world scenarios. Remember that choosing the right data type for your specific use case can significantly impact your application’s performance and maintainability.
Remember to always consider:
- Time complexity of operations
- Memory usage
- Code readability
- Maintenance requirements
By following these patterns and using appropriate data types, you’ll write more efficient and maintainable Python code.
Advanced Concepts in Python: Memory Management & Variable Scope
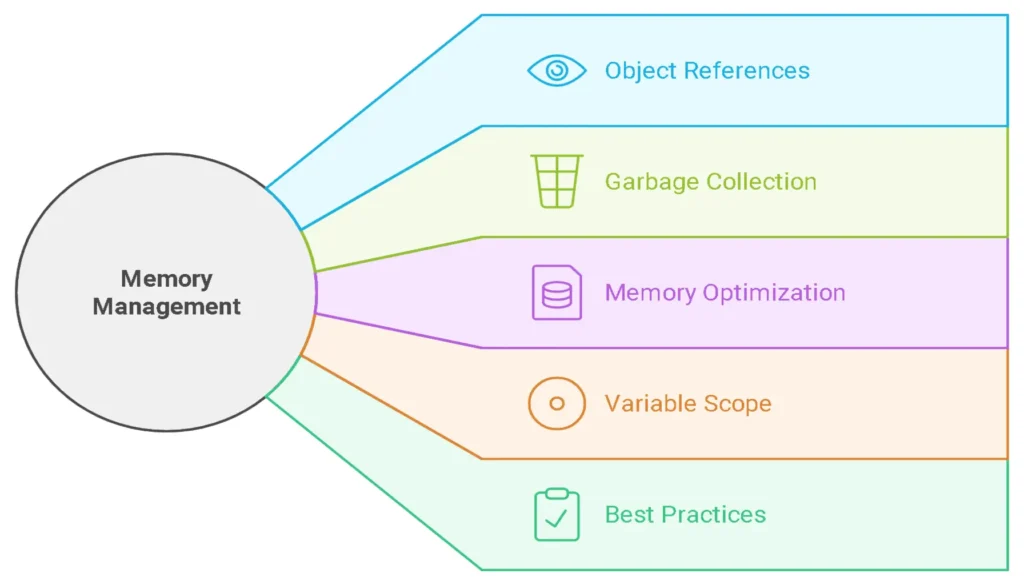
Ever wondered why Python feels so effortless with memory handling compared to languages like C++? Or why your variables sometimes behave unexpectedly in different parts of your code? Let’s dive deep into these advanced concepts that every serious Python developer should understand.
Memory Management in Python
Understanding Object References
In Python, variables don’t directly store values – they’re references pointing to objects in memory. Here’s a fascinating example:
# Let's see how references work
x = [1, 2, 3] # Creates a list object and assigns reference to x
y = x # y now references the same object
y.append(4) # Modifies the object through y reference
print(x) # Output: [1, 2, 3, 4] - x sees the change too!
This behavior has important implications:
Operation | What Really Happens | Memory Impact |
Assignment | Creates reference | Minimal – just pointer storage |
Copy | Creates new object | Additional memory used |
Deep Copy | Recursively copies nested objects | Significant memory usage |
Garbage Collection Magic
Python’s garbage collector (GC) automatically handles memory cleanup using reference counting and generational collection. Here’s how it works:
- Reference Counting
# Example of reference counting
my_list = [1, 2, 3] # Reference count: 1
another_ref = my_list # Reference count: 2
another_ref = None # Reference count: 1
my_list = None # Reference count: 0 - object eligible for cleanup
- Cyclic References
# Cyclic references that GC handles
class Node:
def __init__(self):
self.ref = None
a = Node()
b = Node()
a.ref = b # Create cycle
b.ref = a # Create cycle
Memory Optimization Techniques
Here are some practical tips for optimizing memory usage:
- Use Generators for Large Datasets
# Instead of this (memory-heavy)
numbers = [x * x for x in range(1000000)]
# Use this (memory-efficient)
numbers = (x * x for x in range(1000000))
- Utilize __slots__ for Classes
class Point:
__slots__ = ['x', 'y'] # Restricts attributes and reduces memory
def __init__(self, x, y):
self.x = x
self.y = y
Performance Tips
🚀 Quick performance boosters:
- Use array module for homogeneous data instead of lists
- Prefer set over list for membership testing
- Use collections.deque for queue operations
- Implement object pooling for frequently created objects
Variable Scope
Global vs Local Scope
Understanding scope is crucial for avoiding common pitfalls:
global_var = "I'm global"
def demonstrate_scope():
local_var = "I'm local"
print(global_var) # Works fine
# Modifying global requires 'global' keyword
global global_var
global_var = "Modified global"
demonstrate_scope()
print(global_var) # Shows "Modified global"
# print(local_var) # Would raise NameError
Nonlocal Variables
The nonlocal keyword is useful in nested functions:
def outer_function():
count = 0
def inner_function():
nonlocal count # Allows modification of outer variable
count += 1
return count
return inner_function
counter = outer_function()
print(counter()) # Output: 1
print(counter()) # Output: 2
Scope Resolution: LEGB Rule
Python follows the LEGB rule for scope resolution:
Scope | Description | Example Use Case |
Local | Inside current function | Function variables |
Enclosing | Outer function scope | Closure variables |
Global | Module level | Shared module state |
Built-in | Python’s built-ins | Built-in functions |
Best Practices for Scope Management
- Minimize Global Usage
# Instead of this
global_counter = 0
def increment():
global global_counter
global_counter += 1
# Prefer this
class Counter:
def __init__(self):
self._count = 0
def increment(self):
self._count += 1
- Use Function Arguments
# Instead of this
def process_data():
global config
# Use config...
# Prefer this
def process_data(config):
# Use config…
- Class-based State Management
class DataProcessor:
def __init__(self, config):
self.config = config
def process(self):
# Access self.config instead of global
pass
💡 Pro Tip: Use the locals() and globals() functions to inspect variable scopes during debugging.
Remember: Understanding these advanced concepts isn’t just about writing working code – it’s about writing efficient, maintainable, and scalable Python applications. The better you grasp memory management and scope, the more powerful your Python programs become.
Related Resource: Python Memory Management Documentation
Conclusion: Mastering Python Variables and Data Types
After diving deep into Python’s variables and data types, let’s consolidate what we’ve learned and chart your path forward.
Key Takeaways 🎯
Python’s approach to variables and data types sets it apart from many other programming languages, offering both flexibility and power:
- Dynamic Typing: Python’s dynamic typing system allows for flexible variable assignment while maintaining type safety. Remember, variables are labels pointing to objects, not boxes containing values.
- Built-in Data Types: Python provides a rich set of built-in data types:
- Immutable types (strings, integers, tuples) for data integrity
- Mutable types (lists, dictionaries, sets) for flexible data manipulation
- Special types (None, Boolean) for control flow and logic
- Type Conversion: Understanding when and how to convert between types is crucial for writing robust code and avoiding common pitfalls.
Learning Path Recommendations 🛣️
To continue building your Python expertise, consider this structured approach:
- Fundamentals Practice (1-2 weeks)
- Complete coding challenges focusing on data type manipulation
- Build small projects combining different data types
- Practice type conversion scenarios
- Intermediate Concepts (2-4 weeks)
- Explore object-oriented programming in Python
- Study advanced data structures
- Learn about memory management and optimization
- Advanced Topics (1-2 months)
- Dive into Python’s internals
- Study design patterns and best practices
- Contribute to open-source Python projects
Additional Resources 📚
Here are some high-quality resources to support your learning journey:
- Official Documentation
- Interactive Learning
- Books and References
- “Fluent Python” by Luciano Ramalho
- “Python Cookbook” by David Beazley and Brian K. Jones
Ready to Level Up Your Python Skills? 🚀
Now that you understand Python’s variables and data types, it’s time to put this knowledge into practice:
- Start Coding: Open your favorite IDE and experiment with the concepts we’ve covered.
- Join the Community: Connect with other Python developers on Python Discord or Reddit’s r/learnpython.
- Build Projects: Create something meaningful using your new knowledge.
- Share Knowledge: Help others learn by explaining these concepts in your own words.
Remember: mastering Python variables and data types is just the beginning of your Python journey. Each project and challenge you tackle will deepen your understanding and make you a more confident Python developer.
How was this guide helpful to you? Share your thoughts and experiences in the comments below! 👇
Frequently Asked Questions: Python Variables and Data Types
Let’s dive into the most common questions about Python variables and data types with practical examples and clear explanations.
1. What are the main differences between mutable and immutable types in Python?
Mutable types can be modified after creation, while immutable types cannot. Here’s a quick comparison:
# Immutable types example (strings)
name = "Python"
name.upper() # Creates a new string
print(name) # Still prints "Python"
# Mutable types example (lists)
numbers = [1, 2, 3]
numbers.append(4) # Modifies the original list
print(numbers) # Prints [1, 2, 3, 4]
Common mutable types:
- Lists
- Dictionaries
- Sets
Common immutable types:
- Strings
- Tuples
- Integers
- Floats
- Booleans
2. How do you check the data type of a variable in Python?
There are two main ways to check a variable’s type:
age = 25
name = "John"
# Using type() function
print(type(age)) # Output: <class 'int'>
print(type(name)) # Output: <class 'str'>
# Using isinstance() function
print(isinstance(age, int)) # Output: True
print(isinstance(name, str)) # Output: True
3. What are the four main data structures in Python?
The four main built-in data structures in Python are:
# 1. Lists - ordered, mutable sequences
my_list = [1, 2, 3, 4, 5]
# 2. Tuples - ordered, immutable sequences
my_tuple = (1, 2, 3, 4, 5)
# 3. Dictionaries - key-value pairs
my_dict = {'name': 'John', 'age': 30}
# 4. Sets - unordered collections of unique elements
my_set = {1, 2, 3, 4, 5}
Each has its own use cases and performance characteristics:
Data Structure | Ordered? | Mutable? | Duplicates? | Best Used For |
List | Yes | Yes | Yes | Ordered collections |
Tuple | Yes | No | Yes | Immutable sequences |
Dictionary | Yes* | Yes | No (keys) | Key-value mapping |
Set | No | Yes | No | Unique items |
- *As of Python 3.7+
4. How can you convert between different data types?
Python provides built-in functions for type conversion:
# String to Integer
age_str = "25"
age_int = int(age_str) # 25
# Integer to String
num = 100
num_str = str(num) # "100"
# String to Float
price_str = "19.99"
price_float = float(price_str) # 19.99
# List to Tuple
my_list = [1, 2, 3]
my_tuple = tuple(my_list) # (1, 2, 3)
# String to List
text = "Hello"
char_list = list(text) # ['H', 'e', 'l', 'l', 'o']
5. What is the difference between a variable and a data type?
A variable is a container for storing data, while a data type defines what kind of data can be stored:
# Variable = container
# Data type = what's inside
name = "John" # 'name' is the variable, 'str' is the data type
age = 25 # 'age' is the variable, 'int' is the data type
6. How do you define a variable as a list in Python?
There are several ways to create a list:
# Empty list
my_list = []
my_list = list()
# List with initial values
numbers = [1, 2, 3, 4, 5]
# List comprehension
squares = [x**2 for x in range(5)] # [0, 1, 4, 9, 16]
# Converting other types to list
letters = list("Python") # ['P', 'y', 't', 'h', 'o', 'n']
7. What are the five standard data types in Python?
The five fundamental data types are:
# 1. Numbers
integer_num = 42 # int
float_num = 3.14 # float
complex_num = 1 + 2j # complex
# 2. String
text = "Hello Python" # str
# 3. List
items = [1, 2, 3] # list
# 4. Tuple
coordinates = (x, y) # tuple
# 5. Dictionary
person = {'name': 'John'} # dict
8. Is float a data type in Python?
Yes, float is a built-in data type for decimal numbers:
# Float examples
price = 19.99
pi = 3.14159
scientific = 1.23e-4
# Float operations
result = 10.5 + 5.5 # 16.0
division = 22 / 7 # 3.142857142857143
9. What are the four standard data types in Python?
Python includes four fundamental data types that form the building blocks of data manipulation:
- Numeric Types:
- Integers (int): Whole numbers like 42, -17, 0
- Floating-point (float): Decimal numbers like 3.14, -0.001
- Complex numbers (complex): Numbers with real and imaginary parts like 3+4j
- String Type (str):
- Text data enclosed in quotes
- Examples: “Hello”, ‘Python’, “””Multi-line text”””
- Boolean Type (bool):
- Logical values: True or False
- Used in conditional statements and comparisons
- None Type (NoneType):
- Special type representing absence of value
- Only has one value: None
# Examples of standard data types
number = 42 # Integer
decimal = 3.14 # Float
text = "Python" # String
is_valid = True # Boolean
empty = None # None type
10. How to identify data type in Python?
Python offers several built-in methods to check a variable’s data type:
- Using the type() function:
x = 42
print(type(x)) # Output: <class 'int'>
name = "Python"
print(type(name)) # Output: <class 'str'>
- Using isinstance() function:
x = 42
print(isinstance(x, int)) # Output: True
print(isinstance(x, str)) # Output: False
Pro tip: The type() function is more commonly used for debugging, while isinstance() is preferred in production code as it handles inheritance properly.
11. What is the difference between datatypes in Python?
Here’s a comprehensive comparison of Python’s main data types:
Data Type | Mutability | Ordered | Indexed | Example | Common Use Case |
int | Immutable | N/A | N/A | 42 | Counting, math operations |
float | Immutable | N/A | N/A | 3.14 | Scientific calculations |
str | Immutable | Yes | Yes | “Hello” | Text processing |
list | Mutable | Yes | Yes | [1, 2, 3] | Collections of items |
tuple | Immutable | Yes | Yes | (1, 2, 3) | Fixed collections |
dict | Mutable | No* | No | {“a”: 1} | Key-value mappings |
set | Mutable | No | No | {1, 2, 3} | Unique collections |
- *Note: As of Python 3.7+, dictionaries maintain insertion order.
12. What are variables and their types with examples?
Variables in Python are named references to memory locations storing data. Here’s a comprehensive overview:
# Numeric variables
count = 100 # Integer
price = 19.99 # Float
complex_num = 3 + 4j # Complex
# String variables
name = "Python" # Single line string
description = """ # Multi-line string
This is a
multi-line text
"""
# Collection variables
my_list = [1, 2, 3] # List
my_tuple = (1, 2, 3) # Tuple
my_dict = {"a": 1, "b": 2} # Dictionary
my_set = {1, 2, 3} # Set
# Boolean variables
is_active = True # Boolean
has_error = False # Boolean
# None type
empty_value = None # NoneType
13. How can you know the datatype of a variable?
There are multiple ways to determine a variable’s type:
# Method 1: Using type()
value = 42
print(type(value)) # Output: <class 'int'>
# Method 2: Using isinstance()
print(isinstance(value, int)) # Output: True
# Method 3: String representation of type
print(value.__class__.__name__) # Output: 'int'
Best practice: Use isinstance() when checking types in conditional statements:
def process_data(value):
if isinstance(value, (int, float)):
return value * 2
elif isinstance(value, str):
return value.upper()
else:
raise TypeError("Unsupported data type")
14. Is string a data type in Python?
Yes, string (str) is one of Python’s built-in data types. Strings are immutable sequences of Unicode characters:
# Different ways to create strings
single_quoted = 'Python'
double_quoted = "Python"
multi_line = """Python
is awesome!"""
# String operations
name = "Python"
print(len(name)) # Output: 6
print(name.upper()) # Output: PYTHON
print(name[0]) # Output: P
print(name[1:4]) # Output: yth
15. Is an array a data type in Python?
No, array isn’t a built-in data type in Python. Instead, Python provides several alternatives:
- Lists: Python’s built-in sequence type
numbers = [1, 2, 3, 4, 5]
- NumPy Arrays: Efficient array operations (requires NumPy library)
import numpy as np
numpy_array = np.array([1, 2, 3, 4, 5])
- Array Module: Less commonly used but available in standard library
from array import array
int_array = array('i', [1, 2, 3, 4, 5])
Pro tip: For most purposes, Python lists are sufficient. Use NumPy arrays for numerical computations and when performance is critical.
Remember: Understanding these data types and their appropriate use cases is crucial for writing efficient Python code. Always choose the right data type for your specific needs to optimize performance and maintainability.
One thought on “Python Variables & Data Types: Guide with 50+ Examples”