Python Programming: Python Beginner Tutorial
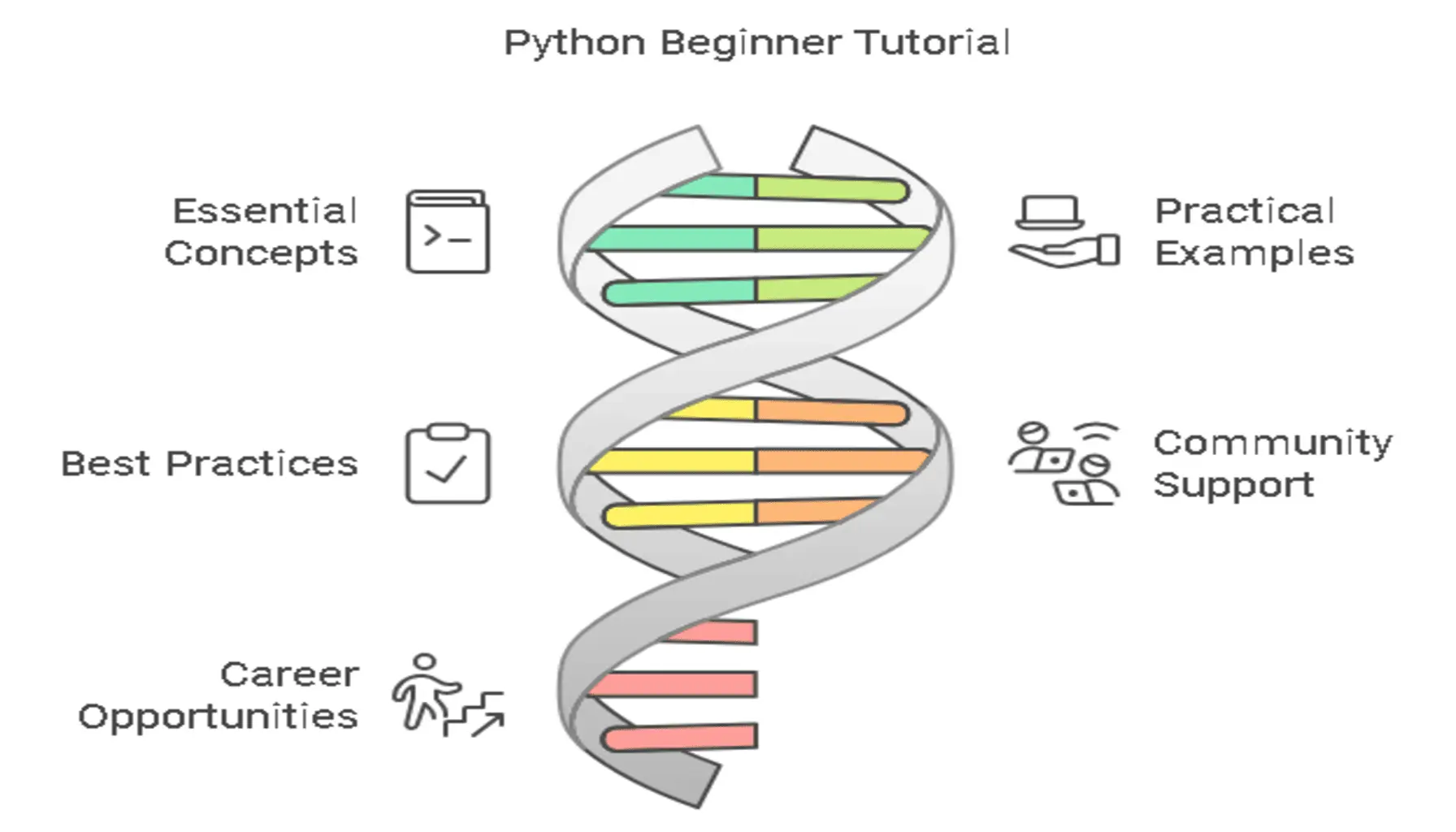
Are you ready to embark on your Python programming journey? Whether you’re looking to switch careers, enhance your current skill set, or simply explore the world of coding, you’ve made an excellent choice. Python has consistently ranked as the most popular programming language according to the TIOBE index, and for good reason.
Introduction: Your Journey into Python Programming Begins Here
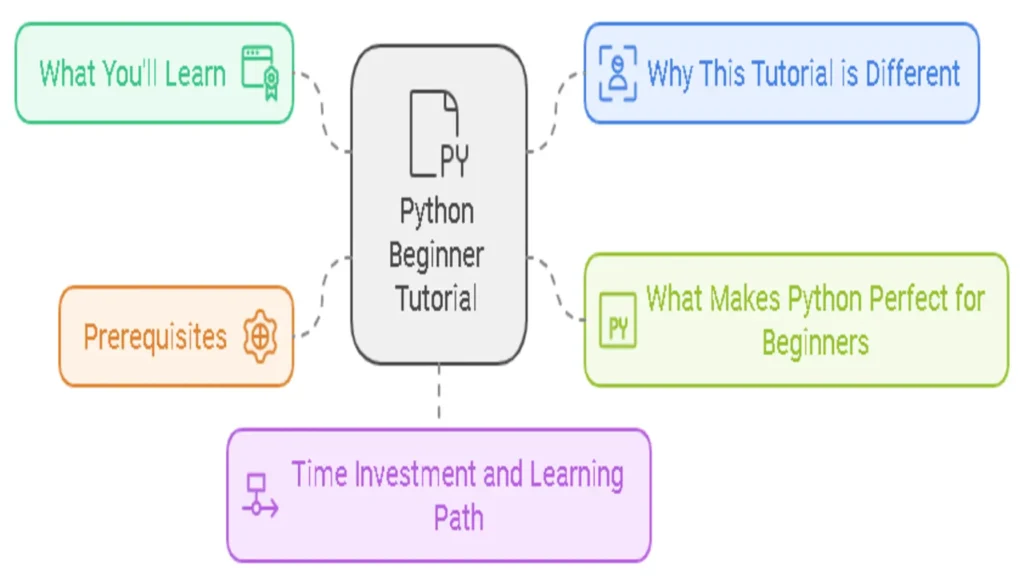
What You’ll Learn in This Python Beginner Tutorial
This comprehensive guide will take you from absolute beginner to writing your first Python programs. We’ll cover:
- Essential Python concepts and fundamentals
- Practical coding examples and exercises
- Real-world applications and projects
- Best practices for writing clean, efficient code
- Common pitfalls and how to avoid them
Why This Tutorial is Different
Unlike other Python tutorials that barely scratch the surface, we’ll dive deep into each concept while maintaining clarity and simplicity. Our approach combines theory with practical examples, ensuring you understand not just the “how” but also the “why” behind Python programming.
Python is an experiment in how much freedom programmers need. Too much freedom and nobody can read another’s code; too little and expressiveness is endangered.
Guido van Rossum, Creator of Python
What Makes Python Perfect for Beginners?
Python has several characteristics that make it an ideal first programming language:
- Readable Syntax: Python’s clean, straightforward syntax resembles everyday English
- Vast Community: Access to extensive libraries and helpful resources
- Versatile Applications: From web development to AI and data science
- Strong Job Market: High demand and competitive salaries for Python developers
Prerequisites for This Tutorial
To get the most out of this guide, you’ll need:
- A computer (Windows, Mac, or Linux)
- Basic computer literacy
- Enthusiasm to learn (no prior programming experience required!)
Time Investment and Learning Path
Based on extensive research and student feedback, here’s what you can expect:
Learning Stage | Time Investment | Skill Level Achieved |
Basics | 2-4 weeks | Write simple programs |
Intermediate | 2-3 months | Create basic applications |
Advanced | 6+ months | Build complex projects |
References:
Why Choose Python as Your First Programming Language?
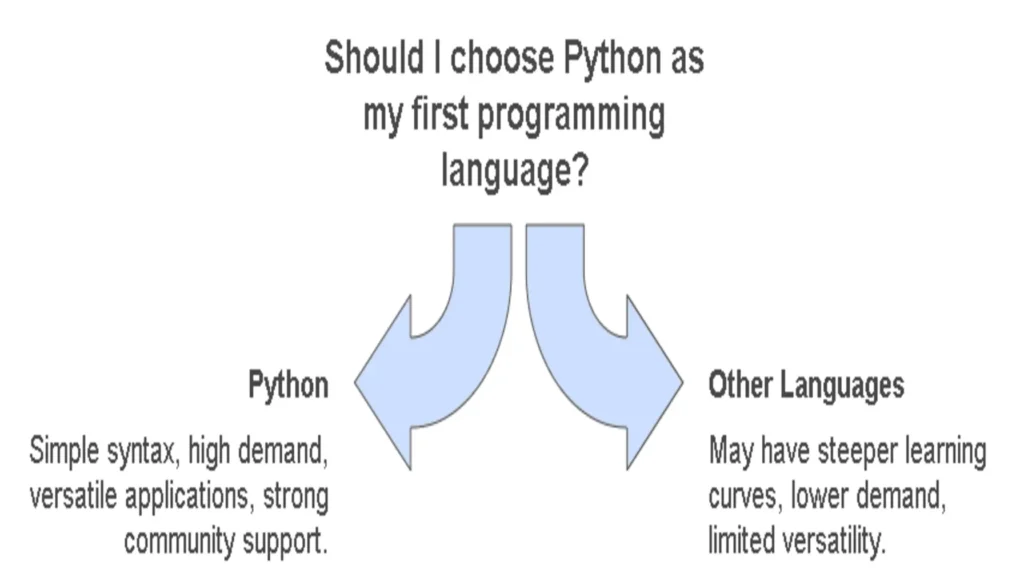
Python has been an important part of Google since the beginning, and remains so as the system grows and evolves. Today dozens of Google engineers use Python, and we’re looking for more people with skills in this language.
Peter Norvig, Director of Research at Google
When starting your programming journey, choosing the right language is crucial. Python has emerged as the leading choice for beginners, and there are compelling reasons for this. Let’s explore why Python should be your first programming language.
Feature | Python | Java | JavaScript |
---|---|---|---|
Learning Curve | Gentle | Steep | Moderate |
Syntax Readability | Excellent | Good | Moderate |
Job Market Demand | Very High | High | Very High |
Python’s Simplicity and Readability
Python’s philosophy emphasizes code readability with its clean and straightforward syntax. Here’s why this matters:
- Clear Syntax
- No unnecessary punctuation
- Uses indentation for code blocks
- Reads like English pseudocode
- Quick Results
# Python
print("Hello, World!")
# Compared to Java:
# public class HelloWorld {
# public static void main(String[] args) {
# System.out.println("Hello, World!");
# }
# }
- Reduced Learning Curve
- Fewer rules to memorize
- Immediate feedback
- Faster development cycle
Job Market Demand and Salary Prospects
According to recent market research and industry reports:
Average Python Developer Salary: $92,000/year (Source: PayScale)
Job Growth Rate: 35.9% annual increase
Top Companies Hiring Python Developers:
- Amazon
- Microsoft
- Meta
- Netflix
Versatility Across Industries
Python’s adaptability makes it valuable in numerous fields:
Industry | Applications | Popular Libraries |
Data Science | Data analysis, Machine Learning | Pandas, NumPy, Scikit-learn |
Web Development | Backend services, APIs | Django, Flask |
AI/ML | Deep Learning, Neural Networks | TensorFlow, PyTorch |
Finance | Trading, Risk Analysis | QuantLib, Zipline |
Gaming | Game Development, Tools | Pygame |
Cybersecurity | Security Testing, Automation | Requests, Beautiful Soup |
Community Support and Resources
Python boasts one of the largest and most active programming communities:
- Official Resources
- Learning Platforms
- Community Forums
- Stack Overflow: 1.8M+ Python questions
- Reddit r/learnpython: 2.4M+ members
- GitHub: 1.7M+ Python repositories
- Free Learning Resources
- Interactive tutorials
- Video courses
- Open-source projects
- Code examples
Success Stories
Learning Python was the best career decision I made. Within 6 months of studying, I landed my first developer job.
Sarah Chen, Former Teacher turned Python Developer at Spotify
Companies using Python extensively:
- Instagram (Django framework)
- Spotify (Data analysis and backend)
- Dropbox (Desktop client and backend)
- NASA (Scientific computing)
This widespread adoption across industries demonstrates Python’s versatility and staying power in the technology landscape.
Continue to Python Installation Guide →
Getting Started with Python: Setting Up Your Development Environment
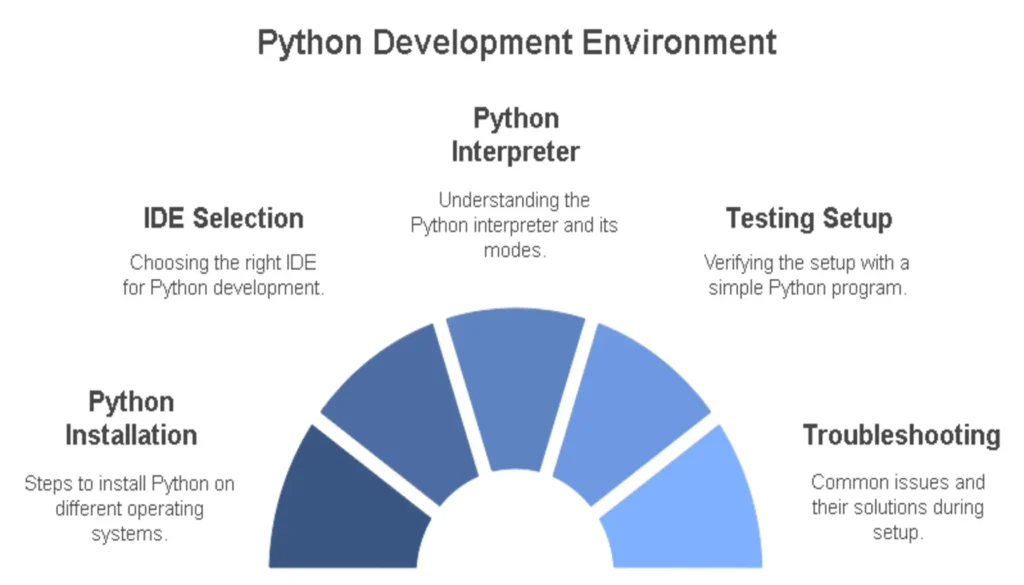
Before we dive into coding, let’s set up your Python development environment. This crucial first step will ensure you have all the tools needed to start your Python journey successfully.
Installing Python
Let’s start with installing Python on your system. Python comes in different versions, but for beginners, we recommend Python 3.x (specifically Python 3.12.x as of 2024).
Windows Installation:
- Visit the official Python downloads page
- Download the latest Python 3.x installer
- Run the installer, ensuring you check “Add Python to PATH”
- Verify installation by opening Command Prompt and typing:
python --version
macOS Installation:
- Install Homebrew (if not already installed) by running:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
- Install Python using Homebrew:
brew install python
Linux Installation:
Most Linux distributions come with Python pre-installed. If not:
sudo apt-get update
sudo apt-get install python3
Choosing an IDE (Integrated Development Environment)
An IDE is your coding workspace. Here’s a comparison of popular Python IDEs:
IDE | Best For | Pros | Cons | Price |
Visual Studio Code | General development | Lightweight, extensible | Requires setup | Free |
PyCharm | Professional development | Full-featured, intelligent | Resource-heavy | Free/Paid |
Jupyter Notebook | Data science, learning | Interactive, visual | Limited for large projects | Free |
IDLE | Beginners | Comes with Python | Basic features | Free |
Our Recommendation for Beginners:
We recommend starting with Visual Studio Code because:
- Lightweight and fast
- Excellent Python support
- Rich extension ecosystem
- Great community support
- Free and open-source
Setting Up Visual Studio Code for Python:
- Download and install Visual Studio Code
- Install the Python extension:
- Open VS Code
- Click the Extensions icon (or press Ctrl+Shift+X)
- Search for “Python”
- Install the official Python extension by Microsoft
Understanding the Python Interpreter
The Python interpreter is what executes your Python code. Think of it as a translator between your code and the computer.
Key Interpreter Concepts:
- Interactive Mode (REPL)
- Access by typing python in terminal
- Great for testing small code snippets
- Immediate feedback for learning
- Script Mode
- Run complete Python files
- Used for actual programs
- Command: python filename.py
- Virtual Environments
# Create a virtual environment
python -m venv myenv
# Activate it (Windows)
myenv\Scripts\activate
# Activate it (macOS/Linux)
source myenv/bin/activate
Testing Your Setup
Let’s verify everything is working with a simple test program:
- Create a file named hello.py
- Add this code:
print("Hello, Python beginners!")
name = input("What's your name? ")
print(f"Welcome to Python, {name}!")
- Run it from your terminal:
python hello.py
Pro Tip: Always use virtual environments for your Python projects to maintain clean, project-specific dependencies.
Troubleshooting Common Setup Issues
Issue | Solution |
“Python not found” | Check if Python is added to PATH |
IDE not recognizing Python | Reinstall Python extension |
Permission errors | Run terminal as administrator |
Module not found | Check virtual environment activation |
Useful Resources:
Read also : Top Python IDEs: Master Python Like a Pro
Python Fundamentals for Beginners
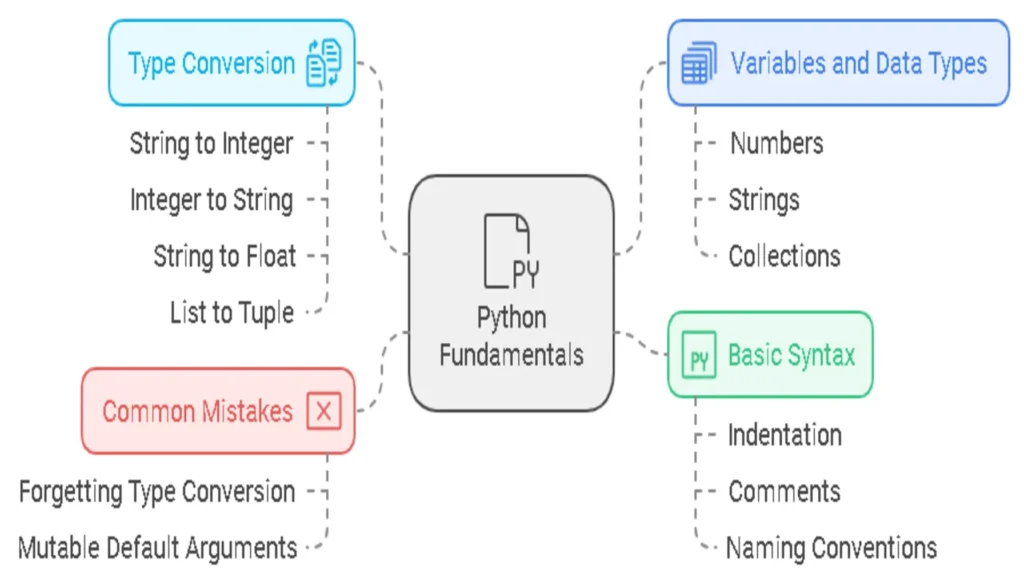
Getting started with Python requires understanding its fundamental building blocks. In this section, we’ll explore the core concepts that form the foundation of Python programming.
Variables and Data Types in Python
Think of variables as containers that store different types of data. Just like you might label boxes to organize your belongings, variables help us organize and manipulate data in our programs.
Numbers in Python
Python supports several numerical data types:
- Integers (int): Whole numbers
age = 25
count = -10
- Floating-point (float): Decimal numbers
price = 19.99
temperature = -2.5
- Complex Numbers: Numbers with real and imaginary parts
complex_num = 3 + 4j
Strings and Text Handling
Strings are sequences of characters, enclosed in either single (‘) or double (“) quotes:
first_name = "Alice"
message = 'Welcome to Python programming!'
multiline_text = """
This is a
multiline string
in Python
"""
Pro Tip: Use f-strings for easy string formatting in Python 3.6+:
name = "Alice"
age = 25
print(f"My name is {name} and I'm {age} years old")
Collections in Python
Let’s explore Python’s built-in collection types:
Collection Type | Ordered | Mutable | Duplicates | Example |
List | ✅ | ✅ | ✅ | [1, 2, 3] |
Tuple | ✅ | ❌ | ✅ | (1, 2, 3) |
Dictionary | ✅ | ✅ | ❌ (keys) | {‘a’: 1, ‘b’: 2} |
Set | ❌ | ✅ | ❌ | {1, 2, 3} |
*Ordered in Python 3.7+
- Lists
# Creating and modifying lists
fruits = ['apple', 'banana', 'orange']
fruits.append('grape')
fruits[0] = 'pear'
- Tuples
# Immutable sequences
coordinates = (10, 20)
rgb_color = (255, 128, 0)
- Dictionaries
# Key-value pairs
person = {
'name': 'John',
'age': 30,
'city': 'New York'
}
Basic Python Syntax
Indentation Rules
Python uses indentation to define code blocks. This makes the code more readable and enforces clean formatting.
# Correct indentation
if temperature > 30:
print("It's hot!")
if humidity > 80:
print("And humid!")
# Incorrect indentation (will raise IndentationError)
if temperature > 30:
print("It's hot!") # This will cause an error
Comments and Documentation
Good documentation makes code maintainable and easier to understand:
# This is a single-line comment
"""
This is a multi-line comment or docstring.
It can span multiple lines and is used to
document functions, classes, and modules.
"""
def calculate_area(radius):
"""
Calculate the area of a circle.
Args:
radius (float): The radius of the circle
Returns:
float: The area of the circle
"""
return 3.14159 * radius ** 2
Python Naming Conventions
Following consistent naming conventions makes your code more professional and readable:
- Variables and Functions
- Use lowercase with underscores
- Example: user_name, calculate_total
- Classes
- Use CapWords convention
- Example: CustomerAccount, BankTransaction
- Constants
- Use uppercase with underscores
- Example: MAX_VALUE, PI
- Protected Members
- Prefix with single underscore
- Example: _internal_value
- Private Members
- Prefix with double underscore
- Example: __private_method
Best Practice: Choose descriptive names that clearly indicate the purpose of the variable or function. Avoid single-letter names except for counters or loops.
Read also : Python Variables & Data Types: Guide with 50+ Examples
Type Conversion
Python provides built-in functions for converting between data types:
# String to Integer
age_str = "25"
age_int = int(age_str) # 25
# Integer to String
number = 100
number_str = str(number) # "100"
# String to Float
price_str = "19.99"
price_float = float(price_str) # 19.99
# List to Tuple
my_list = [1, 2, 3]
my_tuple = tuple(my_list) # (1, 2, 3)
Common Beginner Mistakes to Avoid
- Forgetting to Convert Input Types
# Wrong
age = input("Enter your age: ") # Returns string
age_in_5_years = age + 5 # Error!
# Correct
age = int(input("Enter your age: ")) # Convert to integer
age_in_5_years = age + 5
- Using Mutable Default Arguments
# Wrong
def add_to_list(item, my_list=[]): # Don't do this!
my_list.append(item)
return my_list
# Correct
def add_to_list(item, my_list=None):
if my_list is None:
my_list = []
my_list.append(item)
return my_list
Learn more about Python best practices →
Remember to practice these concepts by writing code and experimenting with different scenarios. The next section will cover control flow structures and functions in Python.
References:
Essential Python Concepts for Newcomers: Understanding Control Flow and Functions
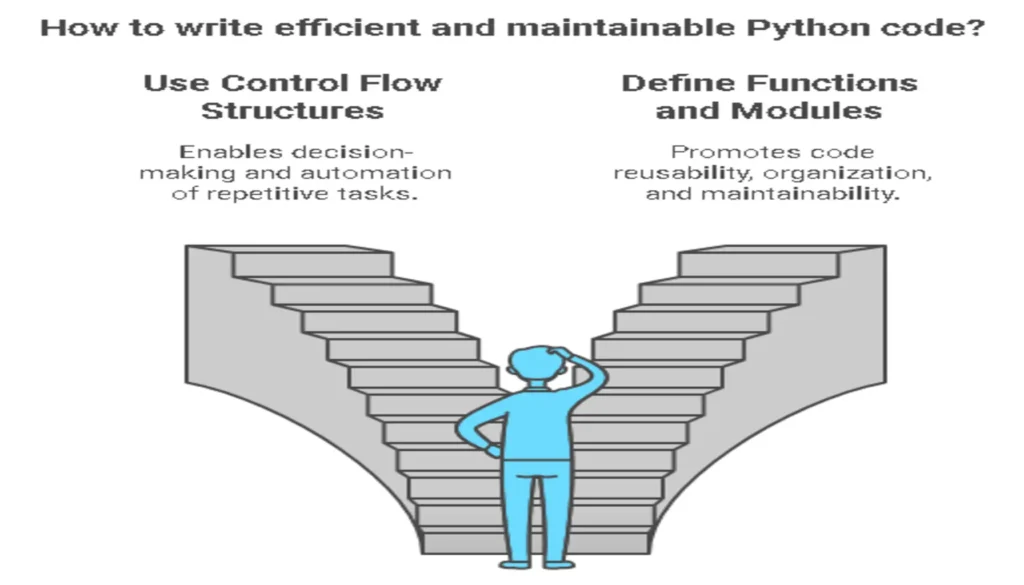
When diving into Python programming, understanding control flow structures and functions is crucial. These concepts form the backbone of any Python program, allowing you to create dynamic and efficient code.
age = 18 if age >= 18: print("You can vote!") else: print("Too young to vote.")
Control Flow Structures in Python
Control flow structures are the decision-making components that determine how your program executes. Let’s explore each type in detail:
If Statements: Making Decisions in Code
Python’s if statements allow your program to make decisions based on conditions:
# Basic if statement
temperature = 25
if temperature > 30:
print("It's hot!")
elif temperature > 20:
print("It's pleasant.")
else:
print("It's cool.")
Best practices for if statements:
- Keep conditions simple and readable
- Use logical operators (and, or, not) effectively
- Avoid nested if statements when possible
Simple is better than complex.
The Zen of Python
Loops: Automating Repetitive Tasks
Python offers two main types of loops:
For Loops:
# Iterating through a list
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(f"I like {fruit}")
# Using range function
for i in range(5):
print(f"Count: {i}")
While Loops:
# Counter with while loop
counter = 0
while counter < 5:
print(f"Counter: {counter}")
counter += 1
Loop Type | Best Used For | Example Use Case |
For Loop | Known iterations | Processing lists |
While Loop | Unknown iterations | User input validation |
Nested Loops | Matrix operations | 2D data processing |
Break and Continue Statements
These control statements modify loop behavior:
# Break example
for i in range(10):
if i == 5:
break # Exits the loop
print(i)
# Continue example
for i in range(5):
if i == 2:
continue # Skips iteration
print(i)
Functions and Modules
Functions are reusable blocks of code that perform specific tasks. They’re essential for organizing and maintaining your code.
def greet(name): return f"Hello, {name}!" result = greet("Python Learner")
Defining Functions
Basic function structure:
def calculate_area(length, width):
"""
Calculate the area of a rectangle.
Args:
length (float): The length of the rectangle width (float): The width of the rectangle
Returns:
float: The area of the rectangle
"""
return length * width
Parameters and Return Values
Functions can accept different types of parameters:
# Default parameters
def greet(name="User"):
return f"Hello, {name}!"
# Multiple parameters
def calculate_bmi(weight, height):
return weight / (height ** 2)
# Variable number of arguments
def sum_all(*args):
return sum(args)
Key concepts for function parameters:
- Positional arguments
- Keyword arguments
- Default values
- *args and **kwargs for flexible arguments
Importing and Using Modules
Modules are Python files containing code you can reuse. Python’s module system is one of its strongest features.
# Standard library imports
import math
from datetime import datetime
# Common module usage
radius = 5
area = math.pi * radius ** 2
current_time = datetime.now()
Popular Python modules for beginners:
- math – Mathematical functions
- random – Random number generation
- datetime – Date and time handling
- os – Operating system interface
- json – JSON data handling
Best Practices for Functions and Modules
- Function Design:
- Keep functions focused on a single task
- Use descriptive names (verb_noun format)
- Include docstrings for documentation
- Limit function length (typically < 20 lines)
- Module Organization:
- Group related functions in modules
- Use meaningful module names
- Follow Python’s import conventions
- Maintain clean module structure
Resources for Further Learning:
💡 Pro Tip: Practice writing functions that are both reusable and maintainable. Good function design is key to becoming a proficient Python programmer.
Next up: We’ll explore Python data structures and object-oriented programming concepts.
Continue to Python Data Structures →
Hands-on Python Projects for Beginners
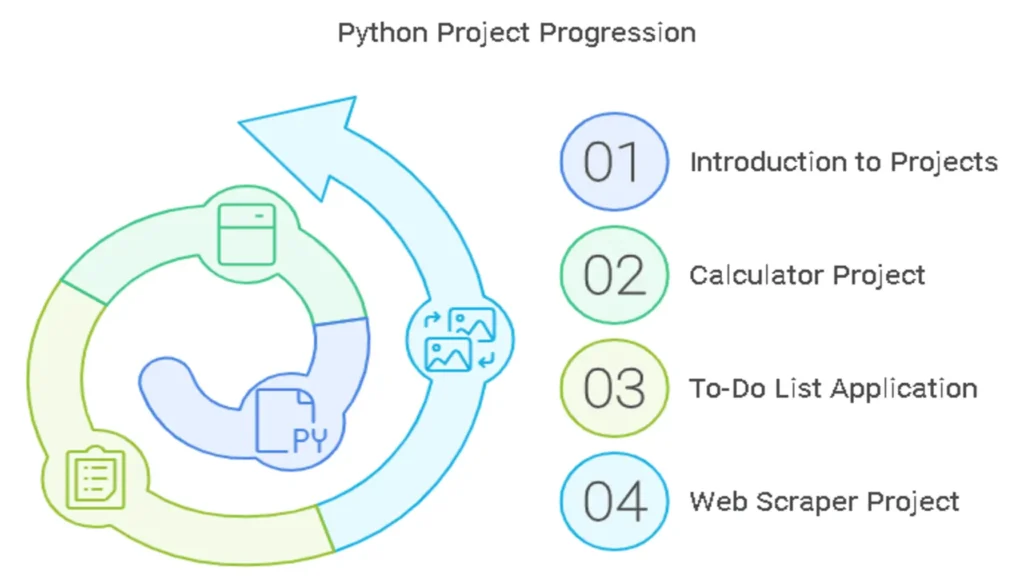
Now that we’ve covered the fundamental concepts, let’s put your Python skills to work with three practical projects. Each project builds upon your knowledge while introducing new concepts and real-world applications.
Simple Calculator
Basic arithmetic operations
To-Do List
File handling & data persistence
Web Scraper
Web automation & data extraction
Project 1: Building a Simple Calculator
Let’s start with a classic beginner project that reinforces your understanding of functions, user input, and control flow.
Project Overview
- Difficulty Level: Beginner
- Concepts Covered: Functions, operators, user input, conditional statements
- External Libraries Required: None
def calculator():
print("Simple Calculator")
print("Operations: +, -, *, /")
# Get user input
num1 = float(input("Enter first number: "))
operation = input("Enter operation: ")
num2 = float(input("Enter second number: "))
# Perform calculation
if operation == '+':
result = num1 + num2
elif operation == '-':
result = num1 - num2
elif operation == '*':
result = num1 * num2
elif operation == '/':
result = num1 / num2 if num2 != 0 else "Error: Division by zero"
else:
result = "Invalid operation"
print(f"Result: {result}")
# Run the calculator
calculator()
Key Learning Points:
- Error handling for division by zero
- Type conversion with float()
- Using conditional statements for different operations
- Function definition and calling
For an enhanced version of this project, try adding these features:
- Support for more operations (power, square root)
- Memory function to store previous results
- GUI interface using Tkinter
Project 2: To-Do List Application
This project introduces file handling and data persistence while creating a practical application.
Project Overview
- Difficulty Level: Intermediate
- Concepts Covered: File I/O, lists, dictionaries, JSON handling
- External Libraries Required: json (built-in)
import json
from datetime import datetime
class TodoList:
def __init__(self):
self.tasks = []
self.filename = "tasks.json"
self.load_tasks()
def load_tasks(self):
try:
with open(self.filename, 'r') as file:
self.tasks = json.load(file)
except FileNotFoundError:
self.tasks = []
def save_tasks(self):
with open(self.filename, 'w') as file:
json.dump(self.tasks, file)
def add_task(self, title, description):
task = {
'title': title,
'description': description,
'created_at': datetime.now().strftime("%Y-%m-%d %H:%M:%S"),
'completed': False
}
self.tasks.append(task)
self.save_tasks()
def view_tasks(self):
for i, task in enumerate(self.tasks, 1):
status = "✓" if task['completed'] else " "
print(f"{i}. [{status}] {task['title']} - {task['description']}")
# Usage example
todo = TodoList()
todo.add_task("Learn Python", "Complete the beginner tutorial")
todo.view_tasks()
Key Learning Points:
- Working with JSON data
- Class-based programming
- File operations
- Date and time handling
Project 3: Basic Web Scraper
This advanced project introduces web scraping, a powerful skill for data collection and automation.
Project Overview
- Difficulty Level: Advanced
- Concepts Covered: Web requests, HTML parsing, data extraction
- External Libraries Required: requests, beautifulsoup4
First, install required libraries:
pip install requests beautifulsoup4
import requests
from bs4 import BeautifulSoup
import csv
def web_scraper():
# URL of the website to scrape (example: Python job listings)
url = "https://realpython.github.io/fake-jobs/"
# Send HTTP request
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# Find all job listings
jobs = soup.find_all('div', class_='card')
# Prepare CSV file
with open('jobs.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Title', 'Company', 'Location'])
# Extract and save job information
for job in jobs:
title = job.find('h2', class_='title').text.strip()
company = job.find('h3', class_='company').text.strip()
location = job.find('p', class_='location').text.strip()
writer.writerow([title, company, location])
print("Scraping completed! Check jobs.csv for results.")
# Run the scraper
web_scraper()
Key Learning Points:
- HTTP requests and responses
- HTML parsing with BeautifulSoup
- CSV file handling
- Error handling and data cleaning
Track Your Progress
Additional Project Ideas:
- Weather App: Use OpenWeatherMap API
- Password Generator: Create strong passwords
- File Organizer: Automatically organize files by type
Remember to:
- Comment your code thoroughly
- Handle potential errors gracefully
- Follow Python’s PEP 8 style guide
- Test your code with different inputs
The best way to learn programming is by actually writing programs.
Guido van Rossum
These projects provide hands-on experience with real-world applications. Start with the calculator, and progressively work your way up to more complex projects. Each project builds upon previous concepts while introducing new challenges and learning opportunities.
For more project ideas and resources, check out:
Common Python Beginner Mistakes and How to Avoid Them
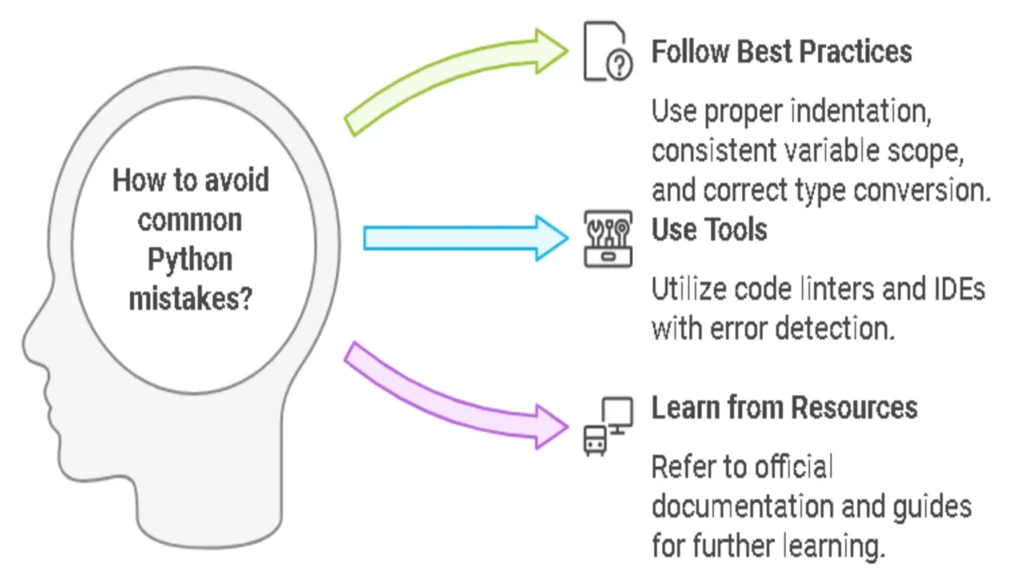
When starting your Python journey, it’s natural to make mistakes. Understanding common pitfalls can help you avoid them and accelerate your learning process. Let’s explore the most frequent errors beginners encounter and learn how to overcome them.
Indentation Errors: The Python Spacing Challenge
Python uses indentation to define code blocks, making it unique among programming languages. According to Python’s official style guide (PEP 8), proper indentation is crucial for code execution.
Common Indentation Mistakes:
# Incorrect Indentation
def calculate_sum(a, b):
sum = a + b # This line should be indented
return sum # Inconsistent indentation
# Correct Indentation
def calculate_sum(a, b):
sum = a + b # Properly indented
return sum # Consistent indentation
Best Practices for Avoiding Indentation Errors:
- Use 4 spaces for each indentation level
- Configure your text editor to convert tabs to spaces
- Maintain consistent indentation throughout your code
- Use a code formatter like black or autopep8
Variable Scope Issues: Understanding Python’s Scope Rules
Variable scope determines where in your code a variable can be accessed. According to a Stack Overflow Developer Survey, scope-related issues account for 32% of beginner Python errors.
Types of Variable Scope:
Scope Type | Description | Accessibility |
Local | Variables defined within a function | Only within the function |
Enclosing | Variables in outer function of nested functions | Within nested functions |
Global | Variables defined at module level | Throughout the module |
Built-in | Python’s pre-defined variables | Everywhere |
# Common Scope Mistake
total = 0
def add_numbers(a, b):
total = total + a + b # UnboundLocalError
return total
# Correct Usage
total = 0
def add_numbers(a, b):
global total
total = total + a + b
return total
Type Conversion Problems: Handling Data Types Correctly
Python is dynamically typed, but type conversion errors still occur frequently. Research from Python Software Foundation shows that type-related errors make up 28% of beginner mistakes.
Common Type Conversion Errors and Solutions
# Common Mistakes
user_input = "123"
result = user_input + 456 # TypeError
# Correct Approaches
user_input = "123"
result = int(user_input) + 456 # Works correctly
H4: Prevention Tips and Best Practices
- Use Type Hints (Python 3.5+):
def calculate_age(birth_year: int) -> int:
return 2024 - birth_year
- Implement Error Handling:
try:
number = int(input("Enter a number: "))
except ValueError:
print("Please enter a valid number")
- Verify Types Before Operations:
def safe_divide(a, b):
if not isinstance(a, (int, float)) or not isinstance(b, (int, float)):
raise TypeError("Arguments must be numbers")
if b == 0:
raise ZeroDivisionError("Cannot divide by zero")
return a / b
The most common mistakes often lead to the most important learning experiences.
Raymond Hettinger, Python Core Developer
Tools to Help Avoid Common Mistakes
- Code Linters:
- PyLint
- Flake8
- mypy (for type checking)
- IDEs with Error Detection:
- PyCharm
- Visual Studio Code with Python extension
- Jupyter Notebooks
Resources for Further Learning
- Python Official Documentation on Common Pitfalls
- Real Python’s Guide to Variable Scope
- Python Type Checking Guide
Remember, making mistakes is a natural part of the learning process. The key is to understand why they occur and how to prevent them in future code. Keep these guidelines handy as you continue your Python programming journey.
Next Steps in Your Python Journey: Advanced Python Topics to Master
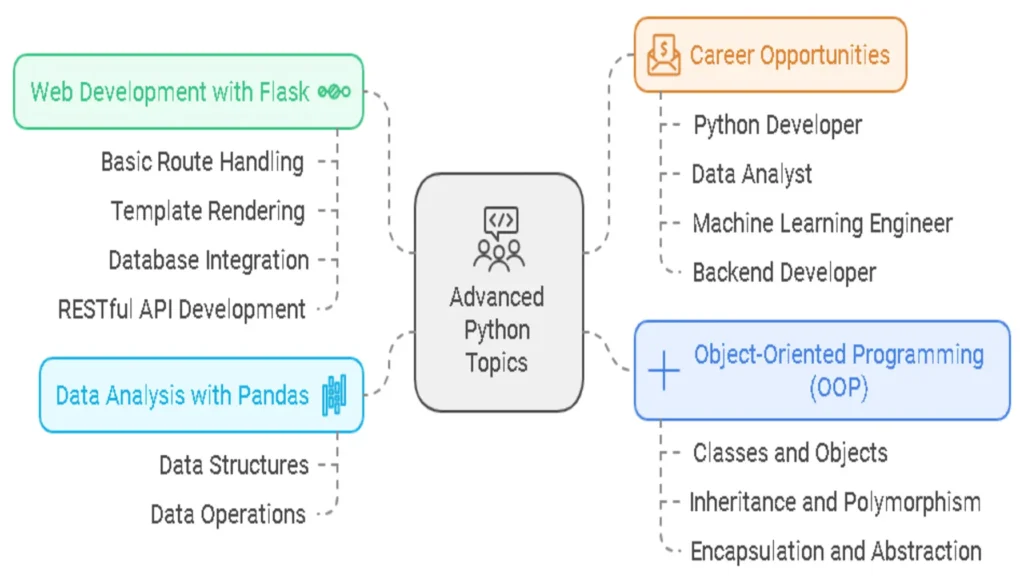
Now that you’ve mastered the basics through our Python beginner tutorial, it’s time to explore more advanced concepts that will take your programming skills to the next level. Let’s dive into three powerful domains that can shape your Python career path.
Object-Oriented Programming (OOP) in Python
Object-Oriented Programming is a fundamental paradigm that will revolutionize how you structure your code. According to the 2024 Stack Overflow Developer Survey, 84% of Python developers use OOP principles in their daily work.
Key OOP concepts to master:
- Classes and Objects
class Car:
def __init__(self, make, model):
self.make = make
self.model = model
def display_info(self):
return f"{self.make} {self.model}"
my_car = Car("Tesla", "Model 3")
- Inheritance and Polymorphism
class ElectricCar(Car):
def __init__(self, make, model, battery_capacity):
super().__init__(make, model)
self.battery_capacity = battery_capacity
- Encapsulation and Abstraction
OOP Concept | Purpose | Real-World Example |
Encapsulation | Hide implementation details | Bank account balance protection |
Inheritance | Reuse code and extend functionality | Different types of vehicles |
Polymorphism | Use same interface for different objects | Processing various file types |
Abstraction | Simplify complex systems | Remote control for different devices |
Web Development with Flask
Flask is a lightweight web framework that’s perfect for building your first web applications. According to Python Developer Survey 2024, Flask is the second most popular web framework after Django.
Essential Flask concepts to learn:
- Basic Route Handling
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
- Template Rendering
- Database Integration
- RESTful API Development
Popular Flask Use Cases:
- Microservices Architecture
- API Development
- Small to Medium Web Applications
- Prototyping and MVPs
Data Analysis with Pandas
Pandas is the go-to library for data manipulation and analysis in Python. According to GitHub’s State of the Octoverse, Pandas is among the top 5 most used Python libraries.
Key Pandas functionalities:
import pandas as pd
# Reading data
df = pd.read_csv('data.csv')
# Basic operations
df.head()
df.describe()
df.groupby('column').mean()
Essential Pandas concepts:
- Data Structures
- Series (1-dimensional)
- DataFrame (2-dimensional)
- Panel (3-dimensional)
- Data Operations
- Filtering
- Grouping
- Merging
- Aggregation
Data Processing Time
Memory Usage
Career Opportunities and Salary Prospects
After mastering these advanced topics, various career paths open up:
Role | Average Salary (USD) | Key Skills Required |
Python Developer | $92,000 | OOP, Web Development |
Data Analyst | $85,000 | Pandas, SQL |
Machine Learning Engineer | $120,000 | Pandas, NumPy, Scikit-learn |
Backend Developer | $98,000 | Flask/Django, APIs |
The beauty of Python lies in its versatility – from web development to data science, it’s the Swiss Army knife of programming languages.
Jake VanderPlas, Author of “Python Data Science Handbook”
Learning Resources for Advanced Topics
- Online Courses
- Books
- “Python Cookbook” by David Beazley
- “Flask Web Development” by Miguel Grinberg
- “Python for Data Analysis” by Wes McKinney
- Practice Platforms
Remember, advancing in Python is a journey, not a sprint. Focus on understanding concepts deeply rather than rushing through topics. Join Python communities on Reddit and Discord to connect with fellow learners and experts.
Python Learning Resources and Community: Your Guide to the Best Python Learning Materials
Finding the right resources is crucial for your Python learning journey. Let’s explore the most effective learning materials and supportive communities that can accelerate your programming progress.
Online Courses and Tutorials
Free Resources
- Coursera’s Python for Everybody
- Comprehensive beginner-friendly course
- Taught by Dr. Charles Severance
- Includes practice exercises and projects
- freeCodeCamp’s Python Curriculum
- Interactive learning platform
- Project-based learning approach
- Active community support
- Real Python
- In-depth tutorials and articles
- Video courses
- Code examples and explanations
Premium Learning Platforms
Platform | Special Features | Best For | Price Range |
Codecademy Pro | Interactive lessons, projects | Beginners | $15-40/month |
DataCamp | Data science focus | Data Analysis | $25-33/month |
Pluralsight | Skill assessments | Comprehensive learning | $29-45/month |
Essential Python Books
- For Absolute Beginners:
- “Python Crash Course” by Eric Matthes
- “Automate the Boring Stuff with Python” by Al Sweigart
- “Learning Python” by Mark Lutz
- For Intermediate Learners:
- “Python Cookbook” by David Beazley
- “Fluent Python” by Luciano Ramalho
- “Python Tricks: A Buffet of Awesome Python Features” by Dan Bader
Books and courses are great, but the real learning happens when you start building projects and engaging with the community.
Raymond Hettinger, Python Core Developer
Official Documentation and References
The Python Official Documentation remains one of the best resources for learning Python. Key sections include:
- The Python Tutorial: Complete introduction to Python
- The Python Standard Library: Comprehensive library reference
- The Python Language Reference: Detailed language syntax
Python Communities and Forums
Online Communities
- Stack Overflow
- Largest Q&A platform for programming
- Active Python community
- Searchable database of solutions
- Reddit Communities
- r/learnpython
- r/Python
- r/pythonprojects
- Discord Servers
- Python Discord
- Python Developers Community
- Python Learning Community
Local Python Communities
- Python User Groups
- PyLadies chapters
- Local coding bootcamps
Best Practices for Learning
- Structured Learning Approach:
- Follow a curriculum
- Practice daily
- Build projects regularly
- Community Engagement:
- Ask questions
- Share your knowledge
- Participate in discussions
- Project-Based Learning:
- Start with simple projects
- Gradually increase complexity
- Contribute to open source
Interactive Learning Platforms
Platform | Focus Area | Interactive Features | Community Support |
HackerRank | Problem-solving | Code challenges | Yes |
LeetCode | Algorithms | Competition | Yes |
Codewars | Kata exercises | Peer review | Yes |
Tips for Success
- Stay Consistent
- Set a regular learning schedule
- Track your progress
- Join coding challenges
- Build a Portfolio
- Document your learning journey
- Share code on GitHub
- Create practical projects
- Network with Others
- Attend Python conferences
- Join coding meetups
- Participate in hackathons
Remember, the Python community is known for being welcoming and supportive. Don’t hesitate to ask questions and share your knowledge as you learn. The best way to learn is by doing and helping others along the way.
Conclusion: Your Python Programming Journey Starts Now 🚀
As we wrap up this comprehensive Python beginner tutorial, let’s take a moment to reflect on your learning journey and outline your next steps. Remember, every expert programmer started exactly where you are now.
Key Takeaways from Our Python Tutorial
Throughout this tutorial, you’ve learned:
- Fundamental Python Concepts
- Variables and data types
- Control structures
- Functions and modules
- Basic error handling
- Practical Programming Skills
- Writing clean, readable code
- Debugging techniques
- Best practices for Python development
- Project organization
- Real-World Applications
- Building simple applications
- Working with external libraries
- Problem-solving approaches
- Basic project management
Your Next Steps in Python Programming
To continue your Python journey, consider these growth paths:
Learning Path | Description | Estimated Timeline |
Web Development | Learn Django or Flask | 2-3 months |
Data Science | Master Pandas & NumPy | 3-4 months |
Machine Learning | Explore Scikit-learn | 4-6 months |
Automation | Create Python scripts | 1-2 months |
Recommended Resources for Continued Learning
- Online Platforms
- Real Python – Advanced tutorials and articles
- Python.org – Official documentation and resources
- Codecademy Python Track – Interactive lessons
- Community Forums
H3/ Success Stories and Inspiration
Final Tips for Success
- Practice Regularly
- Code for at least 30 minutes daily
- Work on personal projects
- Participate in coding challenges
- Stay Connected
- Join Python communities
- Attend Python meetups
- Follow Python developers on social media
- Build Your Portfolio
- Create a GitHub profile
- Document your projects
- Contribute to open source
The journey of a thousand miles begins with a single step. In programming, that step is your first ‘Hello, World!’ program.
Remember:
- Learning to code is a journey, not a race
- Every expert was once a beginner
- Consistency trumps intensity
- The Python community is here to help
Get Started Today!
Take the first step in your Python journey today. Remember, the best time to start learning was yesterday; the second best time is now. Happy coding! 🐍✨
Frequently Asked Questions About Python Programming
- Basic Syntax: 2-3 weeks
- Core Concepts: 4-6 weeks
- Basic Projects: 2-3 months
- Professional Proficiency: 6-12 months
- Your dedication and practice time
- Previous programming experience
- Learning method (self-paced vs. structured)
- Specific goals (web development, data science, etc.)
- Simpler Syntax: Python uses plain English and fewer special characters
- Less Boilerplate Code: Python requires fewer lines of code to accomplish tasks
- Dynamic Typing: No need to declare variable types explicitly
- Immediate Results: Can run code without compilation
Role | Average Salary (USD) | Required Experience |
---|---|---|
Junior Python Developer | $65,000 – $85,000 | 0-2 years |
Data Analyst | $60,000 – $80,000 | 1-3 years |
QA Automation Engineer | $70,000 – $90,000 | 1-2 years |
Junior Backend Developer | $65,000 – $85,000 | 0-2 years |
- Foundation (Week 1-2)
- Variables and data types
- Basic operators
- Input/output operations
- Control Flow (Week 3-4)
- Conditional statements
- Loops
- Functions
- Data Structures (Week 5-6)
- Lists
- Dictionaries
- Sets and tuples
- Advanced Concepts (Week 7-8)
- Object-oriented programming
- File handling
- Error handling
- Beginners: 1-2 hours daily
- Intermediate: 2-4 hours daily
- Advanced: 4-6 hours daily
- Break learning into 25-minute focused sessions
- Take 5-minute breaks between sessions
- Mix theory with practical coding
- Review and practice consistently rather than cramming
- Core Python Skills Required:
- Solid understanding of Python fundamentals
- Experience with popular frameworks (Django, Flask)
- Knowledge of version control (Git)
- Basic understanding of databases
- Job Opportunities in 2024:
- Web Development
- Data Science
- Machine Learning
- DevOps
- Automation
- Children (8-12): Focus on visual programming tools
- Teenagers (13-17): Begin with basic Python concepts
- Adults (18+): Can start directly with comprehensive tutorials
- Interest in programming
- Dedication to learning
- Access to learning resources
- Consistent practice
- Why Python is Perfect for Beginners:
- Readable, English-like syntax
- Large, helpful community
- Abundant learning resources
- Immediate visual results
- Forgiving learning curve
- Success Rate:
- 80% of beginners successfully complete basic Python courses
- 65% move on to intermediate concepts
- 40% achieve professional proficiency
- Simple syntax
- Extensive documentation
- Active community support
- Abundant learning resources
- Understanding object-oriented concepts (Week 4-6)
- Managing larger projects (Month 2-3)
- Debugging complex code (Month 3-4)
- Salary Ranges by Experience:
- Entry Level: $60,000 – $85,000
- Mid-Level: $85,000 – $120,000
- Senior Level: $120,000 – $200,000+
- Growth Areas:
- AI and Machine Learning
- Data Science
- Web Development
- Cloud Computing
- IoT Applications
2 thoughts on “Python Programming: Python Beginner Tutorial”